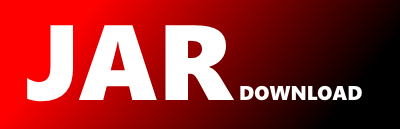
jadex.bdi.examples.cleanerworld_classic.Environment Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jadex-applications-bdi Show documentation
Show all versions of jadex-applications-bdi Show documentation
The Jadex BDI applications package contain
several example applications, benchmarks and
testcases using BDI agents.
The newest version!
package jadex.bdi.examples.cleanerworld_classic;
import jadex.commons.SimplePropertyChangeSupport;
import jadex.commons.beans.PropertyChangeListener;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* The environment object for non distributed applications.
*/
public class Environment implements IEnvironment
{
//-------- class attributes --------
/** The singleton. */
protected static Environment instance;
//-------- attributes --------
/** The daytime. */
protected boolean daytime;
/** The cleaners. */
protected List cleaners;
/** The wastes. */
protected List wastes;
/** The waste bins. */
protected List wastebins;
/** The charging stations. */
protected List stations;
/** The cleaner ages. */
protected Map ages;
/** The helper object for bean events. */
public SimplePropertyChangeSupport pcs;
//-------- constructors --------
/**
* Create a new environment.
*/
public Environment()
{
this.daytime = true;
this.cleaners = new ArrayList();
this.wastes = new ArrayList();
this.wastebins = new ArrayList();
this.stations = new ArrayList();
this.ages = new HashMap();
this.pcs = new SimplePropertyChangeSupport(this);
// Add some things to our world.
addWaste(new Waste(new Location(0.1, 0.5)));
addWaste(new Waste(new Location(0.2, 0.5)));
addWaste(new Waste(new Location(0.3, 0.5)));
addWaste(new Waste(new Location(0.9, 0.9)));
addWastebin(new Wastebin(new Location(0.2, 0.2), 20));
addWastebin(new Wastebin(new Location(0.8, 0.1), 20));
addChargingStation(new Chargingstation(new Location(0.8, 0.8)));
addChargingStation(new Chargingstation(new Location(0.2, 0.4)));
}
/**
* Get the singleton.
* @return The environment.
*/
public static synchronized Environment getInstance()
{
if(instance==null)
{
instance = new Environment();
}
return instance;
}
/**
* Clear the singleton instance.
*/
public static void clearInstance()
{
instance = null;
}
//-------- cleaner actions from IEnvironment --------
/**
* Get the current vision.
* @param cleaner The cleaner.
* @return The current vision, null if failure.
*/
public synchronized Vision getVision(Cleaner cleaner)
{
// Update cleaner
if(cleaners.contains(cleaner))
cleaners.remove(cleaner);
cleaners.add(cleaner);
// reset age for cleaner
ages.put(cleaner, Integer.valueOf(0));
//System.out.println("getVision: "+this.hashCode()+" "+cleaners.size());
Location cloc = cleaner.getLocation();
double range = cleaner.getVisionRange();
ArrayList nearwastes = new ArrayList();
for(int i=0; i100)
{
removeCleaner(cls[i]);
ages.remove(cls[i]);
}
else
{
ages.put(cls[i], Integer.valueOf(age+1));
}
}
}
return (Cleaner[])cleaners.toArray(new Cleaner[cleaners.size()]);
}
/**
* Get a wastebin for a name.
* @return The wastebin.
*/
public synchronized Wastebin getWastebin(String name)
{
Wastebin ret = null;
for(int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy