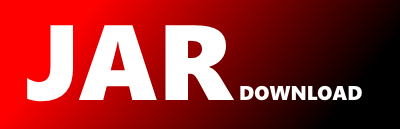
jadex.bdi.examples.hunterprey_classic.environment.MapPanel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jadex-applications-bdi Show documentation
Show all versions of jadex-applications-bdi Show documentation
The Jadex BDI applications package contain
several example applications, benchmarks and
testcases using BDI agents.
The newest version!
package jadex.bdi.examples.hunterprey_classic.environment;
import jadex.bdi.examples.hunterprey_classic.Creature;
import jadex.bdi.examples.hunterprey_classic.CurrentVision;
import jadex.bdi.examples.hunterprey_classic.Food;
import jadex.bdi.examples.hunterprey_classic.Hunter;
import jadex.bdi.examples.hunterprey_classic.Location;
import jadex.bdi.examples.hunterprey_classic.Observer;
import jadex.bdi.examples.hunterprey_classic.Obstacle;
import jadex.bdi.examples.hunterprey_classic.Prey;
import jadex.bdi.examples.hunterprey_classic.WorldObject;
import jadex.commons.gui.SGUI;
import java.awt.Color;
import java.awt.Component;
import java.awt.Dimension;
import java.awt.Frame;
import java.awt.Graphics;
import java.awt.Image;
import java.awt.Rectangle;
import java.awt.event.ComponentAdapter;
import java.awt.event.ComponentEvent;
import java.awt.event.MouseEvent;
import javax.swing.ImageIcon;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.ToolTipManager;
import javax.swing.UIDefaults;
/**
* The map for the hunter prey world.
*/
public class MapPanel extends JPanel
{
//-------- constants --------
/** The image icons. */
public static final UIDefaults icons = new UIDefaults(new Object[]
{
"food", SGUI.makeIcon(MapPanel.class, "/jadex/bdi/examples/hunterprey_classic/images/food.png"),
"obstacle", SGUI.makeIcon(MapPanel.class, "/jadex/bdi/examples/hunterprey_classic/images/obstacle.png"),
"hunter", SGUI.makeIcon(MapPanel.class, "/jadex/bdi/examples/hunterprey_classic/images/hunter.png"),
"prey", SGUI.makeIcon(MapPanel.class, "/jadex/bdi/examples/hunterprey_classic/images/prey.png"),
"observer", SGUI.makeIcon(MapPanel.class, "/jadex/bdi/examples/hunterprey_classic/images/observer.png"),
"background", SGUI.makeIcon(MapPanel.class, "/jadex/bdi/examples/hunterprey_classic/images/background.png")
});
//-------- attributes --------
/** The vision to display in the panel. */
protected CurrentVision cv;
/** The component to display obstacles. */
protected JLabel obstacle;
/** The component to display food. */
protected JLabel food;
/** The component to display prey. */
protected JLabel prey;
/** The component to display hunters. */
protected JLabel hunter;
/** The background image. */
protected Image background_image;
/** The obstacle image. */
protected Image obstacle_image;
/** The food image. */
protected Image food_image;
/** The prey image. */
protected Image prey_image;
/** The hunter image. */
protected Image hunter_image;
/** Flag to indicate that component has changed and sizes have to be recalculated. */
protected boolean rescale;
//-------- constructors --------
/**
* Create a new map panel.
*/
public MapPanel()
{
// Create icon images for objects in the world.
this.background_image = ((ImageIcon)icons.getIcon("background")).getImage();
this.food_image = ((ImageIcon)icons.getIcon("food")).getImage();
this.obstacle_image = ((ImageIcon)icons.getIcon("obstacle")).getImage();
this.hunter_image = ((ImageIcon)icons.getIcon("hunter")).getImage();
this.prey_image = ((ImageIcon)icons.getIcon("prey")).getImage();
// Create components for objects in the world.
this.obstacle = new JLabel(new ImageIcon(obstacle_image), JLabel.CENTER);
this.food = new JLabel(new ImageIcon(food_image), JLabel.CENTER);
this.hunter = new JLabel(new ImageIcon(hunter_image), JLabel.CENTER);
hunter.setVerticalTextPosition(JLabel.BOTTOM);
hunter.setHorizontalTextPosition(JLabel.CENTER);
this.prey = new JLabel(new ImageIcon(prey_image), JLabel.CENTER);
prey.setVerticalTextPosition(JLabel.BOTTOM);
prey.setHorizontalTextPosition(JLabel.CENTER);
// Trigger rescaling of images.
this.addComponentListener(new ComponentAdapter()
{
public void componentResized(ComponentEvent ce)
{
rescale = true;
}
});
// Activate tooltips.
ToolTipManager.sharedInstance().registerComponent(this);
}
//-------- methods --------
/**
* Update the map with a new current vision.
* @param cv The new current vision to display.
*/
public void update(CurrentVision cv)
{
this.cv = cv;
//this.invalidate();
this.repaint();
}
//-------- paint methods --------
// overridden paint method.
protected void paintComponent(Graphics g)
{
if(cv==null)
{
g.setColor(Color.BLACK);
g.drawString("No vision!", getBounds().width/2, getBounds().height/2);
return;
}
int worldwidth = cv.getCreature().getWorldWidth();
int worldheight = cv.getCreature().getWorldHeight();
Rectangle bounds = getBounds();
double cellw = bounds.getWidth()/(double)worldwidth;
double cellh = bounds.getHeight()/(double)worldheight;
// Rescale images if necessary.
if(rescale)
{
((ImageIcon)obstacle.getIcon()).setImage(
obstacle_image.getScaledInstance((int)cellw, (int)cellh, Image.SCALE_DEFAULT));
((ImageIcon)food.getIcon()).setImage(
food_image.getScaledInstance((int)cellw, (int)cellh, Image.SCALE_DEFAULT));
((ImageIcon)hunter.getIcon()).setImage(
hunter_image.getScaledInstance((int)cellw, (int)cellh,Image.SCALE_DEFAULT));
((ImageIcon)prey.getIcon()).setImage(
prey_image.getScaledInstance((int)cellw, (int)cellh, Image.SCALE_DEFAULT));
rescale = false;
}
// Paint background.
Image image = background_image;
int w = image.getWidth(this);
int h = image.getHeight(this);
if(w>0 && h>0)
{
for(int y=0; y=worldwidth-vision)
{
for(int j=1; j<=vision; j++)
{
g.fillRect((int)(cellw*(loc.getX()-j-worldwidth)), (int)(cellh*(loc.getY()-j)), (int)(cellw*(j*2+1)), (int)(cellh*(j*2+1)));
}
}
if(loc.getY()=worldheight-vision)
{
for(int j=1; j<=vision; j++)
{
g.fillRect((int)(cellw*(loc.getX()-j-worldheight)), (int)(cellh*(loc.getY()-j)), (int)(cellw*(j*2+1)), (int)(cellh*(j*2+1)));
}
}
}
}
// Paint creatures.
for(int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy