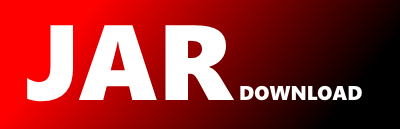
org.yaoqiang.collaboration.ChatRoom Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yaoqiang-bpmn-editor Show documentation
Show all versions of yaoqiang-bpmn-editor Show documentation
an Open Source BPMN 2.0 Modeler
package org.yaoqiang.collaboration;
import java.awt.Dimension;
import java.awt.Toolkit;
import java.awt.event.KeyAdapter;
import java.awt.event.KeyEvent;
import javax.swing.ImageIcon;
import javax.swing.JFrame;
import javax.swing.JScrollPane;
import javax.swing.JSplitPane;
import javax.swing.KeyStroke;
import javax.swing.text.BadLocationException;
import javax.swing.text.Document;
import org.jivesoftware.smack.packet.Message;
/**
* ChatRoom
*
* @author Shi Yaoqiang([email protected])
*/
public class ChatRoom extends JFrame {
private static final long serialVersionUID = -5209416279513025274L;
protected String jid;
protected ChatInputArea chatInputArea;
protected ChatTranscriptArea chatTranscriptArea;
public ChatRoom(String jid) {
this(null, jid);
}
public ChatRoom(String title, String jid) {
this.jid = jid;
// setDefaultCloseOperation(JFrame.DO_NOTHING_ON_CLOSE);
setAlwaysOnTop(true);
setIconImage(new ImageIcon(ContactTreeCellRenderer.class.getResource("/org/yaoqiang/collaboration/images/message.png")).getImage());
if (title == null) {
title = jid;
}
setTitle(title);
setSize(500, 400);
Dimension screenSize = Toolkit.getDefaultToolkit().getScreenSize();
setLocation((screenSize.width - 500) / 2, (screenSize.height - 400) / 2);
creatChatPane();
}
private void creatChatPane() {
chatTranscriptArea = new ChatTranscriptArea();
JScrollPane topPane = new JScrollPane(chatTranscriptArea);
chatInputArea = new ChatInputArea();
chatInputArea.addKeyListener(new KeyAdapter() {
public void keyPressed(KeyEvent e) {
checkForEnter(e);
}
});
JScrollPane bottomPane = new JScrollPane(chatInputArea);
JSplitPane chatPane = new JSplitPane(JSplitPane.VERTICAL_SPLIT, topPane, bottomPane);
chatPane.setBorder(null);
chatPane.setDividerSize(6);
chatPane.setDividerLocation(290);
add(chatPane);
}
private void checkForEnter(KeyEvent e) {
final KeyStroke keyStroke = KeyStroke.getKeyStroke(e.getKeyCode(), e.getModifiers());
if (!keyStroke.equals(KeyStroke.getKeyStroke(KeyEvent.VK_ENTER, KeyEvent.SHIFT_DOWN_MASK)) && e.getKeyChar() == KeyEvent.VK_ENTER) {
e.consume();
sendMessage();
chatInputArea.setText("");
chatInputArea.setCaretPosition(0);
} else if (keyStroke.equals(KeyStroke.getKeyStroke(KeyEvent.VK_ENTER, KeyEvent.SHIFT_DOWN_MASK))) {
final Document document = chatInputArea.getDocument();
try {
document.insertString(chatInputArea.getCaretPosition(), "\n", null);
chatInputArea.requestFocusInWindow();
} catch (BadLocationException badLoc) {
}
}
}
public void sendMessage() {
Message message = new Message(jid, Message.Type.chat);
message.setBody(chatInputArea.getText());
MainPanel.getConnection().sendPacket(message);
chatTranscriptArea.insertMessage(message);
}
public void insertMessage(Message message) {
chatTranscriptArea.insertMessage(message);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy