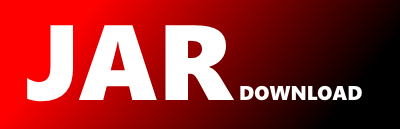
org.yaoqiang.graph.shape.DataObjectShape Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yaoqiang-bpmn-editor Show documentation
Show all versions of yaoqiang-bpmn-editor Show documentation
an Open Source BPMN 2.0 Modeler
package org.yaoqiang.graph.shape;
import java.awt.Polygon;
import java.awt.Rectangle;
import java.awt.Shape;
import org.yaoqiang.bpmn.model.elements.data.DataInput;
import org.yaoqiang.bpmn.model.elements.data.DataObject;
import org.yaoqiang.bpmn.model.elements.data.DataOutput;
import org.yaoqiang.bpmn.model.elements.data.ItemAwareElement;
import org.yaoqiang.graph.model.GraphModel;
import org.yaoqiang.graph.util.Constants;
import com.mxgraph.canvas.mxGraphics2DCanvas;
import com.mxgraph.model.mxICell;
import com.mxgraph.shape.mxBasicShape;
import com.mxgraph.view.mxCellState;
/**
* DataObjectShape
*
* @author Shi Yaoqiang([email protected])
*/
public class DataObjectShape extends mxBasicShape {
public void paintShape(mxGraphics2DCanvas canvas, mxCellState state) {
super.paintShape(canvas, state);
ItemAwareElement dataObject = null;
Object value = ((mxICell)state.getCell()).getValue();
if (value instanceof String) {
dataObject = new DataObject((String) value, false);
} else if (value instanceof ItemAwareElement) {
dataObject = (ItemAwareElement) value;
}
GraphModel model = (GraphModel) state.getView().getGraph().getModel();
double scale = canvas.getScale();
double imgWidth = 16 * scale;
double imgHeight = 16 * scale;
Rectangle imageBounds = state.getRectangle();
imageBounds.setRect(imageBounds.getX() + (imageBounds.getWidth() - imgWidth) / 4, imageBounds.getY(), imgWidth, imgHeight);
String image = null;
if (dataObject instanceof DataInput) {
image = Constants.SHAPE_MARKER + "event_link.png";
} else if (dataObject instanceof DataOutput) {
image = Constants.SHAPE_MARKER + "event_link_throwing.png";
}
canvas.drawImage(imageBounds, image);
if (model.isCollectionDataObject(state.getCell())) {
Rectangle markerImageBounds = state.getRectangle();
markerImageBounds.setRect(markerImageBounds.getX() + (markerImageBounds.getWidth() - imgWidth) / 2,
markerImageBounds.getY() + markerImageBounds.getHeight() - imgHeight, imgWidth, imgHeight);
canvas.drawImage(markerImageBounds, Constants.SHAPE_MARKER + "loop_multiple.png");
}
Rectangle rec = state.getRectangle();
int x = rec.x;
int y = rec.y;
int w = rec.width;
int a = rec.width / 3;
canvas.getGraphics().drawLine(x + w - a, y, x + w - a, y + a);
canvas.getGraphics().drawLine(x + w - a, y + a, x + w, y + a);
}
public Shape createShape(mxGraphics2DCanvas canvas, mxCellState state) {
Rectangle rec = state.getRectangle();
int x = rec.x;
int y = rec.y;
int w = rec.width;
int h = rec.height;
int a = rec.width / 3;
Polygon dataObject = new Polygon();
dataObject.addPoint(x, y);
dataObject.addPoint(x, y + h);
dataObject.addPoint(x + w, y + h);
dataObject.addPoint(x + w, y + a);
dataObject.addPoint(x + w - a, y);
return dataObject;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy