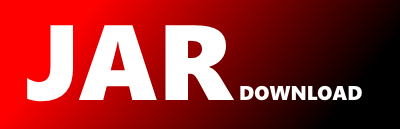
org.yaoqiang.graph.io.graphml.GraphMLGraph Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yaoqiang-bpmn-editor Show documentation
Show all versions of yaoqiang-bpmn-editor Show documentation
an Open Source BPMN 2.0 Modeler
package org.yaoqiang.graph.io.graphml;
import java.awt.Rectangle;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import org.w3c.dom.Element;
import com.mxgraph.model.mxCell;
import com.mxgraph.model.mxGeometry;
import com.mxgraph.util.mxRectangle;
import com.mxgraph.view.mxGraph;
/**
* GraphMLGraph
*
* @author Shi Yaoqiang([email protected])
*/
public class GraphMLGraph {
private static HashMap cellsMap = new HashMap();
private String id = "";
private String edgedefault = "";
private List nodes = new ArrayList();
private List edges = new ArrayList();
public GraphMLGraph(Element graphElement) {
this.id = graphElement.getAttribute(GraphMLConstants.ID);
this.edgedefault = graphElement.getAttribute(GraphMLConstants.EDGE_DEFAULT);
List nodeElements = GraphMLUtils.childsTags(graphElement, GraphMLConstants.NODE);
for (Element el : nodeElements) {
GraphMLNode node = new GraphMLNode(el);
nodes.add(node);
}
List edgeElements = GraphMLUtils.childsTags(graphElement, GraphMLConstants.EDGE);
for (Element el : edgeElements) {
GraphMLEdge edge = new GraphMLEdge(el);
edges.add(edge);
}
}
public void addGraph(mxGraph graph, Object parent) {
List nodeList = getNodes();
for (GraphMLNode node : nodeList) {
addNode(graph, parent, node);
}
List edgeList = getEdges();
for (GraphMLEdge edge : edgeList) {
addEdge(graph, parent, edge);
}
}
public boolean hasData(GraphMLNode node) {
boolean ret = false;
if (node.getNodeData() == null) {
ret = false;
} else {
ret = true;
}
return ret;
}
private mxCell addNode(mxGraph graph, Object parent, GraphMLNode node) {
mxCell v1 = null;
String id = node.getNodeId();
GraphMLData data = node.getNodeData();
if (data != null) {
GraphMLGenericNode gNode = null;
Rectangle alterBounds = null;
GraphMLProxyAutoBoundsNode pNode = data.getDataProxyAutoBoundsNode();
if (pNode != null) {
List groupNodes = pNode.getGroupNodes();
gNode = groupNodes.remove(pNode.getActiveRealizer());
if (groupNodes.size() == 1) {
alterBounds = groupNodes.get(0).getGeometry();
}
} else {
gNode = data.getDataGenericNode();
}
if (gNode != null) {
Rectangle geometry = GraphMLUtils.getGeometryFromNode(gNode, (mxCell) parent);
String label = gNode.getNodeLabel();
String style = GraphMLUtils.getStyleFromNode(gNode);
v1 = (mxCell) graph.insertVertex(parent, id, label, geometry.getX(), geometry.getY(), geometry.getWidth(), geometry.getHeight(), style);
if (alterBounds != null) {
v1.getGeometry().setAlternateBounds(new mxRectangle(alterBounds));
}
}
cellsMap.put(id, v1);
GraphMLGraph gmlGraph = node.getNodeGraph();
if (gmlGraph != null) {
gmlGraph.addGraph(graph, v1);
}
}
return v1;
}
private mxCell addEdge(mxGraph graph, Object parent, GraphMLEdge edge) {
mxCell e = null;
String id = edge.getEdgeId();
Object source = cellsMap.get(edge.getEdgeSource());
Object target = cellsMap.get(edge.getEdgeTarget());
GraphMLData data = edge.getEdgeData();
if (data != null) {
GraphMLGenericEdge gEdge = data.getDataGenericEdge();
if (gEdge != null) {
String style = GraphMLUtils.getStyleFromEdge(gEdge);
String label = gEdge.getEdgeLabel();
e = (mxCell) graph.insertEdge(parent, id, label, source, target, style);
mxGeometry geo = e.getGeometry();
geo.setPoints(GraphMLUtils.getControlPointsFromEdge(gEdge, (mxCell) parent));
}
}
return e;
}
public String getEdgedefault() {
return edgedefault;
}
public String getId() {
return id;
}
public List getNodes() {
return nodes;
}
public List getEdges() {
return edges;
}
public boolean isEmpty() {
return nodes.size() == 0 && edges.size() == 0;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy