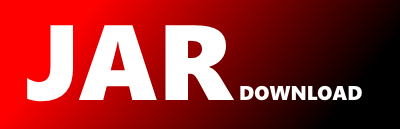
jadex.bdi.planlib.DefaultBDIViewerPanel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jadex-applib-bdi Show documentation
Show all versions of jadex-applib-bdi Show documentation
The Jadex applib BDI package contain
ready to use functionalities for
BDI agents mostly in form of modules
called capabilities.
package jadex.bdi.planlib;
import jadex.base.gui.componentviewer.AbstractComponentViewerPanel;
import jadex.base.gui.componentviewer.IAbstractViewerPanel;
import jadex.base.gui.componentviewer.IComponentViewerPanel;
import jadex.base.gui.plugin.IControlCenter;
import jadex.bdi.runtime.IBDIInternalAccess;
import jadex.bdi.runtime.ICapability;
import jadex.bridge.IComponentStep;
import jadex.bridge.IExternalAccess;
import jadex.bridge.IInternalAccess;
import jadex.commons.SReflect;
import jadex.commons.future.CounterResultListener;
import jadex.commons.future.DelegationResultListener;
import jadex.commons.future.ExceptionDelegationResultListener;
import jadex.commons.future.Future;
import jadex.commons.future.IFuture;
import jadex.commons.transformation.annotations.Classname;
import java.awt.BorderLayout;
import java.util.ArrayList;
import java.util.List;
import javax.swing.JComponent;
import javax.swing.JPanel;
import javax.swing.JTabbedPane;
/**
* Default panel for viewing BDI agents that include viewable capabilities.
*/
public class DefaultBDIViewerPanel extends AbstractComponentViewerPanel
{
//-------- constants --------
/** The constant for the agent optional viewerclass. */
public static final String PROPERTY_AGENTVIEWERCLASS = "bdiviewerpanel.agentviewerclass";
/** The constant for the agent optional viewerclass. */
public static final String PROPERTY_INCLUDESUBCAPABILITIES = "bdiviewerpanel.includesubcapabilities";
//-------- attributes --------
/** The panel. */
protected JPanel panel;
/** The classloader for the component. */
protected ClassLoader cl;
//-------- methods --------
/**
* Called once to initialize the panel.
* Called on the swing thread.
* @param jcc The jcc.
* @param component The component.
*/
public IFuture init(final IControlCenter jcc, final IExternalAccess component)
{
final Future ret = new Future();
this.panel = new JPanel(new BorderLayout());
// Init interface is asynchronous but super implementation is not.
IFuture fut = super.init(jcc, component);
assert fut.isDone();
jcc.getClassLoader(component.getModel().getResourceIdentifier())
.addResultListener(new ExceptionDelegationResultListener(ret)
{
public void customResultAvailable(ClassLoader result)
{
cl = result;
component.scheduleStep(new IComponentStep()
{
@Classname("createPanels")
public IFuture execute(IInternalAccess ia)
{
final IBDIInternalAccess scope = (IBDIInternalAccess)ia;
String[] subcapnames = (String[])scope.getModel().getProperty(PROPERTY_INCLUDESUBCAPABILITIES, cl);
if(subcapnames==null)
{
subcapnames = (String[])scope.getSubcapabilityNames();
}
createPanels(scope, subcapnames, ret);
return IFuture.DONE;
}
}).addResultListener(new DelegationResultListener(ret));
}
});
return ret;
}
/**
* Create the panels.
*/
protected void createPanels(IBDIInternalAccess scope, String[] subcapnames, Future ret)
{
final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy