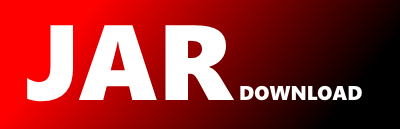
org.yaoqiang.graph.shape.GatewayShape Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yaoqiang-bpmn-editor Show documentation
Show all versions of yaoqiang-bpmn-editor Show documentation
an Open Source BPMN 2.0 Modeler
package org.yaoqiang.graph.shape;
import java.awt.BasicStroke;
import java.awt.Color;
import java.awt.Graphics2D;
import java.awt.Polygon;
import java.awt.Rectangle;
import java.awt.Shape;
import java.util.Map;
import org.yaoqiang.bpmn.model.elements.gateways.EventBasedGateway;
import org.yaoqiang.bpmn.model.elements.gateways.ExclusiveGateway;
import org.yaoqiang.bpmn.model.elements.gateways.Gateway;
import org.yaoqiang.graph.model.GraphModel;
import com.mxgraph.canvas.mxGraphics2DCanvas;
import com.mxgraph.model.mxICell;
import com.mxgraph.shape.mxBasicShape;
import com.mxgraph.util.mxConstants;
import com.mxgraph.util.mxUtils;
import com.mxgraph.view.mxCellState;
/**
* GatewayShape
*
* @author Shi Yaoqiang([email protected])
*/
public class GatewayShape extends mxBasicShape {
public void paintShape(mxGraphics2DCanvas canvas, mxCellState state) {
super.paintShape(canvas, state);
Map style = state.getStyle();
GraphModel model = (GraphModel) state.getView().getGraph().getModel();
Gateway gateway = null;
Object value = ((mxICell)state.getCell()).getValue();
if (value instanceof String) {
gateway = new ExclusiveGateway((String) value);
} else if (value instanceof Gateway) {
gateway = (Gateway) value;
}
Rectangle rect = state.getRectangle();
double scale = canvas.getScale();
Graphics2D g = canvas.getGraphics();
float pw = (float) (mxUtils.getFloat(style, mxConstants.STYLE_STROKEWIDTH, 1) * scale);
if (model.isInclusiveGateway(state.getCell())) {
int inset = (int) Math.round(11 * scale);
int x = rect.x + inset;
int y = rect.y + inset;
int w = rect.width - 2 * inset;
int h = rect.height - 2 * inset;
g.setStroke(new BasicStroke(pw * 3));
g.drawOval(x, y, w, h);
} else if (model.isExclusiveEventGateway(state.getCell())) {
g.setStroke(new BasicStroke(pw));
int inset = (int) Math.round(10 * scale);
int x = rect.x + inset;
int y = rect.y + inset;
int w = rect.width - 2 * inset;
int h = rect.height - 2 * inset;
g.drawOval(x, y, w, h);
if (!((EventBasedGateway) gateway).isInstantiate()) {
inset = (int) Math.round(12 * scale);
x = rect.x + inset;
y = rect.y + inset;
w = rect.width - 2 * inset;
h = rect.height - 2 * inset;
g.drawOval(x, y, w, h);
}
int px = Math.round(rect.x + rect.width / 2);
int py = (int)Math.round(rect.y + rect.width * 0.34);
int p2x = (int)Math.round(rect.x + rect.width * 0.65);
int p2y = (int)Math.round(rect.y + rect.width * 0.45);
int p3x = (int)Math.round(rect.x + rect.width * 0.6);
int p3y = (int)Math.round(rect.y + rect.width * 0.63);
int p4x = (int)Math.round(rect.x + rect.width * 0.4);
int p5x = (int)Math.round(rect.x + rect.width * 0.35);
int[] sx = { px, p2x, p3x, p4x, p5x };
int[] sy = { py, p2y, p3y, p3y, p2y };
g.drawPolygon(sx, sy, 5);
} else if (model.isParallelGateway(state.getCell()) || model.isParallelEventGateway(state.getCell())) {
int px = (int)Math.round(rect.x + rect.width * 0.3);
int py = (int)Math.round(rect.y + rect.width * 0.3);
int p2x = (int)Math.round(rect.x + rect.width * 0.5);
int p2y = (int)Math.round(rect.y + rect.width * 0.5);
int p3x = (int)Math.round(rect.x + rect.width * 0.7);
int p3y = (int)Math.round(rect.y + rect.width * 0.7);
g.setStroke(new BasicStroke(pw * 5));
g.drawLine(px, p2y, p3x, p2y);
g.drawLine(p2x, py, p2x, p3y);
if (model.isParallelEventGateway(state.getCell())) {
g.setStroke(new BasicStroke(pw));
int inset = (int) Math.round(9 * scale);
int x = rect.x + inset;
int y = rect.y + inset;
int w = rect.width - 2 * inset;
int h = rect.height - 2 * inset;
g.drawOval(x, y, w, h);
Color fillColor = mxUtils.getColor(style, mxConstants.STYLE_FILLCOLOR);
g.setColor(fillColor);
g.setStroke(new BasicStroke(pw * 2));
g.drawLine(px, p2y, p3x, p2y);
g.drawLine(p2x, py, p2x, p3y);
}
} else if (model.isComplexGateway(state.getCell())) {
int px = (int)Math.round(rect.x + rect.width * 0.7);
int py = (int)Math.round(rect.y + rect.width * 0.3);
int p2x = (int)Math.round(rect.x + rect.width * 0.3);
int p2y = (int)Math.round(rect.y + rect.width * 0.7);
int p3x = (int)Math.round(rect.x + rect.width * 0.2);
int p3y = (int)Math.round(rect.y + rect.width * 0.8);
int p4x = (int)Math.round(rect.x + rect.width * 0.8);
int p4y = (int)Math.round(rect.y + rect.width * 0.5);
int p5x = (int)Math.round(rect.x + rect.width * 0.5);
int p5y = (int)Math.round(rect.y + rect.width * 0.2);
g.setStroke(new BasicStroke(pw * 4));
g.drawLine(px, py, p2x, p2y);
g.drawLine(p2x, py, px, p2y);
g.drawLine(p3x, p4y, p4x, p4y);
g.drawLine(p5x, p5y, p5x, p3y);
} else if (model.isExclusiveGatewayWithIndicator(state.getCell())) {
int px = (int)Math.round(rect.x + rect.width * 0.65);
int py = (int)Math.round(rect.y + rect.width * 0.25);
int p2x = (int)Math.round(rect.x + rect.width * 0.35);
int p2y = (int)Math.round(rect.y + rect.width * 0.75);
g.setStroke(new BasicStroke(pw * 3));
g.drawLine(px, py, p2x, p2y);
g.drawLine(p2x, py, px, p2y);
}
}
public Shape createShape(mxGraphics2DCanvas canvas, mxCellState state) {
Rectangle bounds = state.getRectangle();
int x = bounds.x;
int y = bounds.y;
int w = bounds.width;
int h = bounds.height;
int halfWidth = w / 2;
int halfHeight = h / 2;
Polygon rhombus = new Polygon();
rhombus.addPoint(x + halfWidth, y);
rhombus.addPoint(x + w, y + halfHeight);
rhombus.addPoint(x + halfWidth, y + h);
rhombus.addPoint(x, y + halfHeight);
return rhombus;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy