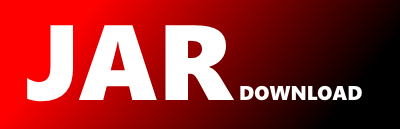
jadex.rules.examples.manners.Manners Maven / Gradle / Ivy
package jadex.rules.examples.manners;
import jadex.rules.rulesystem.LIFOAgenda;
import jadex.rules.rulesystem.RuleSystem;
import jadex.rules.rulesystem.RuleSystemExecutor;
import jadex.rules.rulesystem.Rulebase;
import jadex.rules.rulesystem.rete.RetePatternMatcherFunctionality;
import jadex.rules.state.IOAVState;
import jadex.rules.state.OAVAttributeType;
import jadex.rules.state.OAVJavaType;
import jadex.rules.state.OAVObjectType;
import jadex.rules.state.OAVTypeModel;
import jadex.rules.state.javaimpl.OAVStateFactory;
import jadex.rules.tools.reteviewer.RuleEnginePanel;
import java.io.File;
import java.io.FileReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.LineNumberReader;
/**
* The manners benchmark.
*/
public class Manners
{
protected static boolean print;
//-------- type definitions --------
/** The manners type definition. */
public static final OAVTypeModel manners_type_model;
/** The guest type. */
public static final OAVObjectType guest_type;
/** A guest has a name. */
public static final OAVAttributeType guest_has_name;
/** A guest has a sex. */
public static final OAVAttributeType guest_has_sex;
/** A guest has hobbies. */
//public static OAVAttributeType guest_has_hobbies;
public static final OAVAttributeType guest_has_hobby;
/** The last seat type. */
public static final OAVObjectType lastseat_type;
/** A last seat has a set. */
public static final OAVAttributeType lastseat_has_seat;
/** The seating type. */
public static final OAVObjectType seating_type;
/** A seating has a seat1. */
public static final OAVAttributeType seating_has_seat1;
/** A seating has a seat2. */
public static final OAVAttributeType seating_has_seat2;
/** A seating has a seat1. */
public static final OAVAttributeType seating_has_name1;
/** A seating has a seat2. */
public static final OAVAttributeType seating_has_name2;
/** A seating has an id. */
public static final OAVAttributeType seating_has_id;
/** A seating has an pid. */
public static final OAVAttributeType seating_has_pid;
/** A seating has an pathdone. */
public static final OAVAttributeType seating_has_pathdone;
/** The context type. */
public static final OAVObjectType context_type;
/** A context has a state. */
public static final OAVAttributeType context_has_state;
/** The path type. */
public static final OAVObjectType path_type;
/** A path has an id. */
public static final OAVAttributeType path_has_id;
/** A path has a name. */
public static final OAVAttributeType path_has_name;
/** A path has a seat. */
public static final OAVAttributeType path_has_seat;
/** The chosen type. */
public static final OAVObjectType chosen_type;
/** A chosen has an id. */
public static final OAVAttributeType chosen_has_id;
/** A chosen has a name. */
public static final OAVAttributeType chosen_has_name;
/** A chosen has a hobby. */
public static final OAVAttributeType chosen_has_hobby;
/** The count type. */
public static final OAVObjectType count_type;
/** A count has a c. */
public static final OAVAttributeType count_has_c;
static
{
manners_type_model = new OAVTypeModel("manners_type_model");
manners_type_model.addTypeModel(OAVJavaType.java_type_model);
guest_type = manners_type_model.createType("guest");
guest_has_name = guest_type.createAttributeType("guest_has_name", OAVJavaType.java_string_type);
guest_has_sex = guest_type.createAttributeType("guest_has_sex", OAVJavaType.java_string_type);
guest_has_hobby = guest_type.createAttributeType("guest_has_hobby", OAVJavaType.java_string_type);
lastseat_type = manners_type_model.createType("lastseat");
lastseat_has_seat = lastseat_type.createAttributeType("lastseat_has_seat", OAVJavaType.java_integer_type);
seating_type = manners_type_model.createType("seating");
seating_has_seat1 = seating_type.createAttributeType("seating_has_seat1", OAVJavaType.java_integer_type);
seating_has_seat2 = seating_type.createAttributeType("seating_has_seat2", OAVJavaType.java_integer_type);
seating_has_name1 = seating_type.createAttributeType("seating_has_name1", OAVJavaType.java_string_type);
seating_has_name2 = seating_type.createAttributeType("seating_has_name2", OAVJavaType.java_string_type);
seating_has_id = seating_type.createAttributeType("seating_has_id", OAVJavaType.java_integer_type);
seating_has_pid = seating_type.createAttributeType("seating_has_pid", OAVJavaType.java_integer_type);
seating_has_pathdone = seating_type.createAttributeType("seating_has_pathdone", OAVJavaType.java_boolean_type, OAVAttributeType.NONE, Boolean.FALSE);
context_type = manners_type_model.createType("context");
context_has_state = context_type.createAttributeType("context_has_state", OAVJavaType.java_string_type);
path_type = manners_type_model.createType("path");
path_has_id = path_type.createAttributeType("path_has_id", OAVJavaType.java_integer_type);
path_has_name = path_type.createAttributeType("path_has_name", OAVJavaType.java_string_type);
path_has_seat = path_type.createAttributeType("path_has_seat", OAVJavaType.java_integer_type);
chosen_type = manners_type_model.createType("chosen");
chosen_has_id = chosen_type.createAttributeType("chosen_has_id", OAVJavaType.java_integer_type);
chosen_has_name = chosen_type.createAttributeType("chosen_has_name", OAVJavaType.java_string_type);
chosen_has_hobby = chosen_type.createAttributeType("chosen_has_hobby", OAVJavaType.java_string_type);
count_type = manners_type_model.createType("count");
count_has_c = count_type.createAttributeType("count_has_c", OAVJavaType.java_integer_type);
}
/**
* Main for testing.
*/
public static void main(String[] args)
{
String facts = "manners4.fct";
if(args.length==1)
{
facts = args[0];
}
else
{
if(args.length>1)
System.out.println("USAGE: java "+Manners.class.getName()+" []");
System.out.println("Using default facts file: "+facts);
}
IOAVState state = OAVStateFactory.createOAVState(manners_type_model);
loadData(state, facts);
// print = true;
// IMannersRuleSet ruleset = new MannersRulesClips();
IMannersRuleSet ruleset = new MannersRulesJCL();
// IMannersRuleSet ruleset = new MannersRules();
Rulebase rb = new Rulebase();
rb.addRule(ruleset.createAssignFirstSeatRule());
rb.addRule(ruleset.createFindSeatingRule());
// Switched make-path and path-done to assure that
// make-path activations get executed first in LIFO strategy
rb.addRule(ruleset.createPathDoneRule());
rb.addRule(ruleset.createMakePathRule());
// Switched are-we-done and continue to assure that
// are-we-done activations get executed first in LIFO strategy
rb.addRule(ruleset.createContinueRule());
rb.addRule(ruleset.createAreWeDoneRule());
rb.addRule(ruleset.createPrintResultsRule());
rb.addRule(ruleset.createAllDoneRule());
// Initialize rule system.
RuleSystem rete = new RuleSystem(state, rb, new RetePatternMatcherFunctionality(rb), new LIFOAgenda());
rete.init();
// rete.getAgenda().setHistoryEnabled(true);
state.notifyEventListeners();
RuleSystemExecutor exe = new RuleSystemExecutor(rete, true);
RuleEnginePanel.createRuleEngineFrame(exe, "Miss Manners Rete Structure");
}
/**
* Load a manners data file.
*/
protected static void loadData(IOAVState state, String file)
{
try
{
File facts = new File(file);
LineNumberReader lnr;
if(facts.exists())
{
lnr = new LineNumberReader(new FileReader(facts));
}
else
{
lnr = new LineNumberReader(new InputStreamReader(Manners.class.getResourceAsStream(file)));
}
String line = lnr.readLine();
while(line!=null)
{
if(line.startsWith("(guest"))
{
int index = line.indexOf("(name ");
String name = line.substring(index+6, line.indexOf(")", index));
index = line.indexOf("(sex ");
String sex = line.substring(index+5, line.indexOf(")", index));
index = line.indexOf("(hobby ");
String hobby = line.substring(index+7, line.indexOf(")", index));
Object guest = state.createRootObject(guest_type);
state.setAttributeValue(guest, guest_has_name, name);
state.setAttributeValue(guest, guest_has_sex, sex);
state.setAttributeValue(guest, guest_has_hobby, hobby);
System.out.println("Created guest: name="+name+", sex="+sex+", hobby="+hobby);
}
else if(line.startsWith("(last_seat"))
{
int index = line.indexOf("(seat ");
Integer seat = Integer.valueOf(line.substring(index+6, line.indexOf(")", index)));
Object lastseat = state.createRootObject(lastseat_type);
state.setAttributeValue(lastseat, lastseat_has_seat, seat);
System.out.println("Created lastseat: seat="+seat);
}
else if(line.startsWith("(count"))
{
Object count = state.createRootObject(count_type);
state.setAttributeValue(count, count_has_c, Integer.valueOf(1));
System.out.println("Initialized count: c=1");
}
else if(line.startsWith("(context"))
{
Object context = state.createRootObject(context_type);
state.setAttributeValue(context, context_has_state, "start");
System.out.println("Initialized context: state=start");
}
else if(!line.trim().equals(""))
{
throw new RuntimeException("Cannot handle '"+line+"'.");
}
line = lnr.readLine();
}
lnr.close();
}
catch(IOException e)
{
throw new RuntimeException("Error loading: "+file, e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy