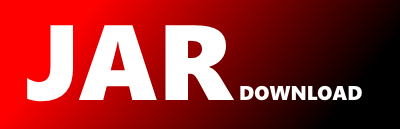
org.yaoqiang.graph.action.GraphActions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yaoqiang-bpmn-editor Show documentation
Show all versions of yaoqiang-bpmn-editor Show documentation
an Open Source BPMN 2.0 Modeler
package org.yaoqiang.graph.action;
import java.awt.Color;
import java.awt.Component;
import java.awt.event.ActionEvent;
import java.awt.print.PageFormat;
import java.awt.print.PrinterException;
import java.awt.print.PrinterJob;
import java.util.Arrays;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import javax.swing.AbstractAction;
import javax.swing.JColorChooser;
import javax.swing.JOptionPane;
import javax.swing.TransferHandler;
import org.yaoqiang.bpmn.model.elements.choreographyactivities.ChoreographyActivity;
import org.yaoqiang.bpmn.model.elements.collaboration.Participant;
import org.yaoqiang.graph.layout.BPMNLayout;
import org.yaoqiang.graph.model.GraphModel;
import org.yaoqiang.graph.swing.GraphComponent;
import org.yaoqiang.graph.util.GraphUtils;
import org.yaoqiang.graph.view.Graph;
import org.yaoqiang.util.Constants;
import org.yaoqiang.util.Resources;
import com.mxgraph.layout.mxIGraphLayout;
import com.mxgraph.model.mxCell;
import com.mxgraph.model.mxGeometry;
import com.mxgraph.model.mxGraphModel;
import com.mxgraph.model.mxICell;
import com.mxgraph.swing.util.mxMorphing;
import com.mxgraph.util.mxConstants;
import com.mxgraph.util.mxEvent;
import com.mxgraph.util.mxEventObject;
import com.mxgraph.util.mxEventSource.mxIEventListener;
import com.mxgraph.util.mxUtils;
/**
* GraphActions
*
* @author Shi Yaoqiang([email protected])
*/
public class GraphActions extends AbstractAction {
private static final long serialVersionUID = 1L;
public static final int ZOOM = 001;
public static final int ZOOM_IN = 002;
public static final int ZOOM_OUT = 003;
public static final int ZOOM_ACTUAL = 004;
public static final int ZOOM_FIT_PAGE = 005;
public static final int ZOOM_FIT_WIDTH = 006;
public static final int ZOOM_CUSTOM = 007;
public static final int EDIT = 101;
public static final int PASTE = 102;
public static final int DUPLICATE = 103;
public static final int DELETE = 104;
public static final int PAGE_SETUP = 105;
public static final int PRINT = 106;
public static final int SELECT_ALL = 201;
public static final int SELECT_NONE = 202;
public static final int SELECT_VERTICES = 203;
public static final int SELECT_EDGES = 204;
public static final int SELECT_PREVIOUS = 205;
public static final int SELECT_NEXT = 206;
public static final int SELECT_PARENT = 207;
public static final int SELECT_CHILD = 208;
public static final int SELECT_PRROCESS = 209;
public static final int ALIGN_LEFT = 301;
public static final int ALIGN_CENTER = 302;
public static final int ALIGN_RIGHT = 303;
public static final int ALIGN_TOP = 304;
public static final int ALIGN_MIDDLE = 305;
public static final int ALIGN_BOTTOM = 306;
public static final int DISTRIBUTE_HORIZONTALLY = 307;
public static final int DISTRIBUTE_VERTICALLY = 308;
public static final int SAME = 309;
public static final int SAME_HEIGHT = 310;
public static final int SAME_WIDTH = 311;
public static final int MOVE_UP = 312;
public static final int MOVE_DOWN = 313;
public static final int MOVE_RIGHT = 314;
public static final int MOVE_LEFT = 315;
public static final int BACKGROUND = 408;
public static final int ADD_PAGE = 409;
public static final int REMOVE_PAGE = 410;
public static final int DEBUG_STYLE = 500;
public static final int FOLD_CELLS = 502;
public static final int ROTATE_SWIMLANE = 503;
public static final int MOVE_LANE = 504;
public static final int LABEL_POSITION = 506;
public static final int PROMPT_VALUE = 508;
public static final int TOGGLE = 509;
public static final int STYLE = 510;
public static final int INIT_PART = 511;
public static final int MULTI_PART = 512;
public static final int ADD_PART = 513;
public static final int DEL_PART = 514;
public static final int SWAP_PART = 515;
public static final int RESET_BACKGROUND = 517;
public static final int AUTO_LAYOUT = 518;
public static final int HOME = 701;
public static final int ENTER_GROUP = 702;
public static final int EXIT_GROUP = 703;
public static final int GROUP = 704;
public static final int UNGROUP = 705;
private int type;
private boolean booleanValue;
private double doubleValue;
private String stringValue;
private String stringValue2;
private Object objectValue;
public GraphActions(int type) {
this.type = type;
}
public static GraphActions getAction(int type) {
return new GraphActions(type);
}
public static GraphActions getScaleAction(double value) {
return new GraphActions(ZOOM).setDoubleValue(value);
}
public static GraphActions getFastMoveAction(int type) {
return new GraphActions(type).setBooleanValue(true);
}
public static GraphActions getMoveLaneAction(double value) {
return new GraphActions(MOVE_LANE).setDoubleValue(value);
}
public static GraphActions getAddPageAction(boolean horizontal) {
return new GraphActions(ADD_PAGE).setBooleanValue(horizontal);
}
public static GraphActions getRemovePageAction(boolean horizontal) {
return new GraphActions(REMOVE_PAGE).setBooleanValue(horizontal);
}
public static GraphActions getLabelPosAction(String labelPosition, String alignment) {
return new GraphActions(LABEL_POSITION).setStringValue(labelPosition).setStringValue2(alignment);
}
public static GraphActions getPromptValueAction(String key, String message) {
return new GraphActions(PROMPT_VALUE).setStringValue(key).setStringValue2(message);
}
public static GraphActions getToggleAction(String key, boolean defaultValue) {
return new GraphActions(TOGGLE).setStringValue(key).setBooleanValue(defaultValue);
}
public static GraphActions getStyleAction(String value) {
return new GraphActions(STYLE).setStringValue(value);
}
public static GraphActions getStyleAction(Object cell, String value) {
return new GraphActions(STYLE).setObjectValue(cell).setStringValue(value);
}
public static GraphActions getInitPartAction(Object part) {
return new GraphActions(INIT_PART).setObjectValue(part);
}
public static GraphActions getMultiPartAction(Object part, String max) {
return new GraphActions(MULTI_PART).setObjectValue(part).setStringValue(max);
}
public static GraphActions getAddPartAction(Object parent, boolean toTop) {
return new GraphActions(ADD_PART).setObjectValue(parent).setBooleanValue(toTop);
}
public static GraphActions getDelPartAction(Object part) {
return new GraphActions(DEL_PART).setObjectValue(part);
}
public static GraphActions getSwapPartAction() {
return new GraphActions(SWAP_PART);
}
public static GraphActions getResetBackgroundAction() {
return new GraphActions(RESET_BACKGROUND);
}
public static GraphActions getAutoLayoutAction() {
return new GraphActions(AUTO_LAYOUT);
}
public static GraphActions getAutoLayoutAction(String value) {
return new GraphActions(AUTO_LAYOUT).setStringValue(value);
}
public static GraphActions getSelectProcessAction(String value) {
return new GraphActions(SELECT_PRROCESS).setStringValue(value);
}
public void actionPerformed(ActionEvent e) {
GraphComponent graphComponent = (GraphComponent) e.getSource();
final Graph graph = graphComponent.getGraph();
GraphModel model = graph.getModel();
Object cell = graph.getSelectionCell();
if (type == ZOOM) {
if (doubleValue > 0) {
graphComponent.zoomTo(doubleValue, graphComponent.isCenterZoom());
}
} else if (type == ZOOM_IN) {
graphComponent.zoomIn();
} else if (type == ZOOM_OUT) {
graphComponent.zoomOut();
} else if (type == ZOOM_ACTUAL) {
graphComponent.zoomActual();
} else if (type == ZOOM_FIT_PAGE) {
graphComponent.setZoomPolicy(GraphComponent.ZOOM_POLICY_PAGE);
} else if (type == ZOOM_FIT_WIDTH) {
graphComponent.setZoomPolicy(GraphComponent.ZOOM_POLICY_WIDTH);
} else if (type == ZOOM_CUSTOM) {
double scale = 0;
String value = (String) JOptionPane.showInputDialog(graphComponent, Resources.get("value"), Resources.get("scale") + " (%)",
JOptionPane.PLAIN_MESSAGE, null, null, "100");
if (value != null) {
scale = Double.parseDouble(value.replace("%", "")) / 100;
}
if (scale > 0) {
graphComponent.zoomTo(scale, graphComponent.isCenterZoom());
}
} else if (type == EDIT) {
graphComponent.startEditing();
} else if (type == PASTE) {
graphComponent.setPasteToPoint(graphComponent.getPopupPoint());
TransferHandler.getPasteAction().actionPerformed(e);
graphComponent.setPasteToPoint(null);
} else if (type == DUPLICATE) {
TransferHandler.getCopyAction().actionPerformed(e);
TransferHandler.getPasteAction().actionPerformed(e);
} else if (type == DELETE) {
graph.removeCells();
} else if (type == PAGE_SETUP) {
PrinterJob pj = PrinterJob.getPrinterJob();
PageFormat format = pj.pageDialog(graphComponent.getPageFormat());
if (format != null) {
Constants.SWIMLANE_WIDTH = (int) (format.getWidth() * 1.25 + (model.getHorizontalPageCount() - 1)
* (Constants.SWIMLANE_START_POINT + format.getWidth() * 1.25));
Constants.SWIMLANE_HEIGHT = (int) (format.getHeight() * 1.2 + (model.getPageCount() - 1)
* (Constants.SWIMLANE_START_POINT + format.getHeight() * 1.2));
graphComponent.setPageFormat(format);
model.setPageFormat(format);
GraphUtils.arrangeAllSwimlaneLength(graph, true);
graphComponent.zoomAndCenter();
}
} else if (type == PRINT) {
PrinterJob pj = PrinterJob.getPrinterJob();
if (pj.printDialog()) {
PageFormat pf = graphComponent.getPageFormat();
pj.setPrintable(graphComponent, pf);
try {
pj.print();
} catch (PrinterException e2) {
System.out.println(e2);
}
}
} else if (type == SELECT_ALL) {
graph.selectAll();
} else if (type == SELECT_NONE) {
graph.clearSelection();
} else if (type == SELECT_VERTICES) {
graph.selectVertices();
} else if (type == SELECT_EDGES) {
graph.selectEdges();
} else if (type == SELECT_PREVIOUS) {
graph.selectPreviousCell();
} else if (type == SELECT_NEXT) {
graph.selectNextCell();
} else if (type == SELECT_PARENT) {
graph.selectParentCell();
} else if (type == SELECT_CHILD) {
graph.selectChildCell();
} else if (type == SELECT_PRROCESS) {
graph.selectProcess(stringValue);
} else if (type == MOVE_UP) {
graph.moveCells("up", booleanValue);
} else if (type == MOVE_DOWN) {
graph.moveCells("down", booleanValue);
} else if (type == MOVE_RIGHT) {
graph.moveCells("right", booleanValue);
} else if (type == MOVE_LEFT) {
graph.moveCells("left", booleanValue);
} else if (type == ALIGN_LEFT) {
graph.alignCells(mxConstants.ALIGN_LEFT);
} else if (type == ALIGN_CENTER) {
graph.alignCells(mxConstants.ALIGN_CENTER);
} else if (type == ALIGN_RIGHT) {
graph.alignCells(mxConstants.ALIGN_RIGHT);
} else if (type == ALIGN_TOP) {
graph.alignCells(mxConstants.ALIGN_TOP);
} else if (type == ALIGN_MIDDLE) {
graph.alignCells(mxConstants.ALIGN_MIDDLE);
} else if (type == ALIGN_BOTTOM) {
graph.alignCells(mxConstants.ALIGN_BOTTOM);
} else if (type == DISTRIBUTE_HORIZONTALLY) {
graph.distributeCells(mxConstants.ALIGN_CENTER);
} else if (type == DISTRIBUTE_VERTICALLY) {
graph.distributeCells(mxConstants.ALIGN_MIDDLE);
} else if (type == SAME) {
graph.sameCells("");
} else if (type == SAME_HEIGHT) {
graph.sameCells(Constants.HEIGHT);
} else if (type == SAME_WIDTH) {
graph.sameCells(Constants.WIDTH);
} else if (type == BACKGROUND) {
Color newColor = JColorChooser.showDialog(graphComponent, Resources.get("background"), null);
if (newColor != null) {
graphComponent.getViewport().setOpaque(true);
graphComponent.getViewport().setBackground(newColor);
graphComponent.getGraph().getModel().setBackgroundColor(newColor);
}
graphComponent.repaint();
} else if (type == ADD_PAGE || type == REMOVE_PAGE) {
int verticalCount = graphComponent.getVerticalPageCount();
int horizontalCount = graphComponent.getHorizontalPageCount();
if (type == ADD_PAGE) {
if (booleanValue) {
graphComponent.setHorizontalPageCount(horizontalCount + 1);
} else {
graphComponent.setVerticalPageCount(verticalCount + 1);
}
} else {
if (booleanValue) {
if (horizontalCount > 1)
graphComponent.setHorizontalPageCount(horizontalCount - 1);
} else {
if (verticalCount > 1)
graphComponent.setVerticalPageCount(verticalCount - 1);
}
}
if (booleanValue) {
model.setHorizontalPageCount(graphComponent.getHorizontalPageCount());
} else {
model.setPageCount(graphComponent.getVerticalPageCount());
}
PageFormat format = model.getPageFormat();
Constants.SWIMLANE_WIDTH = (int) (format.getWidth() * 1.25 + (model.getHorizontalPageCount() - 1)
* (Constants.SWIMLANE_START_POINT + format.getWidth() * 1.25));
Constants.SWIMLANE_HEIGHT = (int) (format.getHeight() * 1.2 + (model.getPageCount() - 1)
* (Constants.SWIMLANE_START_POINT + format.getHeight() * 1.2));
GraphUtils.arrangeAllSwimlaneLength(graphComponent.getGraph(), true);
graphComponent.zoomAndCenter();
} else if (type == DEBUG_STYLE) {
String initial = graph.getModel().getStyle(cell);
String value = (String) JOptionPane.showInputDialog(graphComponent, Resources.get("style"), Resources.get("style"), JOptionPane.PLAIN_MESSAGE,
null, null, initial);
if (value != null) {
graph.setCellStyle(value);
}
} else if (type == FOLD_CELLS) {
if (graph != null && graph.getSelectionCount() == 1) {
if (graph.isSubChoreography(cell)) {
cell = GraphUtils.getChoreographyActivity(graph, cell);
graph.foldCells(!graph.isCellCollapsed(cell), false, new Object[] { cell });
} else {
graph.foldCells(!graph.isCellCollapsed(cell));
}
}
} else if (type == ROTATE_SWIMLANE) {
GraphUtils.rotateSwimlane(graphComponent);
} else if (type == HOME) {
graph.home();
graph.fireEvent(new mxEventObject(Constants.EXIT_GROUP, "cell", cell));
} else if (type == ENTER_GROUP) {
graph.enterGroup();
graph.fireEvent(new mxEventObject(Constants.ENTER_GROUP, "cell", cell));
} else if (type == EXIT_GROUP) {
graph.exitGroup();
graph.fireEvent(new mxEventObject(Constants.EXIT_GROUP, "cell", cell));
} else if (type == GROUP) {
model.beginUpdate();
graph.setSelectionCell(graph.groupCells(null, 20));
model.endUpdate();
} else if (type == UNGROUP) {
model.beginUpdate();
graph.ungroupCells();
model.endUpdate();
} else if (type == LABEL_POSITION) {
model.beginUpdate();
try {
if (stringValue.equals(mxConstants.ALIGN_LEFT)) {
graph.setCellStyles(mxConstants.STYLE_LABEL_POSITION, mxConstants.ALIGN_LEFT);
graph.setCellStyles(mxConstants.STYLE_VERTICAL_LABEL_POSITION, mxConstants.ALIGN_MIDDLE);
graph.setCellStyles(mxConstants.STYLE_ALIGN, mxConstants.ALIGN_RIGHT);
graph.setCellStyles(mxConstants.STYLE_VERTICAL_ALIGN, mxConstants.ALIGN_MIDDLE);
} else if (stringValue.equals(mxConstants.ALIGN_RIGHT)) {
graph.setCellStyles(mxConstants.STYLE_LABEL_POSITION, mxConstants.ALIGN_RIGHT);
graph.setCellStyles(mxConstants.STYLE_VERTICAL_LABEL_POSITION, mxConstants.ALIGN_MIDDLE);
graph.setCellStyles(mxConstants.STYLE_ALIGN, mxConstants.ALIGN_LEFT);
graph.setCellStyles(mxConstants.STYLE_VERTICAL_ALIGN, mxConstants.ALIGN_MIDDLE);
} else if (stringValue.equals(mxConstants.ALIGN_TOP)) {
if (stringValue2.equals(mxConstants.ALIGN_LEFT)) {
graph.setCellStyles(mxConstants.STYLE_LABEL_POSITION, mxConstants.ALIGN_LEFT);
graph.setCellStyles(mxConstants.STYLE_VERTICAL_LABEL_POSITION, mxConstants.ALIGN_TOP);
graph.setCellStyles(mxConstants.STYLE_ALIGN, mxConstants.ALIGN_RIGHT);
graph.setCellStyles(mxConstants.STYLE_VERTICAL_ALIGN, mxConstants.ALIGN_BOTTOM);
} else if (stringValue2.equals(mxConstants.ALIGN_RIGHT)) {
graph.setCellStyles(mxConstants.STYLE_LABEL_POSITION, mxConstants.ALIGN_RIGHT);
graph.setCellStyles(mxConstants.STYLE_VERTICAL_LABEL_POSITION, mxConstants.ALIGN_TOP);
graph.setCellStyles(mxConstants.STYLE_ALIGN, mxConstants.ALIGN_LEFT);
graph.setCellStyles(mxConstants.STYLE_VERTICAL_ALIGN, mxConstants.ALIGN_BOTTOM);
} else {
graph.setCellStyles(mxConstants.STYLE_LABEL_POSITION, mxConstants.ALIGN_CENTER);
graph.setCellStyles(mxConstants.STYLE_VERTICAL_LABEL_POSITION, mxConstants.ALIGN_TOP);
graph.setCellStyles(mxConstants.STYLE_ALIGN, mxConstants.ALIGN_CENTER);
graph.setCellStyles(mxConstants.STYLE_VERTICAL_ALIGN, mxConstants.ALIGN_BOTTOM);
}
} else if (stringValue.equals(mxConstants.ALIGN_BOTTOM)) {
if (stringValue2.equals(mxConstants.ALIGN_LEFT)) {
graph.setCellStyles(mxConstants.STYLE_LABEL_POSITION, mxConstants.ALIGN_LEFT);
graph.setCellStyles(mxConstants.STYLE_VERTICAL_LABEL_POSITION, mxConstants.ALIGN_BOTTOM);
graph.setCellStyles(mxConstants.STYLE_ALIGN, mxConstants.ALIGN_RIGHT);
graph.setCellStyles(mxConstants.STYLE_VERTICAL_ALIGN, mxConstants.ALIGN_TOP);
} else if (stringValue2.equals(mxConstants.ALIGN_RIGHT)) {
graph.setCellStyles(mxConstants.STYLE_LABEL_POSITION, mxConstants.ALIGN_RIGHT);
graph.setCellStyles(mxConstants.STYLE_VERTICAL_LABEL_POSITION, mxConstants.ALIGN_BOTTOM);
graph.setCellStyles(mxConstants.STYLE_ALIGN, mxConstants.ALIGN_LEFT);
graph.setCellStyles(mxConstants.STYLE_VERTICAL_ALIGN, mxConstants.ALIGN_TOP);
} else {
graph.setCellStyles(mxConstants.STYLE_LABEL_POSITION, mxConstants.ALIGN_CENTER);
graph.setCellStyles(mxConstants.STYLE_VERTICAL_LABEL_POSITION, mxConstants.ALIGN_BOTTOM);
graph.setCellStyles(mxConstants.STYLE_ALIGN, mxConstants.ALIGN_CENTER);
graph.setCellStyles(mxConstants.STYLE_VERTICAL_ALIGN, mxConstants.ALIGN_TOP);
}
}
} finally {
model.endUpdate();
}
GraphUtils.setElementStyles(graph, mxConstants.STYLE_LABEL_POSITION, (String) graph.getCellStyle(cell).get(mxConstants.STYLE_LABEL_POSITION), cell);
GraphUtils.setElementStyles(graph, mxConstants.STYLE_VERTICAL_LABEL_POSITION,
(String) graph.getCellStyle(cell).get(mxConstants.STYLE_VERTICAL_LABEL_POSITION), cell);
GraphUtils.setElementStyles(graph, mxConstants.STYLE_ALIGN, (String) graph.getCellStyle(cell).get(mxConstants.STYLE_ALIGN), cell);
GraphUtils.setElementStyles(graph, mxConstants.STYLE_VERTICAL_ALIGN, (String) graph.getCellStyle(cell).get(mxConstants.STYLE_VERTICAL_ALIGN), cell);
} else if (type == TOGGLE) {
graph.toggleCellStyles(stringValue, booleanValue);
GraphUtils.setElementStyles(graph, stringValue, Boolean.toString(booleanValue), null);
} else if (type == PROMPT_VALUE) {
String value = (String) JOptionPane.showInputDialog((Component) e.getSource(), Resources.get("value"), stringValue2, JOptionPane.PLAIN_MESSAGE,
null, null, "");
if (value != null) {
if (value.equals(mxConstants.NONE)) {
value = null;
}
graph.setCellStyles(stringValue, value);
GraphUtils.setElementStyles(graph, stringValue, value, null);
}
} else if (type == MULTI_PART) {
((Participant) objectValue).setMultiplicity(stringValue);
String partId = "_part_" + ((Participant) objectValue).getId();
for (Entry entry : model.getCells().entrySet()) {
if (entry.getKey().endsWith(partId)) {
double height = 0;
Object activity = null;
Object parent = model.getParent(entry.getValue());
Object[] partCells = GraphModel.getChildVertices(model, parent);
for (int i = 0; i < partCells.length; i++) {
if (model.isChoreographyParticipant(partCells[i])) {
mxCell part = (mxCell) partCells[i];
mxGeometry geo = model.getGeometry(part);
if (model.isMultiInstanceParticipant(part)) {
geo.setHeight(Constants.PARTICIPANT_HEIGHT * 1.75);
} else {
geo.setHeight(Constants.PARTICIPANT_HEIGHT);
}
model.setGeometry(part, geo);
height += geo.getHeight();
} else {
activity = partCells[i];
}
}
mxGeometry geo = model.getGeometry(parent);
geo.setHeight(model.getGeometry(activity).getHeight() + height);
model.setGeometry(parent, geo);
GraphUtils.arrangeChoreography(graph, parent, false);
graph.refresh();
graph.resetSelection();
}
}
} else if (type == DEL_PART) {
graph.removeCells(new Object[] { objectValue });
mxGeometry geo = model.getGeometry(cell);
geo.setHeight(geo.getHeight() - model.getGeometry(objectValue).getHeight());
model.setGeometry(cell, geo);
GraphUtils.arrangeChoreography(graph, cell, false);
graph.refresh();
graph.resetSelection();
} else if (type == SWAP_PART) {
Object[] partCells = mxGraphModel.getChildVertices(model, cell);
model.beginUpdate();
mxCell topPart = null;
mxCell bottomPart = null;
for (int i = 0; i < partCells.length; i++) {
if (!graph.isAdditionalChoreographyParticipant(partCells[i])) {
if (graph.isTopChoreographyParticipant(partCells[i])) {
topPart = (mxCell) partCells[i];
} else if (graph.isBottomChoreographyParticipant(partCells[i])) {
bottomPart = (mxCell) partCells[i];
}
}
}
mxGeometry geo = topPart.getGeometry();
String style = topPart.getStyle();
model.setStyle(topPart, bottomPart.getStyle());
model.setStyle(bottomPart, style);
model.setGeometry(topPart, bottomPart.getGeometry());
model.setGeometry(bottomPart, geo);
model.endUpdate();
} else if (type == INIT_PART) {
cell = GraphUtils.getChoreographyActivity(graph, cell);
ChoreographyActivity value = (ChoreographyActivity) ((ChoreographyActivity) model.getValue(cell)).clone();
value.setInitiatingParticipantRef(((Participant) objectValue).getId());
model.setValue(cell, value);
} else if (type == ADD_PART) {
double yOffset = 0;
String style = "";
mxCell subprocess = GraphUtils.getChoreographyActivity(graph, cell);
String id = subprocess.getId() + "_part_" + model.createId(null);
mxGeometry subgeo = model.getGeometry(subprocess);
if (booleanValue) {
yOffset = subgeo.getY() - 1;
style = "participantAdditionalTop";
} else {
yOffset = subgeo.getY() + subgeo.getHeight() - 1;
style = "participantAdditionalBottom";
}
if (graph.isCallChoreography(objectValue)) {
style += ";strokeWidth=3";
}
String value = "Participant";
Map participants = model.getAllParticipants();
int i = 1;
while (participants.get(value) != null) {
value = value.substring(0, 11) + " " + i++;
}
mxCell participantCell = new mxCell(new Participant(value), new mxGeometry(0, yOffset, Constants.ACTIVITY_WIDTH, Constants.PARTICIPANT_HEIGHT),
style);
participantCell.setId(id);
participantCell.setVertex(true);
graph.addCell(participantCell, (mxICell) cell);
mxGeometry geo = model.getGeometry(cell);
geo.setHeight(geo.getHeight() + Constants.PARTICIPANT_HEIGHT);
model.setGeometry(cell, geo);
GraphUtils.arrangeChoreography(graph, cell, false);
graph.refresh();
graph.resetSelection();
} else if (type == MOVE_LANE) {
if (graph != null && !graph.isSelectionEmpty()) {
Object lane = graph.getSelectionCell();
Object parent = model.getParent(lane);
int lanesize = model.getChildCount(parent);
int index = 0;
for (int i = 0; i < lanesize; i++) {
Object child = model.getChildAt(parent, i);
if (child == lane) {
index = i;
break;
}
}
if (doubleValue == 0) {
if (index == 0) {
graph.cellsAdded(new Object[] { lane }, parent, lanesize, null, null, true);
} else {
graph.cellsAdded(new Object[] { lane }, parent, index, null, null, true);
}
} else {
if (index == lanesize - 1) {
graph.cellsAdded(new Object[] { lane }, parent, 1, null, null, true);
} else {
graph.cellsAdded(new Object[] { lane }, parent, index + 2, null, null, true);
}
}
}
} else if (type == STYLE) {
model.beginUpdate();
if (objectValue == null) {
objectValue = graph.getSelectionCell();
}
if (stringValue.equals(mxConstants.ELBOW_HORIZONTAL) || stringValue.equals(mxConstants.ELBOW_VERTICAL)) {
graph.setCellStyles(mxConstants.STYLE_SHAPE, mxUtils.getString(graph.getCellStyle(objectValue), mxConstants.STYLE_SHAPE));
graph.setCellStyles(mxConstants.STYLE_EDGE, mxConstants.EDGESTYLE_ELBOW);
graph.setCellStyles(mxConstants.STYLE_ELBOW, stringValue);
} else if (stringValue.equals(Constants.FLOW_STYLE_STRAIGHT)) {
graph.setCellStyles(mxConstants.STYLE_SHAPE, mxUtils.getString(graph.getCellStyle(objectValue), mxConstants.STYLE_SHAPE));
graph.setCellStyles(mxConstants.STYLE_EDGE, mxConstants.NONE);
} else if (stringValue.equals(mxConstants.SHAPE_CURVE)) {
graph.setCellStyles(mxConstants.STYLE_EDGE, mxConstants.EDGESTYLE_ELBOW);
graph.setCellStyles(mxConstants.STYLE_SHAPE, mxConstants.SHAPE_CURVE);
} else if (stringValue.equals("resetEdgeStyle")) {
graph.setCellStyles(mxConstants.STYLE_EDGE, mxConstants.EDGESTYLE_ELBOW);
graph.setCellStyles(mxConstants.STYLE_SHAPE, mxConstants.SHAPE_CONNECTOR);
} else if (stringValue.equals(Constants.EDGE_TYPE_SEQUENCE_FLOW)) {
model.setStyle(objectValue, Constants.EDGE_TYPE_SEQUENCE_FLOW);
} else if (stringValue.equals(Constants.EDGE_TYPE_DEFAULT_SEQUENCE_FLOW)) {
model.setStyle(objectValue, Constants.EDGE_TYPE_DEFAULT_SEQUENCE_FLOW);
} else if (stringValue.equals(Constants.EDGE_TYPE_CONDITION_SEQUENCE_FLOW)) {
model.setStyle(objectValue, Constants.EDGE_TYPE_CONDITION_SEQUENCE_FLOW);
} else if (stringValue != null && stringValue.split("=").length > 1 && stringValue.lastIndexOf(";whiteSpace=") == -1) {
graph.setCellStyles(stringValue.split("=")[0], stringValue.split("=")[1], new Object[] { objectValue });
if (stringValue.split("=")[0].equals(Constants.STYLE_LOOP) || stringValue.split("=")[0].equals(Constants.STYLE_COMPENSATION)
|| stringValue.split("=")[0].equals(Constants.STYLE_TYPE)) {
if (stringValue.split("=").length > 3) {
graph.setCellStyles(stringValue.split("=")[2], stringValue.split("=")[3], new Object[] { objectValue });
}
}
} else if (model.isTask(objectValue)) {
Map oldstyle = graph.getCellStyle(objectValue);
String loop = mxUtils.getString(oldstyle, Constants.STYLE_LOOP);
String loopImage = mxUtils.getString(oldstyle, Constants.STYLE_LOOP_IMAGE);
String compensation = mxUtils.getString(oldstyle, Constants.STYLE_COMPENSATION);
String compensationImage = mxUtils.getString(oldstyle, Constants.STYLE_COMPENSATION_IMAGE);
model.setStyle(objectValue, stringValue);
if (stringValue != null && (stringValue.startsWith("task") || stringValue.startsWith("call"))) {
graph.setCellStyles(Constants.STYLE_LOOP, loop);
graph.setCellStyles(Constants.STYLE_LOOP_IMAGE, loopImage);
graph.setCellStyles(Constants.STYLE_COMPENSATION, compensation);
graph.setCellStyles(Constants.STYLE_COMPENSATION_IMAGE, compensationImage);
}
} else {
model.setStyle(objectValue, stringValue);
if (model.isStartEvent(objectValue) && model.isEventSubProcess(model.getParent(objectValue))) {
int endIndex = stringValue.lastIndexOf("Event");
if (endIndex != -1) {
String trigger = stringValue.substring(5, endIndex);
graph.setCellStyles("trigger", trigger, new Object[] { model.getParent(objectValue) });
}
}
}
model.endUpdate();
graph.fireEvent(new mxEventObject(mxEvent.MOVE_CELLS, "cells", new Object[] { objectValue }));
if (stringValue.startsWith(Constants.STYLE_LOOP + "=")) {
graph.fireEvent(new mxEventObject(Constants.EVENT_LOOPTYPE_CHANGED, "cells", new Object[] { objectValue }));
} else if (stringValue.startsWith(Constants.STYLE_INSTANTIATE) || stringValue.startsWith(Constants.STYLE_COMPENSATION)
|| stringValue.startsWith(Constants.ACTIVITY_STYLE_LOOP_MI) || stringValue.startsWith(Constants.STYLE_LOOP_IMAGE)) {
graph.fireEvent(new mxEventObject(Constants.EVENT_ATTR_CHANGED, "cells", new Object[] { objectValue }));
} else {
if (!model.isEdge(objectValue)) {
graph.fireEvent(new mxEventObject(mxEvent.CHANGE, "cells", new Object[] { objectValue }));
}
}
} else if (type == RESET_BACKGROUND) {
GraphUtils.setElementStyles(graph, null, null, null);
graph.setCellStyles(mxConstants.STYLE_FILLCOLOR, null);
graph.setCellStyles(mxConstants.STYLE_GRADIENTCOLOR, null);
graph.setCellStyles(mxConstants.STYLE_OPACITY, null);
} else if (type == AUTO_LAYOUT) {
final mxIGraphLayout layout = new BPMNLayout(graph);
if (layout instanceof BPMNLayout) {
int nodeDistance = Integer.parseInt(Constants.SETTINGS.getProperty("nodeDistance", "20"));
int levelDistance = Integer.parseInt(Constants.SETTINGS.getProperty("levelDistance", "40"));
((BPMNLayout) layout).setNodeDistance(nodeDistance);
((BPMNLayout) layout).setLevelDistance(levelDistance);
}
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy