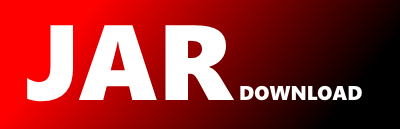
jadex.rules.examples.hanoi.Hanoi Maven / Gradle / Ivy
package jadex.rules.examples.hanoi;
import jadex.commons.gui.SGUI;
import jadex.rules.rulesystem.IAction;
import jadex.rules.rulesystem.ICondition;
import jadex.rules.rulesystem.IRule;
import jadex.rules.rulesystem.IVariableAssignments;
import jadex.rules.rulesystem.RuleSystem;
import jadex.rules.rulesystem.RuleSystemExecutor;
import jadex.rules.rulesystem.Rulebase;
import jadex.rules.rulesystem.rete.RetePatternMatcherFunctionality;
import jadex.rules.rulesystem.rules.AndCondition;
import jadex.rules.rulesystem.rules.BoundConstraint;
import jadex.rules.rulesystem.rules.IOperator;
import jadex.rules.rulesystem.rules.LiteralConstraint;
import jadex.rules.rulesystem.rules.NotCondition;
import jadex.rules.rulesystem.rules.ObjectCondition;
import jadex.rules.rulesystem.rules.Rule;
import jadex.rules.rulesystem.rules.Variable;
import jadex.rules.state.IOAVState;
import jadex.rules.state.IOAVStateListener;
import jadex.rules.state.OAVAttributeType;
import jadex.rules.state.OAVJavaType;
import jadex.rules.state.OAVObjectType;
import jadex.rules.state.OAVTypeModel;
import jadex.rules.state.javaimpl.OAVStateFactory;
import jadex.rules.tools.reteviewer.RuleEnginePanel;
import java.awt.Graphics;
import java.awt.Insets;
import java.awt.Rectangle;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
import java.util.ArrayList;
import java.util.List;
import javax.swing.JComponent;
import javax.swing.JFrame;
/**
* OAV test doing towers of Hanoi.
*/
public class Hanoi
{
//-------- OAV metamodel --------
/** The type model. */
public static final OAVTypeModel hanoi_type_model;
/** The java OAV atribute type. */
public static final OAVObjectType java_oavattribute_type;
/** The agent type. */
public static final OAVObjectType agent_type;
/** Agent has tower A. */
public static final OAVAttributeType agent_has_tower_a;
/** Agent has tower B. */
public static final OAVAttributeType agent_has_tower_b;
/** Agent has tower C. */
public static final OAVAttributeType agent_has_tower_c;
/** Agent has move goals. */
public static final OAVAttributeType agent_has_movegoals;
/** The disc type. */
public static final OAVObjectType disc_type;
/** Disc has size. */
public static final OAVAttributeType disc_has_size;
/** The move goal type. */
public static final OAVObjectType movegoal_type;
/** Move goal is executing. */
public static final OAVAttributeType movegoal_is_executing;
/** Move goal has precondition. */
public static final OAVAttributeType movegoal_has_precodition;
/** Move goal has postcondition. */
public static final OAVAttributeType movegoal_has_postcodition;
/** Move goal has from. */
public static final OAVAttributeType movegoal_has_from ;
/** Move goal has to. */
public static final OAVAttributeType movegoal_has_to;
/** Move goal has temp. */
public static final OAVAttributeType movegoal_has_temp;
/** Move goal has number. */
public static final OAVAttributeType movegoal_has_number;
static
{
hanoi_type_model = new OAVTypeModel("hanoi_type_model");
hanoi_type_model.addTypeModel(OAVJavaType.java_type_model);
java_oavattribute_type = hanoi_type_model.createJavaType(OAVAttributeType.class, OAVJavaType.KIND_VALUE);
disc_type = hanoi_type_model.createType("disc");
disc_has_size = disc_type.createAttributeType("disc_has_size", OAVJavaType.java_integer_type);
movegoal_type = hanoi_type_model.createType("movegoal");
movegoal_is_executing = movegoal_type.createAttributeType("movegoal_is_executing", OAVJavaType.java_boolean_type, OAVAttributeType.NONE, Boolean.FALSE);
movegoal_has_precodition = movegoal_type.createAttributeType("movegoal_has_precondition", movegoal_type);
movegoal_has_postcodition = movegoal_type.createAttributeType("movegoal_has_postcondition", movegoal_type);
movegoal_has_from = movegoal_type.createAttributeType("movegoal_has_from", java_oavattribute_type);
movegoal_has_to = movegoal_type.createAttributeType("movegoal_has_to", java_oavattribute_type);
movegoal_has_temp = movegoal_type.createAttributeType("movegoal_has_temp", java_oavattribute_type);
movegoal_has_number = movegoal_type.createAttributeType("movegoal_has_number", OAVJavaType.java_integer_type);
agent_type = hanoi_type_model.createType("agent");
agent_has_tower_a = agent_type.createAttributeType("agent_has_tower_a", disc_type, OAVAttributeType.LIST);
agent_has_tower_b = agent_type.createAttributeType("agent_has_tower_b", disc_type, OAVAttributeType.LIST);
agent_has_tower_c = agent_type.createAttributeType("agent_has_tower_c", disc_type, OAVAttributeType.LIST);
agent_has_movegoals = agent_type.createAttributeType("agent_has_movegoals", movegoal_type, OAVAttributeType.SET);
}
//-------- main --------
/**
* Main for testing.
*/
public static void main(String[] args)
{
int discs = 15;
// Implementation to use: 1=Rete, 2=Goals, 3=State, 4=Lists
int impl = 1;
boolean show_towers = true;
boolean show_rete = true;
test(discs, impl, show_towers, show_rete);
// Run benchmark comparing all implementations.
// benchmark(discs);
}
/**
* Test method. Calls one of the implementations once.
*/
protected static void test(int discs, int impl, boolean showtowers, boolean showrete)
{
switch(impl)
{
// Rete implementation.
case 1:
{
IOAVState state = createState();
RuleSystem rete = initializeRete(state, showrete);
Object agent = initState(discs, state, showtowers);
moveWithRete(state, agent, agent_has_tower_a, agent_has_tower_c, agent_has_tower_b, discs, rete, showrete);
break;
}
// State with goals but without Rete.
case 2:
{
IOAVState state = createState();
Object agent = initState(discs, state, showtowers);
moveWithoutRete(state, agent, agent_has_tower_a, agent_has_tower_c, agent_has_tower_b, discs);
break;
}
// Simple state based implementation
case 3:
{
IOAVState state = createState();
Object agent = initState(discs, state, showtowers);
moveWithState(state, agent, agent_has_tower_a, agent_has_tower_c, agent_has_tower_b, discs);
break;
}
// Simple list based implementation (no state involved).
case 4:
{
IOAVState state = createState();
Object agent = initState(discs, state, showtowers);
List from = new ArrayList();
List to = new ArrayList();
List temp = new ArrayList();
from.addAll(state.getAttributeValues(agent, agent_has_tower_a));
moveWithoutState(from, to, temp, discs);
break;
}
}
}
/**
* Create an OAV state.
*/
protected static IOAVState createState()
{
return OAVStateFactory.createOAVState(hanoi_type_model);
// return new JenaOAVState();
}
/**
* Initialize the state with the given number of discs.
*/
protected static Object initState(int discs, IOAVState state, boolean showtowers)
{
// Setup
Object agent = state.createRootObject(agent_type);
for(int i=discs; i>0; i--)
{
Object disc = state.createObject(disc_type);
state.setAttributeValue(disc, disc_has_size, Integer.valueOf(i));
state.addAttributeValue(agent, agent_has_tower_a, disc);
}
// Show Hanoi towers in JFrame.
if(showtowers)
showFrame(state, agent);
return agent;
}
/**
* Main for testing.
*/
public static void benchmark(int maxdiscs)
{
int times = (int)Math.pow(2, maxdiscs);
int discs = 1;
while(times>1)
{
// Simple list based implementation (no state involved).
IOAVState state = createState();
Object agent = initState(discs, state, false);
List from = new ArrayList();
List to = new ArrayList();
List temp = new ArrayList();
from.addAll(state.getAttributeValues(agent, agent_has_tower_a));
long nostatestart = System.currentTimeMillis();
for(int i=0; i<(times*3); i++)
{
if(i%2==0)
{
moveWithoutState(from, to, temp, discs);
}
else
{
moveWithoutState(to, from, temp, discs);
}
}
double nostatetime = calcTime(nostatestart, times*3, discs);
// Simple state based implementation
state = createState();
agent = initState(discs, state, false);
long statestart = System.currentTimeMillis();
for(int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy