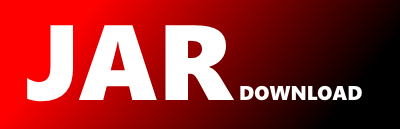
org.yaoqiang.graph.view.GraphView Maven / Gradle / Ivy
package org.yaoqiang.graph.view;
import java.util.Map;
import org.yaoqiang.graph.model.GraphModel;
import org.yaoqiang.util.Constants;
import com.mxgraph.model.mxCell;
import com.mxgraph.model.mxGeometry;
import com.mxgraph.model.mxIGraphModel;
import com.mxgraph.util.mxConstants;
import com.mxgraph.util.mxPoint;
import com.mxgraph.util.mxUtils;
import com.mxgraph.view.mxCellState;
import com.mxgraph.view.mxGraph;
import com.mxgraph.view.mxGraphView;
/**
* GraphView
*
* @author Shi Yaoqiang([email protected])
*/
public class GraphView extends mxGraphView {
public GraphView(mxGraph graph) {
super(graph);
}
public Graph getGraph() {
return (Graph) graph;
}
public double getWordWrapWidth(mxCellState state) {
Map style = state.getStyle();
String shape = mxUtils.getString(style, mxConstants.STYLE_SHAPE, "label");
if (shape.equals("event") || shape.equals("gateway") || shape.equals("dataobject") || shape.equals("datastore") || shape.equals("conversation") || "message".equals(style.get(Constants.STYLE_TYPE))) {
return 100;
}
return super.getWordWrapWidth(state);
}
public void validateBounds(mxCellState parentState, Object cell) {
mxIGraphModel model = graph.getModel();
mxCellState state = getState(cell, true);
if (state != null && state.isInvalid()) {
if (!graph.isCellVisible(cell)) {
removeState(cell);
} else if (cell != currentRoot && parentState != null) {
state.getAbsoluteOffset().setX(0);
state.getAbsoluteOffset().setY(0);
state.setOrigin(new mxPoint(parentState.getOrigin()));
mxGeometry geo = graph.getCellGeometry(cell);
if (geo != null) {
if (!model.isEdge(cell)) {
mxPoint origin = state.getOrigin();
mxPoint offset = geo.getOffset();
if (offset == null) {
offset = EMPTY_POINT;
}
if (geo.isRelative()) {
origin.setX(origin.getX() + geo.getX() * parentState.getWidth() / scale + offset.getX());
origin.setY(origin.getY() + geo.getY() * parentState.getHeight() / scale + offset.getY());
} else {
state.setAbsoluteOffset(new mxPoint(scale * offset.getX(), scale * offset.getY()));
origin.setX(origin.getX() + geo.getX());
origin.setY(origin.getY() + geo.getY());
}
}
// Updates the cell state's bounds
state.setX(scale * (translate.getX() + state.getOrigin().getX()));
state.setY(scale * (translate.getY() + state.getOrigin().getY()));
state.setWidth(scale * geo.getWidth());
state.setHeight(scale * geo.getHeight());
if (model.isVertex(cell)) {
updateVertexLabelOffset(state);
}
// Updates the cached label
updateLabel(state);
}
}
// Applies child offset to origin
mxPoint offset = graph.getChildOffsetForCell(cell);
if (offset != null) {
state.getOrigin().setX(state.getOrigin().getX() + offset.getX());
state.getOrigin().setY(state.getOrigin().getY() + offset.getY());
}
}
// Recursively validates the child bounds
if (state != null && (!graph.isCellCollapsed(cell) || cell == currentRoot)) {
// ==============start==============
if (cell != currentRoot && ((mxCell) cell).getGeometry() != null
&& (getGraph().getModel().isCollapsedSubProcess(cell) || getGraph().isCollapsedSwimlane(cell))) {
int childCount = model.getChildCount(cell);
for (int i = 0; i < childCount; i++) {
Object child = model.getChildAt(cell, i);
if (getGraph().getModel().isBoundaryEvent(child)) {
validateBounds(state, child);
}
}
}
// ==============end================
else {
int childCount = model.getChildCount(cell);
for (int i = 0; i < childCount; i++) {
Object child = model.getChildAt(cell, i);
if (cell == currentRoot && getGraph().getModel().isBoundaryEvent(child)) {
continue;
}
validateBounds(state, child);
}
}
}
}
public Object getVisibleTerminal(Object edge, boolean source) {
GraphModel model = getGraph().getModel();
Object result = model.getTerminal(edge, source);
Object best = result;
while (result != null && result != currentRoot) {
if (!graph.isCellVisible(best) || graph.isCellCollapsed(result) || model.isCollapsedSubProcess(result)
&& !model.isBoundaryEvent(model.getTerminal(edge, source))) {
best = result;
}
result = model.getParent(result);
}
// Checks if the result is not a layer
if (model.getParent(best) == model.getRoot()) {
best = null;
}
return best;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy