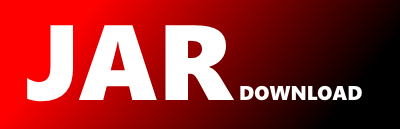
jadex.bridge.service.IServiceContainer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jadex-bridge Show documentation
Show all versions of jadex-bridge Show documentation
Jadex bridge is a base package for kernels and platforms,
i.e. it is used by both and provides commonly used
interfaces and classes for active components and
their management.
The newest version!
package jadex.bridge.service;
import jadex.bridge.IComponentIdentifier;
import jadex.bridge.nonfunctional.INFMixedPropertyProvider;
import jadex.bridge.sensor.service.IMethodInvocationListener;
import jadex.bridge.service.component.IServiceInvocationInterceptor;
import jadex.bridge.service.component.ServiceInvocationContext;
import jadex.commons.IRemoteFilter;
import jadex.commons.IResultCommand;
import jadex.commons.MethodInfo;
import jadex.commons.future.IFuture;
import jadex.commons.future.IIntermediateFuture;
import jadex.commons.future.ITerminableIntermediateFuture;
import java.lang.reflect.Method;
import java.util.Collection;
/**
* Internal interface for a service container. Allows
* adding and removing services.
*/
// todo: remove IServiceProvider interface
// move search method from IServiceProvider to IExternalAccess
// use external access for searching
// make this interface the public interface for internal usage of provider
public interface IServiceContainer extends IServiceProvider
{
//-------- internal admin methods --------
/**
* Start the service.
* @return A future that is done when the service has completed starting.
*/
// todo: remove, only call from platform
public IFuture start();
/**
* Shutdown the service.
* @return A future that is done when the service has completed its shutdown.
*/
// todo: remove, only call from platform
public IFuture shutdown();
/**
* Add a service to the container.
* The service is started, if the container is already running.
* @param service The service.
* @param info The provided service info.
* @return A future that is done when the service has completed starting.
*/
public IFuture addService(IInternalService service, ProvidedServiceInfo info);
/**
* Removes a service from the container (shutdowns also the service if the container is running).
* @param service The service identifier.
* @return A future that is done when the service has completed its shutdown.
*/
public IFuture removeService(IServiceIdentifier sid);
/**
* Get provided (declared) service.
* @param name The service name.
* @return The service.
*/
public IService getProvidedService(String name);
/**
* Get provided (declared) service.
* @param clazz The interface.
* @return The service.
*/
public IService getProvidedService(Class> clazz);
/**
* Get provided (declared) service.
* @param clazz The interface.
* @return The service.
*/
public IService[] getProvidedServices(Class> clazz);
/**
* Get the provided service
*/
public Object getProvidedServiceRawImpl(Class> clazz);
/**
* Get the required service infos.
*/
public RequiredServiceInfo[] getRequiredServiceInfos();
/**
* Get the required service info.
* @param name The name.
* @return The required service info.
*/
public RequiredServiceInfo getRequiredServiceInfo(String name);
/**
* Set the required services.
* @param required services The required services to set.
*/
public void setRequiredServiceInfos(RequiredServiceInfo[] requiredservices);
/**
* Add required services for a given prefix.
* @param prefix The name prefix to use.
* @param required services The required services to set.
*/
public void addRequiredServiceInfos(RequiredServiceInfo[] requiredservices);
/**
* Get the required service property provider for a service.
*/
public INFMixedPropertyProvider getRequiredServicePropertyProvider(IServiceIdentifier sid);
/**
* Has the service a property provider.
*/
public boolean hasRequiredServicePropertyProvider(IServiceIdentifier sid);
/**
* Get a required service of a given name.
* @param name The service name.
* @return The service.
*/
public IFuture getRequiredService(String name);
/**
* Get a required services of a given name.
* @param name The services name.
* @return Each service as an intermediate result and a collection of services as final result.
*/
public ITerminableIntermediateFuture getRequiredServices(String name);
/**
* Get a required service.
* @return The service.
*/
public IFuture getRequiredService(String name, boolean rebind);
/**
* Get a required services.
* @return Each service as an intermediate result and a collection of services as final result.
*/
public ITerminableIntermediateFuture getRequiredServices(String name, boolean rebind);
/**
* Get a required service.
* @return The service.
*/
public IFuture getRequiredService(String name, boolean rebind, IRemoteFilter filter);
/**
* Get a required services.
* @return Each service as an intermediate result and a collection of services as final result.
*/
public ITerminableIntermediateFuture getRequiredServices(String name, boolean rebind, IRemoteFilter filter);
/**
* Get the result of the last search.
* @param name The required service name.
* @return The last result.
*/
public T getLastRequiredService(String name);
/**
* Get the result of the last search.
* @param name The required services name.
* @return The last result.
*/
public Collection getLastRequiredServices(String name);
/**
* Add a service interceptor.
* @param interceptor The interceptor.
* @param service The service.
* @param pos The position (0=first).
*/
public void addInterceptor(IServiceInvocationInterceptor interceptor, Object service, int pos);
/**
* Remove a service interceptor.
* @param interceptor The interceptor.
* @param service The service.
*/
public void removeInterceptor(IServiceInvocationInterceptor interceptor, Object service);
/**
* Get the interceptors of a service.
* @param service The service.
* @return The interceptors.
*/
public IServiceInvocationInterceptor[] getInterceptors(Object service);
/**
* Add a method invocation handler.
*/
public void addMethodInvocationListener(IServiceIdentifier sid, MethodInfo mi, IMethodInvocationListener listener);
/**
* Remove a method invocation handler.
*/
public void removeMethodInvocationListener(IServiceIdentifier sid, MethodInfo mi, IMethodInvocationListener listener);
/**
* Notify listeners that a service method has been called.
*/
public void notifyMethodListeners(IServiceIdentifier sid, boolean start, Object proxy, final Method method, final Object[] args, Object callid, ServiceInvocationContext context);
/**
* Test if service and method has listeners.
*/
public boolean hasMethodListeners(IServiceIdentifier sid, MethodInfo mi);
/**
* Get one service of a type from a specific component.
* @param type The class.
* @param cid The component identifier of the target component.
* @return The corresponding service.
*/
public IFuture getService(Class type, IComponentIdentifier cid);
/**
* Get one service of a type.
* @param type The class.
* @return The corresponding service.
*/
public IFuture searchService(Class type);
/**
* Get one service of a type.
* @param type The class.
* @return The corresponding service.
*/
public IFuture searchService(Class type, String scope);
// todo: remove! is just convenience for search(type, upwards)
/**
* Get one service of a type and only search upwards (parents).
* @param type The class.
* @return The corresponding service.
*/
public IFuture searchServiceUpwards(Class type);
/**
* Get all services of a type.
* @param type The class.
* @return Each service as an intermediate result and a collection of services as final result.
*/
public IIntermediateFuture searchServices(Class type);
/**
* Get all services of a type.
* @param type The class.
* @return Each service as an intermediate result and a collection of services as final result.
*/
public IIntermediateFuture searchServices(Class type, String scope);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy