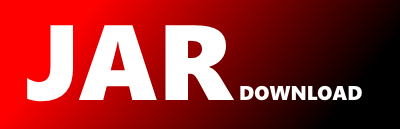
org.yaoqiang.collaboration.ComboPanel Maven / Gradle / Ivy
package org.yaoqiang.collaboration;
import java.awt.BorderLayout;
import java.awt.Dimension;
import java.awt.event.ActionListener;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.Vector;
import javax.swing.BorderFactory;
import javax.swing.Box;
import javax.swing.JComboBox;
import javax.swing.JLabel;
import javax.swing.JPanel;
import org.yaoqiang.util.Resources;
/**
* ComboPanel
*
* @author Shi Yaoqiang([email protected])
*/
public class ComboPanel extends JPanel {
private static final long serialVersionUID = -2708869980534018181L;
Dimension textDim = new Dimension(120, 27);
protected String key;
protected JComboBox jcb;
public ComboPanel(String key, Collection> choices, boolean hasEmpty, boolean isEditable, boolean isEnabled) {
this.setLayout(new BorderLayout());
this.setBorder(BorderFactory.createEmptyBorder(5, 5, 5, 5));
this.key = key;
JLabel jl = new JLabel(Resources.get(key) + ": ");
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy