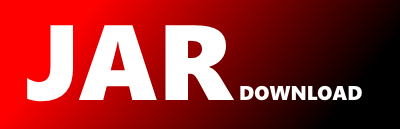
org.yaoqiang.graph.io.vdx.VdxCodecUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yaoqiang-bpmn-editor Show documentation
Show all versions of yaoqiang-bpmn-editor Show documentation
an Open Source BPMN 2.0 Modeler
package org.yaoqiang.graph.io.vdx;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import org.yaoqiang.graph.view.Graph;
import org.yaoqiang.util.Constants;
import com.mxgraph.model.mxCell;
import com.mxgraph.model.mxGeometry;
import com.mxgraph.util.mxPoint;
/**
* VdxCodecUtils
*
* @author Shi Yaoqiang([email protected])
*/
public class VdxCodecUtils {
private static double screenCoordinatesPerCm = 40;
private static final double CENTIMETERS_PER_INCHES = 2.54;
public static List nodeListTags(NodeList nl, String tag) {
ArrayList ret = new ArrayList();
if (nl != null) {
int length = nl.getLength();
for (int i = 0; i < length; i++) {
if (tag.equals(nl.item(i).getNodeName())) {
Node type = nl.item(i).getAttributes().getNamedItem(VdxConstants.TYPE);
if (!type.getNodeValue().equals(VdxConstants.TYPE_FOREIGN)) {
ret.add((Element) nl.item(i));
}
}
}
}
return ret;
}
public static boolean nodeListHasTag(NodeList nl, String tag) {
boolean has = false;
if (nl != null) {
int length = nl.getLength();
for (int i = 0; (i < length) && !has; i++) {
has = (nl.item(i)).getNodeName().equals(tag);
}
}
return has;
}
public static Element nodeListTag(NodeList nl, String tag) {
if (nl != null) {
int length = nl.getLength();
boolean has = false;
for (int i = 0; (i < length) && !has; i++) {
has = (nl.item(i)).getNodeName().equals(tag);
if (has) {
return (Element) nl.item(i);
}
}
}
return null;
}
public static Element nodeListTagIndexed(NodeList nl, String tag, String ix) {
if (nl != null) {
int length = nl.getLength();
boolean has = false;
for (int i = 0; (i < length) && !has; i++) {
has = (nl.item(i)).getNodeName().equals(tag) && ((Element) (nl.item(i))).getAttribute("IX").equals(ix);
if (has) {
return (Element) nl.item(i);
}
}
}
return null;
}
public static String getShapeIdByNameU(Map vertexShapeMap, String nameU) {
String id = "";
for (VdxShape shape : vertexShapeMap.values()) {
if (shape.getNameU().equals(nameU)) {
return shape.getId();
}
}
return id;
}
public static Map getShapePropMap(NodeList nl) {
Map props = new HashMap();
if (nl != null) {
int length = nl.getLength();
for (int i = 0; i < length; i++) {
if (VdxConstants.PROP.equals(nl.item(i).getNodeName())) {
Element propElement = (Element) nl.item(i);
String name = propElement.getAttribute(VdxConstants.NAME_U);
Element valElement = nodeListTag(propElement.getChildNodes(), VdxConstants.VALUE);
String value = valElement.getTextContent();
props.put(name, value);
}
}
}
return props;
}
public static List copyNodeList(NodeList nodeList) {
ArrayList copy = new ArrayList();
int length = nodeList.getLength();
for (int i = 0; i < length; i++) {
copy.add(nodeList.item(i));
}
return copy;
}
public static void adjustCellDimentions(Graph graph, mxCell cell) {
mxGeometry geo = cell.getGeometry();
if (graph.getModel().isEvent(cell)) {
geo.setWidth(32);
geo.setHeight(32);
} else if (graph.getModel().isGateway(cell)) {
geo.setWidth(42);
geo.setHeight(42);
} else if (graph.getModel().isDataObject(cell)) {
geo.setWidth(30);
geo.setHeight(38);
} else if (graph.getModel().isDataStore(cell)) {
geo.setWidth(35);
geo.setHeight(30);
} else if (graph.getModel().isConversationNode(cell)) {
geo.setWidth(40);
geo.setHeight(35);
} else if (graph.getModel().isTask(cell) || graph.getModel().isCallActivity(cell)) {
if (geo.getWidth() <= Constants.ACTIVITY_WIDTH) {
geo.setWidth(Constants.ACTIVITY_WIDTH);
}
if (geo.getHeight() <= Constants.ACTIVITY_HEIGHT) {
geo.setHeight(Constants.ACTIVITY_HEIGHT);
}
} else if (graph.getModel().isChoreographyParticipant(cell)) {
if (graph.getModel().isMultiInstanceParticipant(cell)) {
geo.setHeight(Constants.PARTICIPANT_HEIGHT * 1.75);
} else {
geo.setHeight(Constants.PARTICIPANT_HEIGHT);
}
}
}
public static mxPoint adjustConstraint(mxPoint constraint) {
constraint.setX(Math.max(0, constraint.getX()));
constraint.setY(Math.max(0, constraint.getY()));
constraint.setX(Math.min(1, constraint.getX()));
constraint.setY(Math.min(1, constraint.getY()));
return constraint;
}
public static double conversionFactor() {
double ret = 0;
ret = screenCoordinatesPerCm * CENTIMETERS_PER_INCHES;
return ret;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy