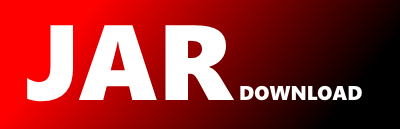
org.yaoqiang.graph.io.graphml.GraphMLUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yaoqiang-bpmn-editor Show documentation
Show all versions of yaoqiang-bpmn-editor Show documentation
an Open Source BPMN 2.0 Modeler
package org.yaoqiang.graph.io.graphml;
import java.awt.Point;
import java.awt.Rectangle;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import com.mxgraph.model.mxCell;
import com.mxgraph.util.mxPoint;
/**
* GraphMLUtils
*
* @author Shi Yaoqiang([email protected])
*/
public class GraphMLUtils {
public static List childsTags(Element element, String tag) {
NodeList nl = element.getChildNodes();
ArrayList ret = new ArrayList();
if (nl != null) {
int length = nl.getLength();
for (int i = 0; i < length; i++) {
if (tag.equals((nl.item(i)).getNodeName())) {
ret.add((Element) nl.item(i));
}
}
}
return ret;
}
/**
* Checks if the NodeList has a Node with name = tag.
*
* @param nl
* NodeList
* @param tag
* Name of the node.
* @return Returns true
if the Node List has a Node with name = tag.
*/
public static boolean nodeListHasTag(NodeList nl, String tag) {
boolean has = false;
if (nl != null) {
int length = nl.getLength();
for (int i = 0; (i < length) && !has; i++) {
has = (nl.item(i)).getNodeName().equals(tag);
}
}
return has;
}
/**
* Returns the first Element that has name = tag in Node List.
*
* @param nl
* NodeList
* @param tag
* Name of the Element
* @return Element with name = 'tag'.
*/
public static Element nodeListTag(NodeList nl, String tag) {
if (nl != null) {
int length = nl.getLength();
boolean has = false;
for (int i = 0; (i < length) && !has; i++) {
has = (nl.item(i)).getNodeName().equals(tag);
if (has) {
return (Element) nl.item(i);
}
}
}
return null;
}
/**
* Returns a list with the elements included in the Node List that have name = tag.
*
* @param nl
* NodeList
* @param tag
* name of the Element.
* @return List with the indicated elements.
*/
public static List nodeListTags(NodeList nl, String tag) {
ArrayList ret = new ArrayList();
if (nl != null) {
int length = nl.getLength();
for (int i = 0; i < length; i++) {
if (tag.equals((nl.item(i)).getNodeName())) {
ret.add((Element) nl.item(i));
}
}
}
return ret;
}
/**
* Checks if the childrens of element has a Node with name = tag.
*
* @param element
* Element
* @param tag
* Name of the node.
* @return Returns true
if the childrens of element has a Node with name = tag.
*/
public static boolean childsHasTag(Element element, String tag) {
NodeList nl = element.getChildNodes();
boolean has = false;
if (nl != null) {
int length = nl.getLength();
for (int i = 0; (i < length) && !has; i++) {
has = (nl.item(i)).getNodeName().equals(tag);
}
}
return has;
}
/**
* Returns the first Element that has name = tag in the childrens of element.
*
* @param element
* Element
* @param tag
* Name of the Element
* @return Element with name = 'tag'.
*/
public static Element childsTag(Element element, String tag) {
NodeList nl = element.getChildNodes();
if (nl != null) {
int length = nl.getLength();
boolean has = false;
for (int i = 0; (i < length) && !has; i++) {
has = (nl.item(i)).getNodeName().equals(tag);
if (has) {
return (Element) nl.item(i);
}
}
}
return null;
}
public static List copyNodeList(NodeList nodeList) {
ArrayList copy = new ArrayList();
int length = nodeList.getLength();
for (int i = 0; i < length; i++) {
copy.add(nodeList.item(i));
}
return copy;
}
public static String getStyleFromNode(GraphMLGenericNode node) {
String style = "whiteSpace=wrap;";
if (node.isEvent()) {
if (node.isStartEvent() || node.isEventStartEvent() || node.isNonInterruptingEventStartEvent()) {
style = "start";
} else if (node.isEntEvent()) {
style = "end";
} else if (node.isIntermediateCatchEvent() || node.isIntermediateThrowEvent() || node.isBoundaryEvent() || node.isNonInterruptingBoundaryEvent()) {
style = "intermediate";
}
if (node.isMessageEvent()) {
style += "Message";
} else if (node.isTimerEvent()) {
style += "Timer";
} else if (node.isErrorEvent()) {
style += "Error";
} else if (node.isCancelEvent()) {
style += "Cancel";
} else if (node.isCompensationEvent()) {
style += "Compensation";
} else if (node.isConditionalEvent()) {
style += "Conditional";
} else if (node.isSignalEvent()) {
style += "Signal";
} else if (node.isLinkEvent()) {
style += "Link";
} else if (node.isMultipleEvent()) {
style += "Multiple";
} else if (node.isParallelMultipleEvent()) {
style += "ParallelMultiple";
} else if (node.isTerminateEvent()) {
style += "Terminate";
}
if (node.isIntermediateThrowEvent()) {
style += "Throw";
} else if (node.isNonInterruptingEventStartEvent() || node.isNonInterruptingBoundaryEvent()) {
style += "NonInterrupting";
}
style += "Event";
} else if (node.isGateway()) {
if (node.isGatewayWithoutMarker()) {
style += "exclusiveGateway";
}
if (node.isExclusiveGateway()) {
style += "exclusiveGatewayWithIndicator";
} else if (node.isInclusiveGateway()) {
style += "inclusiveGateway";
} else if (node.isParallelGateway()) {
style += "parallelGateway";
} else if (node.isComplexGateway()) {
style += "complexGateway";
} else if (node.isParallelEventGateway()) {
style += "parallelEventGateway";
} else if (node.isInstantiateEventGateway()) {
style += "eventGatewayInstantiate";
} else if (node.isEventGateway()) {
style += "eventGateway";
}
} else if (node.isConversation()) {
if (node.isSubConversation()) {
if (node.isCallConversation()) {
style = "callCollaboration;";
} else {
style = "subConversation;";
}
} else {
if (node.isCallConversation()) {
style = "callConversation;";
} else {
style = "conversation;";
}
}
} else if (node.isArtifact()) {
if (node.isDataObject()) {
if (node.isDataInput()) {
style = "datainput";
} else if (node.isDataOutput()) {
style = "dataoutput";
} else {
style = "dataobject";
}
if (node.isCollectionDataObject()) {
style += "s;";
}
} else if (node.isDataStore()) {
style = "datastore;";
} else if (node.isInitiatingMessage()) {
style = "initiatingMessage;";
} else if (node.isMessage()) {
style = "nonInitiatingMessage;";
} else if (node.isAnnotation()) {
style = "annotation;";
} else if (node.isGroup()) {
style = "group;";
}
} else if (node.isActivity()) {
if (node.isCompensationActivity()) {
style += "compensation=1;compensationImage=/org/yaoqiang/graph/images/marker_compensation.png;";
}
if (node.isTransaction()) {
style += "tranSubprocess;";
} else if (node.isEventSubProcess()) {
style += "eventSubprocess;";
} else if (node.isCallActivity()) {
if (node.isCallSubProcess()) {
style += "callSubprocess;";
} else if (node.isBusinessRuleTask()) {
style += "callBusinessRule;";
} else if (node.isScriptTask()) {
style += "callScript;";
} else if (node.isManualTask()) {
style += "callManual;";
} else if (node.isUserTask()) {
style += "callUser;";
} else {
style += "callActivity;";
}
} else if (node.isAdHocActivity()) {
style += "adHocSubprocess;";
} else if (node.isSubProcess()) {
style += "subprocess;";
} else if (node.isTask()) {
if (node.isSendTask()) {
style += "taskSend;";
} else if (node.isReceiveTask()) {
style += "taskReceive;";
} else if (node.isUserTask()) {
style += "taskUser;";
} else if (node.isManualTask()) {
style += "taskManual;";
} else if (node.isBusinessRuleTask()) {
style += "taskBusinessRule;";
} else if (node.isServiceTask()) {
style += "taskService;";
} else if (node.isScriptTask()) {
style += "taskScript;";
} else {
style += "task;";
}
}
if (node.isLoopActivity()) {
style += "loop=standard;loopImage=/org/yaoqiang/graph/images/marker_loop.png;";
} else if (node.isMultiInstanceParallelActivity()) {
style += "loop=multi_instance;loopImage=/org/yaoqiang/graph/images/marker_multiple.png;";
} else if (node.isMultiInstanceSequentialActivity()) {
style += "loop=multi_instance_sequential;loopImage=/org/yaoqiang/graph/images/marker_multiple_sequential.png;";
}
}
return style;
}
public static String getStyleFromEdge(GraphMLGenericEdge edge) {
String style = "";
if (edge.isSequenceFlow()) {
style = "defaultEdge;";
} else if (edge.isConditionalSequenceFlow()) {
style = "conditionFlow;";
} else if (edge.isDefaultSequenceFlow()) {
style = "defaultFlow;";
} else if (edge.isAssociation()) {
style = "association;";
} else if (edge.isDataAssociation()) {
style = "dataAssociation;";
} else if (edge.isMessageFlow()) {
style = "messageFlow;";
} else if (edge.isConversationLink()) {
style = "conversationLink;";
}
return style;
}
public static Rectangle getGeometryFromNode(GraphMLGenericNode node, mxCell parent) {
Rectangle geo = node.getGeometry();
if (node.isEvent()) {
geo.setSize(32, 32);
} else if (node.isGateway()) {
geo.setSize(42, 42);
} else if (node.isDataObject()) {
geo.setSize(29, 38);
} else if (node.isConversation()) {
geo.setSize(40, 35);
}
if (parent != null && parent.getGeometry() != null) {
Point ploc = parent.getGeometry().getPoint();
geo.setLocation((int) (geo.getX() - ploc.getX()), (int) (geo.getY() - ploc.getY()));
}
return geo;
}
public static List getControlPointsFromEdge(GraphMLGenericEdge edge, mxCell parent) {
List mxPoints = new ArrayList();
List points = edge.getPoints();
for (Point p : points) {
mxPoint cp = new mxPoint(p);
if (parent != null && parent.getGeometry() != null) {
Point ploc = parent.getGeometry().getPoint();
cp.setX(cp.getX() - ploc.getX());
cp.setY(cp.getY() - ploc.getY());
}
mxPoints.add(cp);
}
return mxPoints;
}
public static HashMap getStyleMap(String style, String asig) {
HashMap styleMap = new HashMap();
String key = "";
String value = "";
int index = 0;
if (!style.equals("")) {
String[] entries = style.split(";");
for (String entry : entries) {
index = entry.indexOf(asig);
if (index == -1) {
key = "";
value = entry;
styleMap.put(key, value);
} else {
key = entry.substring(0, index);
value = entry.substring(index + 1);
styleMap.put(key, value);
}
}
}
return styleMap;
}
public static String getStyleString(Map styleMap, String asig) {
String style = "";
Iterator
© 2015 - 2025 Weber Informatics LLC | Privacy Policy