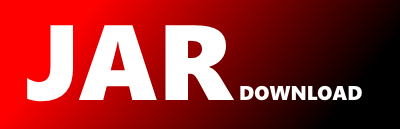
org.yaoqiang.graph.util.TooltipBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yaoqiang-bpmn-editor Show documentation
Show all versions of yaoqiang-bpmn-editor Show documentation
an Open Source BPMN 2.0 Modeler
package org.yaoqiang.graph.util;
import java.util.Iterator;
import java.util.LinkedHashMap;
import java.util.Map;
import org.w3c.dom.Element;
import org.yaoqiang.bpmn.model.elements.core.common.SequenceFlow;
import org.yaoqiang.bpmn.model.elements.data.ItemAwareElement;
import org.yaoqiang.graph.model.GraphModel;
import org.yaoqiang.graph.view.Graph;
import org.yaoqiang.util.Constants;
import org.yaoqiang.util.Resources;
import com.mxgraph.model.mxCell;
import com.mxgraph.util.mxUtils;
/**
* TooltipBuilder
*
* @author Shi Yaoqiang([email protected])
*/
public class TooltipBuilder {
public static final String EMPTY_STRING = "";
public static final String EMPTY_VALUE = "empty";
public static final String HTML_OPEN = "";
public static final String HTML_CLOSE = "";
public static final String DIV_OPEN = "";
public static final String DIV_CLOSE = "";
public static final String STRONG_OPEN = "";
public static final String STRONG_CLOSE = "";
public static final String LINE_BREAK = "
";
public static final String COLON_SPACE = ": ";
public String getTooltip(Graph graph, mxCell cell) {
GraphModel model = graph.getModel();
Map toDisplay = new LinkedHashMap();
String title = "Element";
String process = cell.getAttribute("process");
String attached = cell.getAttribute("attached");
String call = cell.getAttribute("call");
String cancelActivity = "";
if (model.isBoundaryEvent(cell)) {
// cancelActivity = graph.cancelActivity(cell) ? "true" : "false";
}
String compensation = "";
if ((model.isTask(cell) || model.isSubProcess(cell) || model.isCallActivity(cell)) && !model.isChoreographySubprocess(cell)
&& !model.isChoreographyTask(cell)) {
// compensation = graph.isForCompensation(cell) ? "true" : "false";
}
String loop = "";
String sequential = "";
String looptype = mxUtils.getString(graph.getCellStyle(cell), Constants.STYLE_LOOP, "");
if (looptype.equals(Constants.ACTIVITY_STYLE_LOOP_STANDARD)) {
loop = "Standard";
} else if (looptype.equals(Constants.ACTIVITY_STYLE_LOOP_MI)) {
if (model.isChoreographyTask(cell) || model.isChoreographySubprocess(cell)) {
loop = "Parallel Multi-Instance";
} else {
loop = "Multi-Instance";
sequential = "false";
}
} else if (looptype.equals(Constants.ACTIVITY_STYLE_LOOP_MI_SEQUENTIAL)) {
if (model.isChoreographyTask(cell) || model.isChoreographySubprocess(cell)) {
loop = "Sequential Multi-Instance";
} else {
loop = "Multi-Instance";
sequential = "true";
}
}
String instantiate = "";
String eventGatewayType = "";
if (model.isEventGateway(cell)) {
instantiate = Boolean.toString(model.isInstantiateEventGateway(cell));
eventGatewayType = model.isParallelEventGateway(cell) ? "Parallel" : "Exclusive";
}
if (model.isReceiveTask(cell)) {
instantiate = Boolean.toString(model.isInstantiateReceiveTask(cell));
}
String collection = model.isDataObject(cell) ? Boolean.toString(model.isCollectionDataObject(cell)) : "";
String source = cell.getSource() != null ? cell.getSource().getId() : "";
String target = cell.getTarget() != null ? cell.getTarget().getId() : "";
String value = cell.getValue() instanceof Element ? cell.getAttribute("value") : cell.getValue() != null ? cell.getValue().toString() : "";
if (model.isPool(cell)) {
title = "Participant (Pool)";
} else if (model.isLane(cell)) {
title = "Lane";
} else if (model.isStartEvent(cell)) {
title = "Start Event";
if (model.isMultipleEvent(cell)) {
title = "Multiple Start Event";
}
} else if (model.isIntermediateEvent(cell)) {
if (model.isBoundaryEvent(cell)) {
title = "Boundary Event";
if (model.isMultipleEvent(cell)) {
title = "Boundary Multiple Event";
}
} else {
if (model.isIntermediateCatchEvent(cell)) {
title = "Intermediate Catch Event";
} else if (model.isIntermediateThrowEvent(cell)) {
title = "Intermediate Throw Event";
}
if (model.isMultipleEvent(cell)) {
title = "Multiple " + title;
}
}
} else if (model.isEndEvent(cell)) {
title = "End Event";
if (model.isMultipleEvent(cell)) {
title = "Multiple End Event";
}
} else if (model.isGateway(cell)) {
title = "Exclusive Gateway";
if (model.isInclusiveGateway(cell)) {
title = "Inclusive Gateway";
} else if (model.isParallelGateway(cell)) {
title = "Parallel Gateway";
} else if (model.isComplexGateway(cell)) {
title = "Complex Gateway";
} else if (model.isEventGateway(cell)) {
title = "Event-Based Gateway";
}
} else if (model.isTask(cell)) {
title = "Task";
if (model.isChoreographyTask(cell)) {
title = "Choreography Task";
} else if (model.isSendTask(cell)) {
title = "Send Task";
} else if (model.isReceiveTask(cell)) {
title = "Receive Task";
} else if (model.isServiceTask(cell)) {
title = "Service Task";
} else if (model.isUserTask(cell)) {
title = "User Task";
} else if (model.isScriptTask(cell)) {
title = "Script Task";
} else if (model.isManualTask(cell)) {
title = "Manual Task";
} else if (model.isBusinessRuleTask(cell)) {
title = "Business Rule Task";
}
} else if (model.isSubProcess(cell)) {
title = "Sub-Process";
if (model.isChoreographySubprocess(cell)) {
title = "Sub-Choreography";
}
} else if (model.isCallActivity(cell)) {
title = "Call Activity";
if (model.isChoreographySubprocess(cell)) {
title = "Call Choreography";
}
} else if (model.isChoreographyParticipant(cell)) {
title = "Participant";
} else if (model.isConversationNode(cell)) {
title = "Conversation";
if (model.isSubConversation(cell)) {
title = "Sub Conversation";
}
if (model.isCallConversation(cell)) {
title = "Call Conversation";
}
} else if (model.isGroupArtifact(cell)) {
title = "Group";
} else if (model.isAnnotation(cell)) {
title = "Text Annotation";
} else if (model.isDataObject(cell)) {
title = "Data Object";
} else if (model.isDataInput(cell)) {
title = "Data Input";
} else if (model.isDataOutput(cell)) {
title = "Data Output";
} else if (model.isDataStore(cell)) {
title = "Data Store";
} else if (model.isAssociation(cell)) {
title = "Association";
} else if (model.isDataAssociation(cell)) {
title = "Data Association";
} else if (model.isSequenceFlow(cell)) {
title = "Sequence Flow";
} else if (model.isMessageFlow(cell)) {
title = "Message Flow";
} else if (model.isMessage(cell)) {
title = "Message";
} else if (model.isConversationLink(cell)) {
title = "Conversation Link";
} else if (graph.isCompensationAssociation(cell)) {
title = "Compensation Association";
}
toDisplay.put("title", title);
if (model.isChoreographyParticipant(cell)) {
toDisplay.put("id", cell.getId().substring(cell.getId().indexOf("_part_") + 6));
} else {
toDisplay.put("id", cell.getId());
}
if (model.isAnnotation(cell)) {
toDisplay.put("text", value);
} else if (model.isDataObject(cell)) {
toDisplay.put("name", value);
toDisplay.put("state", ((ItemAwareElement) cell.getValue()).getDataState());
} else {
toDisplay.put("name", value);
}
// toDisplay.put("style", cell.getStyle());
if (process != null && process.length() != 0) {
toDisplay.put("processRef", process);
}
if (attached != null && attached.length() != 0) {
toDisplay.put("attachedToRef", attached);
}
if (cancelActivity != null && cancelActivity.length() != 0) {
toDisplay.put("cancelActivity", cancelActivity);
}
if (eventGatewayType != null && eventGatewayType.length() != 0) {
toDisplay.put("event gateway type", eventGatewayType);
}
if (instantiate != null && instantiate.length() != 0) {
toDisplay.put("instantiate", instantiate);
}
if (collection != null && collection.length() != 0) {
toDisplay.put("isCollection", collection);
}
if (compensation != null && compensation.length() != 0) {
toDisplay.put("isForCompensation", compensation);
}
if (loop != null && loop.length() != 0) {
toDisplay.put("loop type", loop);
if (sequential != null && sequential.length() != 0) {
toDisplay.put("isSequential", sequential);
}
}
if (call != null && call.length() != 0) {
toDisplay.put("calledElement", call);
}
if (source != null && source.length() != 0) {
toDisplay.put("sourceRef", source);
}
if (target != null && target.length() != 0) {
toDisplay.put("targetRef", target);
}
if (model.isConditionalSequenceFlow(cell)) {
toDisplay.put("conditionExpression", ((SequenceFlow) cell.getValue()).getConditionExpression().toValue());
}
return makeTooltip(toDisplay);
}
public static String getQuickHintsForElement(String name) {
String s = HTML_OPEN;
s += makeHtmlTitleLine(Resources.get(name));
if (name.startsWith("vertical")) {
name = name.substring(8).toLowerCase();
} else if (name.equals("startNonInterruptingEvent")) {
name = "startEvent";
} else if (name.startsWith("intermediate")) {
name = "intermediateEvent";
} else if (name.startsWith("boundary")) {
name = "boundaryEvent";
} else if (name.startsWith("exclusiveGateway")) {
name = "exclusiveGateway";
} else if (name.startsWith("callChoreography")) {
name = "callChoreography";
} else if (name.equals("callCollaboration")) {
name = "callConversation";
} else if (name.startsWith("dataInput")) {
name = "dataInput";
} else if (name.startsWith("dataOutput")) {
name = "dataOutput";
} else if (name.endsWith("Message")) {
name = "message";
} else if (name.startsWith("call")) {
name = "callActivity";
}
if (!name.startsWith("organization")) {
s += Resources.get(name + "Desc");
}
s += HTML_CLOSE;
return s;
}
/**
* Neat little thing. Makes HTML formated string for tooltip (made of property names and coresponding values).
*/
protected static String makeTooltip(Map elements) {
if (elements == null)
return "";
String s = HTML_OPEN;
Iterator> it = elements.entrySet().iterator();
Map.Entry me = it.next();
s += makeHtmlTitleLine(me.getValue());
while (it.hasNext()) {
me = it.next();
s += makeAnotherHtmlLine(me.getKey(), me.getValue());
}
s = s.substring(0, s.length() - LINE_BREAK.length());
s += HTML_CLOSE;
return s;
}
/** Helps when generating tooltip for some element title. */
protected static String makeHtmlTitleLine(String title) {
String textToAppend = "";
textToAppend += DIV_OPEN;
textToAppend += STRONG_OPEN;
textToAppend += title;
textToAppend += STRONG_CLOSE;
textToAppend += DIV_CLOSE;
return textToAppend;
}
/** Helps when generating tooltip for some element. */
protected static String makeAnotherHtmlLine(String label, String text) {
int MAX_LENGTH = 45;
int MAX_LINES_PER_TEXT = 0;
String textToAppend = "";
textToAppend += STRONG_OPEN;
textToAppend += label + COLON_SPACE;
textToAppend += STRONG_CLOSE;
String val = text;
val = val.replaceAll("<", "<");
val = val.replaceAll(">", ">");
int vl = val.length();
if (vl > MAX_LENGTH) {
String newVal = "";
int hm = vl / MAX_LENGTH;
for (int i = 0; i <= hm; i++) {
int startI = i * MAX_LENGTH;
int endI = (i + 1) * MAX_LENGTH;
if (endI > vl) {
endI = vl;
}
newVal = newVal + val.substring(startI, endI);
if (i == MAX_LINES_PER_TEXT) {
newVal = newVal + " ...";
break;
}
if (i < hm) {
newVal += LINE_BREAK;
newVal += makeEmptyHTMLText((label + COLON_SPACE).length());
}
}
val = newVal;
} else if (vl == 0) {
val = EMPTY_VALUE;
}
textToAppend += val;
textToAppend += LINE_BREAK;
return textToAppend;
}
protected static String makeEmptyHTMLText(int length) {
if (length < 0)
return null;
String es = "";
for (int i = 0; i < length; i++) {
es += " ";
}
return es;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy