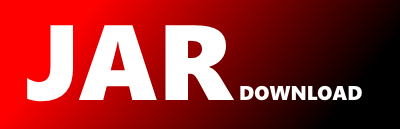
org.yaoqiang.graph.io.vdx.VdxShape Maven / Gradle / Ivy
package org.yaoqiang.graph.io.vdx;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.w3c.dom.Element;
import org.w3c.dom.NodeList;
import com.mxgraph.util.mxPoint;
/**
* VdxShape
*
* @author Shi Yaoqiang([email protected])
*/
public class VdxShape {
protected Element shape;
protected Element xForm;
protected Map mastersMap = new HashMap();
protected Map props = new HashMap();
public VdxShape(Element shape, Map mastersMap) {
this.shape = shape;
this.xForm = getPrimaryTag(VdxConstants.X_FORM);
this.mastersMap = mastersMap;
this.props = getShapeProps();
}
public final Element getShape() {
return shape;
}
public final void setShape(Element shape) {
this.shape = shape;
}
public String getId() {
return shape.getAttribute(VdxConstants.ID);
}
public String getNameU() {
String nameU = "";
if (shape.hasAttribute(VdxConstants.NAME_U)) {
nameU = shape.getAttribute(VdxConstants.NAME_U);
} else if (shape.hasAttribute(VdxConstants.MASTER)) {
nameU = mastersMap.get(shape.getAttribute(VdxConstants.MASTER));
}
return nameU;
}
public String getText() {
String ret = "";
Element text = getPrimaryTag(VdxConstants.TEXT);
if (text != null) {
ret = text.getTextContent();
if (ret.trim().equals("")) {
ret = "";
}
}
String state = props.get(VdxConstants.PROP_NAME_DATASTATE_NAME);
if (state != null) {
ret += "[" + state + "]";
}
return ret;
}
public double getWidth() {
return getNumericalValueOfSecundaryTag(xForm, VdxConstants.WIDTH);
}
public double getHeight() {
return getNumericalValueOfSecundaryTag(xForm, VdxConstants.HEIGHT);
}
public double getPinX() {
return getNumericalValueOfSecundaryTag(xForm, VdxConstants.PIN_X);
}
public double getPinY() {
return getNumericalValueOfSecundaryTag(xForm, VdxConstants.PIN_Y);
}
public double getLocPinX() {
return getNumericalValueOfSecundaryTag(xForm, VdxConstants.LOC_PIN_X);
}
public double getLocPinY() {
return getNumericalValueOfSecundaryTag(xForm, VdxConstants.LOC_PIN_Y);
}
public mxPoint getBeginXY(double parentHeight) {
Element xForm1D = getPrimaryTag(VdxConstants.X_FORM_1D);
double beginX = getNumericalValueOfSecundaryTag(xForm1D, VdxConstants.BEGIN_X);
double beginY = parentHeight - getNumericalValueOfSecundaryTag(xForm1D, VdxConstants.BEGIN_Y);
return new mxPoint(beginX, beginY);
}
public mxPoint getEndXY(double parentHeight) {
Element xForm1D = getPrimaryTag(VdxConstants.X_FORM_1D);
double endX = getNumericalValueOfSecundaryTag(xForm1D, VdxConstants.END_X);
double endY = parentHeight - getNumericalValueOfSecundaryTag(xForm1D, VdxConstants.END_Y);
return new mxPoint(endX, endY);
}
public List getRoutingPoints(double parentHeight) {
mxPoint beginXY = getBeginXY(parentHeight);
ArrayList pointList = new ArrayList();
NodeList lineTos = shape.getElementsByTagName(VdxConstants.LINE_TO);
ArrayList lineToList = new ArrayList();
int numLineTos = lineTos.getLength();
for (int l = 0; l < numLineTos; l++) {
Element lineTo = (Element) lineTos.item(l);
if (!(lineTo.hasAttribute(VdxConstants.DELETED) && (lineTo.getAttribute(VdxConstants.DELETED)).equals("1"))) {
lineToList.add(lineTo);
}
}
int numPoints = lineToList.size();
for (int k = 0; (k < (numPoints - 1)); k++) {
Element lineTo = lineToList.get(k);
mxPoint lineToXY = getLineToXY(lineTo);
Double x = (beginXY.getX() + lineToXY.getX());
Double y = (beginXY.getY() + lineToXY.getY());
pointList.add(new mxPoint(x, y));
}
return pointList;
}
public mxPoint getLineToXY(Element lineTo) {
Element xElem = (Element) lineTo.getElementsByTagName(VdxConstants.X).item(0);
Element yElem = (Element) lineTo.getElementsByTagName(VdxConstants.Y).item(0);
double lineToX = Double.valueOf(xElem.getTextContent()) * VdxCodecUtils.conversionFactor();
double lineToY = (Double.valueOf(yElem.getTextContent()) * VdxCodecUtils.conversionFactor()) * -1;
return new mxPoint(lineToX, lineToY);
}
public mxPoint getOriginPoint(double parentHeight) {
double x = getPinX() - getLocPinX();
double y = parentHeight - (getPinY() + (getHeight() - getLocPinY()));
return new mxPoint(x, y);
}
public mxPoint getDimentions() {
return new mxPoint(getWidth(), getHeight());
}
public boolean isVertexShape() {
String nameU = getNameU();
if (nameU.length() == 0 || props.get(VdxConstants.PROP_NAME_LINK_TYPE) != null || nameU.startsWith("Dynamic connector") || nameU.startsWith("Sequence Flow") || nameU.startsWith("Message Flow")
|| nameU.startsWith("Conversation Link") || nameU.startsWith("Association") || nameU.startsWith("Data Association")) {
return false;
}
return true;
}
public boolean isPoolShape() {
if (getNameU().startsWith(VdxConstants.NAME_U_POOL)) {
return true;
}
return false;
}
public boolean isLaneShape() {
if (getNameU().startsWith(VdxConstants.NAME_U_LANE)) {
return true;
}
return false;
}
public boolean isExpandedSubProcessShape() {
if (getNameU().startsWith(VdxConstants.NAME_U_SUBPROCESS_EXPANDED) || getNameU().startsWith(VdxConstants.NAME_U_EXPANDED_SUBPROCESS)) {
return true;
}
return false;
}
public boolean isAttachedMessageShape() {
if (getNameU().startsWith(VdxConstants.NAME_U_MESSAGE) && getAttachedToShape().length() != 0) {
return true;
}
return false;
}
public boolean isAttachedEventShape() {
if (getNameU().startsWith(VdxConstants.NAME_U_INTERMEDIATE) && getAttachedToShape().length() != 0) {
return true;
}
return false;
}
public String getAttachedToShape() {
String attachedTo = "";
if (isVertexShape()) {
String f = this.getAttributeValueOfSecundaryTag(xForm, VdxConstants.PIN_X, VdxConstants.F);
if (f.startsWith("PNTX(LOCTOPAR(PNT(")) {
attachedTo = f.substring(18, f.indexOf("!Connections"));
if (attachedTo.startsWith("'") && attachedTo.endsWith("'")) {
attachedTo = attachedTo.substring(1, attachedTo.length() - 1);
}
}
}
return attachedTo;
}
public String getStyleFromShape(String stencilType) {
if (stencilType.equals(VdxConstants.STENCIL_TYPE_ITP)) {
return getStyleFromITPShape();
} else {
return getStyleFromTrisotechShape();
}
}
public String getStyleFromITPShape() {
String style = "whiteSpace=wrap;";
String nameU = getNameU();
if (nameU.startsWith(VdxConstants.NAME_U_START)) {
style = "startEvent;";
} else if (nameU.startsWith(VdxConstants.NAME_U_INTERMEDIATE)) {
style = "intermediateEvent;";
} else if (nameU.startsWith(VdxConstants.NAME_U_END)) {
style = "endEvent;";
} else if (nameU.startsWith(VdxConstants.NAME_U_TASK)) {
style += "task;";
} else if (nameU.startsWith(VdxConstants.NAME_U_GATEWAY) || nameU.startsWith(VdxConstants.NAME_U_GATEWAY_DE)) {
style += "gateway;";
} else if (nameU.startsWith(VdxConstants.NAME_U_SUBPROCESS_COLLAPSED_DE) || nameU.startsWith(VdxConstants.NAME_U_SUBPROCESS_COLLAPSED) || nameU.startsWith(VdxConstants.NAME_U_SUBPROCESS_EXPANDED)) {
style += "subprocess;";
} else if (nameU.startsWith(VdxConstants.NAME_U_CALL_ACTIVITY_TASK)) {
style += "callActivity;";
} else if (nameU.startsWith(VdxConstants.NAME_U_CALL_ACTIVITY_SUBPROCESS)) {
style += "callSubprocess;";
} else if (nameU.startsWith(VdxConstants.NAME_U_DATAOBJECT)) {
style = "dataobject;";
}else if (nameU.startsWith(VdxConstants.NAME_U_DATASTORE)) {
style = "datastore;";
} else if (nameU.startsWith(VdxConstants.NAME_U_GROUP)) {
style += "group;";
} else if (nameU.startsWith(VdxConstants.NAME_U_TEXT_ANNOTATION)) {
style += "annotation;";
} else if (nameU.startsWith(VdxConstants.NAME_U_MESSAGE)) {
style += "initiatingMessage;";
} else if (nameU.startsWith(VdxConstants.NAME_U_POOL)) {
String type = props.get(VdxConstants.PROP_NAME_ORIENTATION_ITP);
if ("1".equals(type)) {
style += "swimlane;auto=0;type=vertical;horizontal=1;";
} else {
style += "swimlane;auto=0;";
}
} else if (nameU.startsWith(VdxConstants.NAME_U_LANE)) {
style += "lane;auto=0;";
}
return style;
}
public String getStyleFromTrisotechShape() {
String style = "whiteSpace=wrap;";
String nameU = getNameU();
String loopType = props.get(VdxConstants.PROP_NAME_LOOP_TYPE);
String isForCompensation = props.get(VdxConstants.PROP_NAME_ACTIVITY_COMPENSATION);
if (nameU.startsWith(VdxConstants.NAME_U_TASK)) {
String taskType = props.get(VdxConstants.PROP_NAME_TASK_TYPE);
String taskInstantiate = props.get(VdxConstants.PROP_NAME_TASK_INSTANTIATE);
String isCallActivity = props.get(VdxConstants.PROP_NAME_IS_CALL_ACTIVITY);
String script = props.get(VdxConstants.PROP_NAME_SCRIPT);
String styleScript = "";
String scriptFormat = props.get(VdxConstants.PROP_NAME_SCRIPT_FORMAT);
if (scriptFormat != null && scriptFormat.length() != 0) {
styleScript = "scriptFormat=" + scriptFormat + ";";
}
if (script != null && script.length() != 0) {
styleScript = styleScript + "script=" + script;
}
if ("1".equals(isCallActivity)) {
if ("User".equals(taskType)) {
style += "callUser;";
} else if ("Script".equals(taskType)) {
style += "callScript;" + styleScript;
} else if ("Manual".equals(taskType)) {
style += "callManual;";
} else if ("BusinessRule".equals(taskType)) {
style += "callBusinessRule;";
} else {
style += "callActivity;";
}
} else {
if ("Service".equals(taskType)) {
style += "taskService;";
} else if ("Receive".equals(taskType)) {
if ("1".equals(taskInstantiate)) {
style += "taskReceive;instantiate=1";
} else {
style += "taskReceive;";
}
} else if ("Send".equals(taskType)) {
style += "taskSend;";
} else if ("User".equals(taskType)) {
style += "taskUser;";
} else if ("Script".equals(taskType)) {
style += "taskScript;" + styleScript;
} else if ("Manual".equals(taskType)) {
style += "taskManual;";
} else if ("BusinessRule".equals(taskType)) {
style += "taskBusinessRule;";
} else {
style += "task;";
}
}
if ("Standard".equals(loopType)) {
style += "loop=standard;loopImage=/org/yaoqiang/graph/images/marker_loop.png;";
} else if ("MultiInstanceParallel".equals(loopType)) {
style += "loop=multi_instance;loopImage=/org/yaoqiang/graph/images/marker_multiple.png;";
} else if ("MultiInstanceSequential".equals(loopType)) {
style += "loop=multi_instance_sequential;loopImage=/org/yaoqiang/graph/images/marker_multiple_sequential.png;";
}
} else if (nameU.startsWith(VdxConstants.NAME_U_SUBPROCESS) || nameU.startsWith(VdxConstants.NAME_U_EXPANDED_SUBPROCESS)) {
String type = props.get(VdxConstants.PROP_NAME_SUBPROCESS_TYPE);
if ("Embedded".equals(type)) {
String isTransaction = props.get(VdxConstants.PROP_NAME_IS_TRANSACTION);
String isAdhoc = props.get(VdxConstants.PROP_NAME_IS_ADHOC);
if ("1".equals(isTransaction)) {
style += "tranSubprocess;";
} else if ("1".equals(isAdhoc)) {
style += "adHocSubprocess;";
} else {
style += "subprocess;";
}
} else if ("Event".equals(type)) {
style += "eventSubprocess;";
} else if ("CallActivity".equals(type)) {
style += "callSubprocess;";
} else {
style += "subprocess;";
}
if ("Standard".equals(loopType)) {
style += "loop=standard;loopImage=/org/yaoqiang/graph/images/marker_loop.png;";
} else if ("MultiInstanceParallel".equals(loopType)) {
style += "loop=multi_instance;loopImage=/org/yaoqiang/graph/images/marker_multiple.png;";
} else if ("MultiInstanceSequential".equals(loopType)) {
style += "loop=multi_instance_sequential;loopImage=/org/yaoqiang/graph/images/marker_multiple_sequential.png;";
}
} else if (nameU.startsWith(VdxConstants.NAME_U_START_EVENT)) {
String trigger = props.get(VdxConstants.PROP_NAME_START_EVENT_TRIGGER);
String interrupting = props.get(VdxConstants.PROP_NAME_START_EVENT_INTERRUPTING);
if ("1".equals(interrupting)) {
if ("Message".equals(trigger)) {
style = "startMessageEvent;";
} else if ("Timer".equals(trigger)) {
style = "startTimerEvent;";
} else if ("Conditional".equals(trigger)) {
style = "startConditionalEvent;";
} else if ("Signal".equals(trigger)) {
style = "startSignalEvent;";
} else if ("Error".equals(trigger)) {
style = "startErrorEvent;";
} else if ("Escalation".equals(trigger)) {
style = "startEscalationEvent;";
} else if ("Compensation".equals(trigger)) {
style = "startCompensationEvent;";
} else if ("Multiple".equals(trigger)) {
style = "startMultipleEvent;";
} else if ("ParallelMultiple".equals(trigger)) {
style = "startParallelMultipleEvent;";
} else {
style = "startEvent;";
}
} else {
if ("Message".equals(trigger)) {
style = "startMessageNonInterruptingEvent;";
} else if ("Timer".equals(trigger)) {
style = "startTimerNonInterruptingEvent;";
} else if ("Conditional".equals(trigger)) {
style = "startConditionalNonInterruptingEvent;";
} else if ("Signal".equals(trigger)) {
style = "startSignalNonInterruptingEvent;";
} else if ("Escalation".equals(trigger)) {
style = "startEscalationNonInterruptingEvent;";
} else if ("Multiple".equals(trigger)) {
style = "startMultipleNonInterruptingEvent;";
} else if ("ParallelMultiple".equals(trigger)) {
style = "startParallelMultipleNonInterruptingEvent;";
} else {
style = "startEvent;";
}
}
} else if (nameU.startsWith(VdxConstants.NAME_U_END_EVENT)) {
String results = props.get(VdxConstants.PROP_NAME_END_EVENT_RESULTS);
if ("Message".equals(results)) {
style = "endMessageEvent;";
} else if ("Error".equals(results)) {
style = "endErrorEvent;";
} else if ("Cancel".equals(results)) {
style = "endCancelEvent;";
} else if ("Compensation".equals(results)) {
style = "endCompensationEvent;";
} else if ("Signal".equals(results)) {
style = "endSignalEvent;";
} else if ("Multiple".equals(results)) {
style = "endMultipleEvent;";
} else if ("Terminate".equals(results)) {
style = "endTerminateEvent;";
} else if ("Escalation".equals(results)) {
style = "endEscalationEvent;";
} else {
style = "endEvent;";
}
} else if (nameU.startsWith(VdxConstants.NAME_U_INTERMEDIATE_EVENT)) {
String direction = props.get(VdxConstants.PROP_NAME_EVENT_DIRECTION);
String trigger = props.get(VdxConstants.PROP_NAME_INTERMEDIATE_EVENT_TRIGGER);
if ("Throwing".equals(direction)) {
if ("Message".equals(trigger)) {
style = "endMessageEvent;";
} else if ("Escalation".equals(trigger)) {
style = "intermediateEscalationThrowEvent;";
} else if ("Link".equals(trigger)) {
style = "intermediateLinkThrowEvent;";
} else if ("Compensation".equals(trigger)) {
style = "intermediateCompensationThrowEvent;";
} else if ("Signal".equals(trigger)) {
style = "intermediateSignalThrowEvent;";
} else if ("Multiple".equals(trigger)) {
style = "intermediateMultipleThrowEvent;";
} else {
style = "intermediateEvent;";
}
} else {
String interrupting = props.get(VdxConstants.PROP_NAME_INTERMEDIATE_EVENT_INTERRUPTING);
if ("1".equals(interrupting)) {
if ("Message".equals(trigger)) {
style = "intermediateMessageEvent;";
} else if ("Timer".equals(trigger)) {
style = "intermediateTimerEvent;";
} else if ("Escalation".equals(trigger)) {
style = "intermediateEscalationEvent;";
} else if ("Conditional".equals(trigger)) {
style = "intermediateConditionalEvent;";
} else if ("Link".equals(trigger)) {
style = "intermediateLinkEvent;";
} else if ("Error".equals(trigger)) {
style = "intermediateErrorEvent;";
} else if ("Cancel".equals(trigger)) {
style = "intermediateCancelEvent;";
} else if ("Compensation".equals(trigger)) {
style = "intermediateCompensationEvent;";
} else if ("Signal".equals(trigger)) {
style = "intermediateSignalEvent;";
} else if ("Multiple".equals(trigger)) {
style = "intermediateMultipleEvent;";
} else if ("ParallelMultiple".equals(trigger)) {
style = "intermediateParallelMultipleEvent;";
} else {
style = "intermediateEvent;";
}
} else {
if ("Message".equals(trigger)) {
style = "intermediateMessageNonInterruptingEvent;";
} else if ("Timer".equals(trigger)) {
style = "intermediateTimerNonInterruptingEvent;";
} else if ("Escalation".equals(trigger)) {
style = "intermediateEscalationNonInterruptingEvent;";
} else if ("Conditional".equals(trigger)) {
style = "intermediateConditionalNonInterruptingEvent;";
} else if ("Signal".equals(trigger)) {
style = "intermediateSignalNonInterruptingEvent;";
} else if ("Multiple".equals(trigger)) {
style = "intermediateMultipleNonInterruptingEvent;";
} else if ("ParallelMultiple".equals(trigger)) {
style = "intermediateParallelMultipleNonInterruptingEvent;";
} else {
style = "intermediateEvent;";
}
}
}
} else if (nameU.startsWith(VdxConstants.NAME_U_GATEWAY)) {
String type = props.get(VdxConstants.PROP_NAME_GATEWAY_TYPE);
if ("Exclusive".equals(type)) {
String marker = props.get(VdxConstants.PROP_NAME_GATEWAY_MARKER);
if ("1".equals(marker)) {
style += "exclusiveGatewayWithIndicator;";
} else {
style += "exclusiveGateway;";
}
} else if ("Inclusive".equals(type)) {
style += "inclusiveGateway;";
} else if ("Complex".equals(type)) {
style += "complexGateway;";
} else if ("Parallel".equals(type)) {
style += "parallelGateway;";
} else if ("EventExclusive".equals(type)) {
style += "eventGateway;";
} else if ("EventExclusiveStart".equals(type)) {
style += "eventGatewayInstantiate;";
} else if ("EventParallelStart".equals(type)) {
style += "parallelEventGateway;";
} else {
style += "gateway;";
}
} else if (nameU.startsWith(VdxConstants.NAME_U_DATAOBJECT)) {
String type = props.get(VdxConstants.PROP_NAME_DATAOBJECT_TYPE);
String isCollection = props.get(VdxConstants.PROP_NAME_IS_COLLECTION);
if ("1".equals(isCollection)) {
if ("Input".equals(type)) {
style = "datainputs;";
} else if ("Output".equals(type)) {
style = "dataoutputs;";
} else {
style = "dataobjects;";
}
} else {
if ("Input".equals(type)) {
style = "datainput;";
} else if ("Output".equals(type)) {
style = "dataoutput;";
} else {
style = "dataobject;";
}
}
} else if (nameU.startsWith(VdxConstants.NAME_U_DATASTORE)) {
style = "datastore;";
} else if (nameU.startsWith(VdxConstants.NAME_U_GROUP)) {
style += "group;";
} else if (nameU.startsWith(VdxConstants.NAME_U_ANNOTATION)) {
style += "annotation;";
} else if (nameU.startsWith(VdxConstants.NAME_U_POOL)) {
String type = props.get(VdxConstants.PROP_NAME_ORIENTATION);
if ("VERTICAL".equals(type)) {
style += "swimlane;auto=0;type=vertical;horizontal=1;";
} else {
style += "swimlane;auto=0;";
}
String mi = props.get(VdxConstants.PROP_NAME_POOL_MULTIINSTANCE);
if ("1".equals(mi)) {
style += "multi_instance=1;";
}
} else if (nameU.startsWith(VdxConstants.NAME_U_LANE)) {
String type = props.get(VdxConstants.PROP_NAME_ORIENTATION);
if ("VERTICAL".equals(type)) {
style += "lane;auto=0;type=vertical;horizontal=1;";
} else {
style += "lane;auto=0;";
}
} else if (nameU.startsWith(VdxConstants.NAME_U_CONVERSATION) || nameU.startsWith(VdxConstants.NAME_U_SUBCONVERSATION)) {
String conversationType = props.get(VdxConstants.PROP_NAME_CONVERSATION_TYPE);
if ("Conversation".equals(conversationType)) {
style = "conversation";
} else if ("SubConversation".equals(conversationType)) {
style = "subConversation";
} else if ("CallConversation".equals(conversationType)) {
style = "callConversation";
} else if ("CallSubConversation".equals(conversationType)) {
style = "callCollaboration";
} else {
if (nameU.startsWith(VdxConstants.NAME_U_CONVERSATION)) {
style = "conversation";
} else {
style = "subConversation";
}
}
}
if ("1".equals(isForCompensation)) {
style += "compensation=1;compensationImage=/org/yaoqiang/graph/images/marker_compensation.png;";
}
return style;
}
public String getStyleFromEdgeShape() {
String style = null;
String linkType = props.get(VdxConstants.PROP_NAME_LINK_TYPE);
String conditionType = props.get(VdxConstants.PROP_NAME_CONDITION_TYPE);
String conditionExpression = props.get(VdxConstants.PROP_NAME_CONDITION_EXPRESSION);
String isConnectedToGateway = props.get(VdxConstants.PROP_NAME_IS_CONN_TO_GATEWAY_TYPE);
if ("Message Flow".equals(linkType)) {
style = "messageFlow;";
} else if ("Conversation Link".equals(linkType)) {
style = "conversationLink;";
} else if ("Association".equals(linkType)) {
style = "association;";
} else if ("Data Association".equals(linkType)) {
style = "dataAssociation;";
} else if ("Sequence Flow".equals(linkType)) {
String expr = "";
if (conditionExpression != null && conditionExpression.length() != 0) {
expr = "expression=" + conditionExpression;
}
if ("Expression".equals(conditionType)) {
if ("1".equals(isConnectedToGateway)) {
style = "defaultEdge;" + expr;
} else {
style = "conditionFlow;" + expr;
}
} else if ("Default".equals(conditionType)) {
style = "defaultFlow;";
} else {
style = "defaultEdge;";
}
} else {
String nameU = getNameU();
if (nameU.startsWith("Sequence Flow")) {
String expr = "";
if (conditionExpression != null && conditionExpression.length() != 0) {
expr = "expression=" + conditionExpression;
}
if ("Expression".equals(conditionType)) {
if ("1".equals(isConnectedToGateway)) {
style = "defaultEdge;" + expr;
} else {
style = "conditionFlow;" + expr;
}
} else if ("Default".equals(conditionType)) {
style = "defaultFlow;";
} else {
style = "defaultEdge;";
}
} else if (nameU.startsWith("Message Flow")) {
style = "messageFlow;";
} else if (nameU.startsWith("Conversation Link")) {
style = "conversationLink;";
} else if (nameU.startsWith("Association")) {
style = "association;";
} else if (nameU.startsWith("Data Association")) {
style = "dataAssociation;";
} else {
style = "defaultEdge;";
}
}
return style;
}
protected Map getShapeProps() {
NodeList childrens = shape.getChildNodes();
return VdxCodecUtils.getShapePropMap(childrens);
}
protected Element getPrimaryTag(String tag) {
NodeList childrens = shape.getChildNodes();
Element primary = null;
if (VdxCodecUtils.nodeListHasTag(childrens, tag)) {
primary = VdxCodecUtils.nodeListTag(childrens, tag);
}
return primary;
}
protected double getNumericalValueOfSecundaryTag(Element primary, String tag) {
double val = 0;
NodeList xChildrens = null;
if (primary != null) {
xChildrens = primary.getChildNodes();
}
Element elem = null;
if (VdxCodecUtils.nodeListHasTag(xChildrens, tag)) {
elem = VdxCodecUtils.nodeListTag(xChildrens, tag);
}
if (elem != null) {
val = Double.parseDouble(elem.getTextContent()) * VdxCodecUtils.conversionFactor();
}
return val;
}
protected String getAttributeValueOfSecundaryTag(Element primary, String tag, String attr) {
String val = "";
NodeList xChildrens = null;
if (primary != null) {
xChildrens = primary.getChildNodes();
}
Element elem = null;
if (VdxCodecUtils.nodeListHasTag(xChildrens, tag)) {
elem = VdxCodecUtils.nodeListTag(xChildrens, tag);
}
if (elem != null) {
val = elem.getAttribute(attr);
}
return val;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy