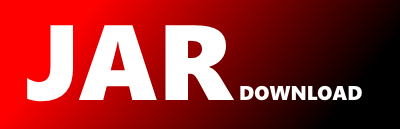
com.mxgraph.view.mxStylesheet Maven / Gradle / Ivy
/**
* $Id: mxStylesheet.java,v 1.27 2010-03-26 10:24:58 gaudenz Exp $
* Copyright (c) 2007, Gaudenz Alder
*/
package com.mxgraph.view;
import java.awt.Color;
import java.util.Hashtable;
import java.util.Map;
import org.yaoqiang.util.Constants;
import com.mxgraph.util.mxConstants;
import com.mxgraph.util.mxUtils;
/**
* Defines the appearance of the cells in a graph. The following example changes the font size for all vertices by
* changing the default vertex style in-place:
* getDefaultVertexStyle().put(mxConstants.STYLE_FONTSIZE, 16);
*
*
* To change the default font size for all cells, set mxConstants.DEFAULT_FONTSIZE.
*/
public class mxStylesheet {
/**
* Shared immutable empty hashtable (for undefined cell styles).
*/
public static final Map EMPTY_STYLE = new Hashtable();
/**
* Maps from names to styles.
*/
protected Map> styles = new Hashtable>();
/**
* Constructs a new stylesheet and assigns default styles.
*/
public mxStylesheet() {
setDefaultVertexStyle(createDefaultVertexStyle());
setDefaultEdgeStyle(createDefaultEdgeStyle());
}
/**
* Returns all styles as map of name, hashtable pairs.
*
* @return All styles in this stylesheet.
*/
public Map> getStyles() {
return styles;
}
/**
* Sets all styles in the stylesheet.
*/
public void setStyles(Map> styles) {
this.styles = styles;
}
/**
* Creates and returns the default vertex style.
*
* @return Returns the default vertex style.
*/
protected Map createDefaultVertexStyle() {
Map style = new Hashtable();
style.put(mxConstants.STYLE_SHAPE, mxConstants.SHAPE_RECTANGLE);
style.put(mxConstants.STYLE_PERIMETER, mxPerimeter.RectanglePerimeter);
style.put(mxConstants.STYLE_VERTICAL_ALIGN, mxConstants.ALIGN_MIDDLE);
style.put(mxConstants.STYLE_ALIGN, mxConstants.ALIGN_CENTER);
style.put(mxConstants.STYLE_FILLCOLOR, "#C3D9FF");
style.put(mxConstants.STYLE_STROKECOLOR, "#6482B9");
style.put(mxConstants.STYLE_FONTCOLOR, "#774400");
return style;
}
/**
* Creates and returns the default edge style.
*
* @return Returns the default edge style.
*/
protected Map createDefaultEdgeStyle() {
Map style = new Hashtable();
style.put(mxConstants.STYLE_SHAPE, mxConstants.SHAPE_CONNECTOR);
style.put(mxConstants.STYLE_ENDARROW, mxConstants.ARROW_CLASSIC);
style.put(mxConstants.STYLE_VERTICAL_ALIGN, mxConstants.ALIGN_MIDDLE);
style.put(mxConstants.STYLE_ALIGN, mxConstants.ALIGN_CENTER);
style.put(mxConstants.STYLE_STROKECOLOR, "#6482B9");
style.put(mxConstants.STYLE_FONTCOLOR, "#446299");
return style;
}
/**
* Returns the default style for vertices.
*
* @return Returns the default vertex style.
*/
public Map getDefaultVertexStyle() {
return styles.get("defaultVertex");
}
/**
* Sets the default style for vertices.
*
* @param value
* Style to be used for vertices.
*/
public void setDefaultVertexStyle(Map value) {
putCellStyle("defaultVertex", value);
}
/**
* Returns the default style for edges.
*
* @return Returns the default edge style.
*/
public Map getDefaultEdgeStyle() {
return styles.get("defaultEdge");
}
/**
* Sets the default style for edges.
*
* @param value
* Style to be used for edges.
*/
public void setDefaultEdgeStyle(Map value) {
putCellStyle("defaultEdge", value);
}
/**
* Stores the specified style under the given name.
*
* @param name
* Name for the style to be stored.
* @param style
* Key, value pairs that define the style.
*/
public void putCellStyle(String name, Map style) {
styles.put(name, style);
}
/**
* Returns the cell style for the specified cell or the given defaultStyle if no style can be found for the given
* stylename.
*
* @param name
* String of the form [(stylename|key=value);] that represents the style.
* @param defaultStyle
* Default style to be returned if no style can be found.
* @return Returns the style for the given formatted cell style.
*/
public Map getCellStyle(String name, Map defaultStyle) {
Map style = defaultStyle;
if (name != null && name.length() > 0) {
String[] pairs = name.split(";");
if (style != null && !name.startsWith(";")) {
style = new Hashtable(style);
} else {
style = new Hashtable();
}
for (int i = 0; i < pairs.length; i++) {
String tmp = pairs[i];
int c = tmp.indexOf('=');
if (c >= 0) {
String key = tmp.substring(0, c);
String value = tmp.substring(c + 1);
if (value.equals(mxConstants.NONE)) {
style.remove(key);
} else {
style.put(key, value);
}
} else {
Map tmpStyle = styles.get(tmp);
if (tmpStyle != null) {
style.putAll(tmpStyle);
}
}
}
}
// ==============start==============
if (mxUtils.isTrue(style, mxConstants.STYLE_SHADOW, false)) {
style.put(mxConstants.STYLE_SHADOW, Constants.SETTINGS.getProperty("style_" + mxConstants.STYLE_SHADOW, "1").equals("1"));
}
String current_lineColor = (String) style.get(mxConstants.STYLE_STROKECOLOR);
String def_lineColor = "#000000";
if (def_lineColor.equals(current_lineColor)) {
String lineColor = Constants.SETTINGS.getProperty("style_" + mxConstants.STYLE_STROKECOLOR, "");
if (lineColor.length() != 0) {
style.put(mxConstants.STYLE_STROKECOLOR, lineColor);
}
}
String shape = (String) style.get(mxConstants.STYLE_SHAPE);
String current_fillColor = (String) style.get(mxConstants.STYLE_FILLCOLOR);
String def_fillColor = "#E8EEF7";
String fillColor = "";
if (Constants.SHAPE_EVENT.equals(shape)) {
String type = (String) style.get(Constants.STYLE_TYPE);
if ("start_event".equals(type)) {
def_fillColor = "#33FF00";
fillColor = Constants.SETTINGS.getProperty("style_startEvent_fillColor", "");
} else if ("end_event".equals(type)) {
def_fillColor = "#FF0000";
fillColor = Constants.SETTINGS.getProperty("style_endEvent_fillColor", "");
} else {
def_fillColor = "#C8FF00";
fillColor = Constants.SETTINGS.getProperty("style_intermediateEvent_fillColor", "");
}
} else if (Constants.SHAPE_GATEWAY.equals(shape)) {
def_fillColor = "#FF9999";
fillColor = Constants.SETTINGS.getProperty("style_gateway_fillColor", "");
} else if (Constants.SHAPE_ACTIVITY.equals(shape)) {
def_fillColor = "#99FF99";
fillColor = Constants.SETTINGS.getProperty("style_task_fillColor", "");
} else if (Constants.SHAPE_SUBPROCESS.equals(shape)) {
def_fillColor = "#CCCCFF";
fillColor = Constants.SETTINGS.getProperty("style_subprocess_fillColor", "");
} else if (mxConstants.SHAPE_SWIMLANE.equals(shape)) {
def_fillColor = "#888888";
fillColor = Constants.SETTINGS.getProperty("style_swimlane_fillColor", "");
} else if (Constants.SHAPE_DATAOBJECT.equals(shape)) {
def_fillColor = "#E8EEF7";
fillColor = Constants.SETTINGS.getProperty("style_dataobject_fillColor", "");
} else if (Constants.SHAPE_DATASTORE.equals(shape)) {
def_fillColor = "#E8EEF7";
fillColor = Constants.SETTINGS.getProperty("style_datastore_fillColor", "");
} else if (Constants.SHAPE_CONVERSATION_NODE.equals(shape)) {
def_fillColor = "#ABC5FF";
fillColor = Constants.SETTINGS.getProperty("style_conversation_fillColor", "");
} else if (mxConstants.SHAPE_LABEL.equals(shape)) {
def_fillColor = "#FFCC00";
String type = (String) style.get(Constants.STYLE_TYPE);
if ("ou".equals(type)) {
fillColor = Constants.SETTINGS.getProperty("style_organizationalUnit_fillColor", "");
} else if ("role".equals(type)) {
fillColor = Constants.SETTINGS.getProperty("style_organizationalRole_fillColor", "");
}
}
if (def_fillColor.equals(current_fillColor)) {
if (fillColor.length() != 0) {
style.put(mxConstants.STYLE_FILLCOLOR, fillColor);
}
}
String current_gradientColor = (String) style.get(mxConstants.STYLE_GRADIENTCOLOR);
current_fillColor = (String) style.get(mxConstants.STYLE_FILLCOLOR);
if (current_fillColor != null) {
Color tmp = mxUtils.parseColor(current_fillColor);
String gradientColor = "#"
+ mxUtils.getHexColorString(new Color(Math.max(tmp.getRed() - 48, 0), Math.max(tmp.getGreen() - 48, 0), Math.max(tmp.getBlue() - 48, 0)))
.substring(2);
if (current_gradientColor == null) {
style.put(mxConstants.STYLE_GRADIENTCOLOR, gradientColor);
}
}
if (Constants.SETTINGS.getProperty("style_" + mxConstants.STYLE_GRADIENTCOLOR, "1").equals("0")) {
style.remove(mxConstants.STYLE_GRADIENTCOLOR);
}
String current_fontColor = (String) style.get(mxConstants.STYLE_FONTCOLOR);
String current_fontSize = (String) style.get(mxConstants.STYLE_FONTSIZE);
String current_fontFamily = (String) style.get(mxConstants.STYLE_FONTFAMILY);
String current_fontStyle = (String) style.get(mxConstants.STYLE_FONTSTYLE);
if (mxConstants.SHAPE_SWIMLANE.equals(shape) ? ("#000000".equals(current_fontColor) && "14".equals(current_fontSize) && "1".equals(current_fontStyle) && current_fontFamily == null)
: ((mxConstants.SHAPE_CONNECTOR.equals(shape) || "11".equals(current_fontSize) && current_fontFamily == null)
&& "#000000".equals(current_fontColor) && current_fontStyle == null)) {
String fontColor = Constants.SETTINGS.getProperty("style_" + mxConstants.STYLE_FONTCOLOR, "");
if (fontColor.length() != 0) {
style.put(mxConstants.STYLE_FONTCOLOR, fontColor);
}
String font = Constants.SETTINGS.getProperty("style_" + mxConstants.STYLE_FONTFAMILY, "");
if (font.length() != 0) {
style.put(mxConstants.STYLE_FONTFAMILY, font);
}
String fontSize = Constants.SETTINGS.getProperty("style_" + mxConstants.STYLE_FONTSIZE, "");
if (fontSize.length() == 0) {
if (mxConstants.SHAPE_CONNECTOR.equals(shape)) {
fontSize = "11";
}
}
if (fontSize.length() != 0) {
style.put(mxConstants.STYLE_FONTSIZE, fontSize);
}
}
// ==============end================
return style;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy