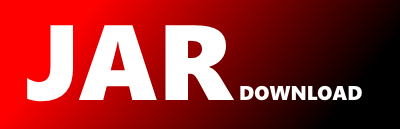
jadex.bdi.examples.blackjack.player.PlayerSearchDealerPlan Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jadex-applications-bdi Show documentation
Show all versions of jadex-applications-bdi Show documentation
The Jadex BDI applications package contain
several example applications, benchmarks and
testcases using BDI agents.
The newest version!
package jadex.bdi.examples.blackjack.player;
import jadex.bdi.runtime.IGoal;
import jadex.bdi.runtime.Plan;
import jadex.bridge.IComponentIdentifier;
import jadex.bridge.ISearchConstraints;
import jadex.bridge.service.RequiredServiceInfo;
import jadex.bridge.service.search.SServiceProvider;
import jadex.bridge.service.types.df.IDF;
import jadex.bridge.service.types.df.IDFComponentDescription;
import jadex.bridge.service.types.df.IDFServiceDescription;
import java.util.Random;
/**
*
*/
public class PlayerSearchDealerPlan extends Plan
{
//-------- methods --------
/**
* First the player searches a dealer, then sends a join-request to this
* dealer.
*/
public void body()
{
//System.out.println("Searching dealer...");
// Create a service description to search for.
IDF df = (IDF)SServiceProvider.getService(getServiceContainer(), IDF.class, RequiredServiceInfo.SCOPE_PLATFORM).get(this);
IDFServiceDescription sd = df.createDFServiceDescription(null, "blackjack", null);
IDFComponentDescription ad = df.createDFComponentDescription(null, sd);
ISearchConstraints sc = df.createSearchConstraints(-1, 0);
// Use a subgoal to search for a dealer-agent
IGoal ft = createGoal("df_search");
ft.getParameter("description").setValue(ad);
ft.getParameter("constraints").setValue(sc);
dispatchSubgoalAndWait(ft);
IDFComponentDescription[] result = (IDFComponentDescription[])ft.getParameterSet("result").getValues();
if(result==null || result.length==0)
{
getLogger().warning("No blackjack-dealer found.");
fail();
}
else
{
// at least one matching description found,
getLogger().info(result.length + " blackjack-dealer found");
// choose one dealer randomly out of all the dealer-agents
IComponentIdentifier dealer = result[new Random().nextInt(result.length)].getName();
getBeliefbase().getBelief("dealer").setFact(dealer);
}
}
/**
* Called when something went wrong (e.g. timeout).
*/
public void failed()
{
// Remove dealer fact.
getBeliefbase().getBelief("dealer").setFact(null);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy