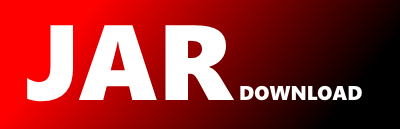
jadex.base.gui.asynctree.AsyncTreeModel Maven / Gradle / Ivy
package jadex.base.gui.asynctree;
import jadex.commons.collection.MultiCollection;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.Set;
import javax.swing.SwingUtilities;
import javax.swing.event.TreeModelEvent;
import javax.swing.event.TreeModelListener;
import javax.swing.tree.TreeModel;
import javax.swing.tree.TreePath;
/**
* Tree model, which dynamically represents running components.
*/
public class AsyncTreeModel implements TreeModel
{
//-------- attributes --------
/** The root node. */
protected ITreeNode root;
/** The tree listeners. */
protected final List listeners;
/** The node listeners. */
protected final List nodelisteners;
/** The node lookup table. */
protected final Map nodes;
/** The added nodes. */
protected final Map added;
/** The zombie node ids (id->remove counter). */
protected final Map zombies;
/** The icon overlays. */
protected final List overlays;
/** The changed nodes (delayed update for improving perceived speed). */
protected MultiCollection changed;
//-------- constructors --------
/**
* Create a component tree model.
*/
public AsyncTreeModel()
{
this.listeners = new ArrayList();
this.nodelisteners = new ArrayList();
this.nodes = new HashMap();
this.added = new HashMap();
this.zombies = new HashMap();
this.overlays = new ArrayList();
}
//-------- TreeModel interface --------
/**
* Get the root node.
*/
public Object getRoot()
{
assert SwingUtilities.isEventDispatchThread();// || Starter.isShutdown();
return root;
}
/**
* Get the given child of a node.
*/
public Object getChild(Object parent, int index)
{
assert SwingUtilities.isEventDispatchThread();// || Starter.isShutdown();
return ((ITreeNode)parent).getChild(index);
}
/**
* Get the number of children of a node.
*/
public int getChildCount(Object parent)
{
assert SwingUtilities.isEventDispatchThread();// || Starter.isShutdown();
return ((ITreeNode)parent).getChildCount();
}
/**
* Get the index of a child.
*/
public int getIndexOfChild(Object parent, Object child)
{
assert SwingUtilities.isEventDispatchThread();// || Starter.isShutdown();
return ((ITreeNode)parent).getIndexOfChild((ITreeNode)child);
}
/**
* Test if the node is a leaf.
*/
public boolean isLeaf(Object node)
{
assert SwingUtilities.isEventDispatchThread();// || Starter.isShutdown();
return ((ITreeNode)node).isLeaf();
}
/**
* Edit the value of a node.
*/
public void valueForPathChanged(TreePath path, Object newValue)
{
assert SwingUtilities.isEventDispatchThread();// || Starter.isShutdown();
throw new UnsupportedOperationException("Component Tree is not editable.");
}
/**
* Add a listener.
*/
public void addTreeModelListener(TreeModelListener l)
{
assert SwingUtilities.isEventDispatchThread();// || Starter.isShutdown();
this.listeners.add(l);
}
/**
* Remove a listener.
*/
public void removeTreeModelListener(TreeModelListener l)
{
assert SwingUtilities.isEventDispatchThread();// || Starter.isShutdown();
this.listeners.remove(l);
}
//-------- helper methods --------
/**
* Set the root node.
*/
public void setRoot(ITreeNode root)
{
assert SwingUtilities.isEventDispatchThread();// || Starter.isShutdown();
if(this.root!=null)
deregisterNode(this.root);
this.root = root;
if(root!=null)
addNode(root);
// System.err.println(""+hashCode()+" set root");
fireTreeChanged(root);
}
/**
* Inform listeners that tree has changed from given node on.
*/
public void fireTreeChanged(ITreeNode node)
{
assert SwingUtilities.isEventDispatchThread();// || Starter.isShutdown();
List path = buildTreePath(node);
// System.err.println(""+hashCode()+" Path changed: "+node+", "+path+", "+node.getCachedChildren());
for(int i=0; i1)
{
num = new Integer(num!=null ? num.intValue()-1 : 1);
zombies.put(node.getId(), num);
// if(node.getId().toString().startsWith("ANDTest@"))
// System.out.println("Zombie node count decreased: "+node+", "+num);
}
else
{
// if(node.getId().toString().startsWith("ANDTest@"))
// System.out.println("Zombie node removed: "+node+", "+num);
deregisterNode(node);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy