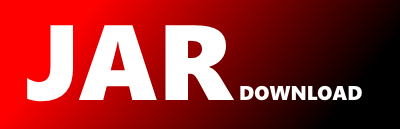
jadex.extension.ws.invoke.WebServiceWrapperInvocationHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jadex-platform-extension-webservice Show documentation
Show all versions of jadex-platform-extension-webservice Show documentation
The Jadex webservice platform extension package contains
basic functionality for WSDL web services and REST web services.
The newest version!
package jadex.extension.ws.invoke;
import jadex.bridge.IComponentIdentifier;
import jadex.bridge.IComponentStep;
import jadex.bridge.IExternalAccess;
import jadex.bridge.IInternalAccess;
import jadex.bridge.service.RequiredServiceInfo;
import jadex.bridge.service.annotation.Service;
import jadex.bridge.service.search.SServiceProvider;
import jadex.bridge.service.types.cms.CreationInfo;
import jadex.bridge.service.types.cms.IComponentManagementService;
import jadex.commons.future.DelegationResultListener;
import jadex.commons.future.ExceptionDelegationResultListener;
import jadex.commons.future.Future;
import jadex.commons.future.IFuture;
import java.lang.reflect.InvocationHandler;
import java.lang.reflect.Method;
/**
* Create a new web service wrapper invocation handler.
*
* Creates an 'web service invocation agent' for each method invocation.
* Lets this invocation agent call the web service by using the mapping
* data to determine details about the service call.
* The invocation agent returns the result and terminates itself after the call.
*/
@Service
class WebServiceWrapperInvocationHandler implements InvocationHandler
{
//-------- attributes --------
/** The agent. */
protected IInternalAccess agent;
/** The web service. */
protected WebServiceMappingInfo mapping;
//-------- constructors --------
/**
* Create a new service wrapper invocation handler.
* @param agent The internal access of the agent.
* @mapping The mapping info about the web service to Java.
*/
public WebServiceWrapperInvocationHandler(IInternalAccess agent, WebServiceMappingInfo mapping)
{
if(agent==null)
throw new IllegalArgumentException("Agent must not null.");
if(mapping==null)
throw new IllegalArgumentException("Web service mapping must not null.");
this.agent = agent;
this.mapping = mapping;
}
//-------- methods --------
/**
* Called when a wrapper method is invoked.
* Uses the cms to create a new invocation agent and lets this
* agent call the web service. The result is transferred back
* into the result future of the caller.
*/
public Object invoke(Object proxy, final Method method, final Object[] args) throws Throwable
{
final Future
© 2015 - 2025 Weber Informatics LLC | Privacy Policy