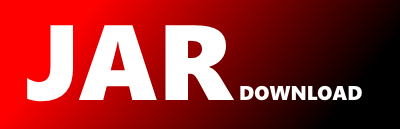
jadex.base.gui.config.PlatformConfigPanel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jadex-tools-base-swing Show documentation
Show all versions of jadex-tools-base-swing Show documentation
Reusable classes for starting Jadex, running test cases and building
JCC plugins.
package jadex.base.gui.config;
import jadex.base.Starter;
import jadex.bridge.modelinfo.IModelInfo;
import jadex.bridge.service.types.factory.SBootstrapLoader;
import jadex.commons.Properties;
import jadex.commons.Property;
import jadex.commons.SReflect;
import jadex.commons.SUtil;
import jadex.commons.future.Future;
import jadex.commons.future.IFuture;
import jadex.commons.gui.PropertiesPanel;
import jadex.commons.gui.SGUI;
import jadex.commons.gui.future.SwingDefaultResultListener;
import jadex.commons.gui.future.SwingExceptionDelegationResultListener;
import jadex.xml.PropertiesXMLHelper;
import java.awt.BorderLayout;
import java.awt.Component;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.util.ArrayList;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import javax.swing.JButton;
import javax.swing.JComboBox;
import javax.swing.JComponent;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JTabbedPane;
import javax.swing.JTable;
import javax.swing.SwingUtilities;
import javax.swing.UIManager;
import javax.swing.filechooser.FileFilter;
import javax.swing.table.DefaultTableCellRenderer;
/**
* A panel to configure and start a Jadex platform.
*/
public class PlatformConfigPanel extends JPanel
{
// /** The default factories for given extensions. */
// public static Map FACTORIES;
//
// /** The default platform models. */
// public static Set MODELS;
//
// static
// {
// // Todo: externalize/dynamically check available factories!?
// FACTORIES = new LinkedHashMap();
// FACTORIES.put(".component.xml", "jadex.component.ComponentComponentFactory");
// FACTORIES.put("Agent.class", "jadex.micro.MicroAgentFactory");
// FACTORIES.put(".agent.xml", "jadex.bdi.BDIAgentFactory");
// FACTORIES.put(".bpmn", "jadex.bpmn.BpmnFactory");
// FACTORIES.put(".application.xml", "jadex.application.ApplicationComponentFactory");
// FACTORIES.put(".gpmn", "jadex.gpmn.GpmnFactory");
//
// // Todo: externalize/dynamically check available platform models!?
// MODELS = new LinkedHashSet();
// MODELS.add("jadex.standalone.Platform.component.xml");
// MODELS.add("jadex.standalone.PlatformAgent.class");
// MODELS.add("jadex.standalone.Platform.bpmn");
// }
//-------- attributes --------
/** The argument panel (stored for later removal). */
protected Component argpanel;
/** The argument table model (stored for changing the configuration). */
protected ArgumentTableModel argmodel;
/** The argument table model (stored for changing the configuration). */
protected ClasspathPanel classpath;
/** The found factories (component file suffix -> factory class name). */
protected Map factories;
//-------- constructors --------
/**
* Create a platform config panel.
*/
public PlatformConfigPanel(final ClasspathPanel classpath)
{
super(new BorderLayout());
this.classpath = classpath;
this.factories = new LinkedHashMap();
final PropertiesPanel modelpanel = new PropertiesPanel("Platform Model");
// final JComboBox model = modelpanel.createComboBox("Model", MODELS.toArray(new String[MODELS.size()]), true, 0);
// final JComboBox factory = modelpanel.createComboBox("Factory", FACTORIES.values().toArray(new String[FACTORIES.size()]), true, 0);
final JComboBox model = modelpanel.createComboBox("Model", SUtil.EMPTY_STRING_ARRAY, true, 0);
final JComboBox factory = modelpanel.createComboBox("Factory", SUtil.EMPTY_STRING_ARRAY, true, 0);
final JComboBox config = modelpanel.createComboBox("Configuration", SUtil.EMPTY_STRING_ARRAY, false, 0);
config.setEnabled(false);
JButton[] buts = modelpanel.createButtons("buts", new String[]
{
"Load Model", "Scan for Factories", "Scan for Models"
}, 0);
JButton[] buts1 = modelpanel.createButtons("buts", new String[]
{
"Load Settings...", "Save Settings...", "Start Platform"
}, 0);
model.addActionListener(new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
// When platform model is selected, choose appropriate factory, if any.
for(String suffix: factories.keySet())
{
if(((String)model.getSelectedItem()).endsWith(suffix))
{
factory.setSelectedItem(factories.get(suffix));
break;
}
}
}
});
config.addActionListener(new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
if(argmodel!=null)
{
argmodel.setConfiguration((String)config.getSelectedItem());
}
}
});
buts[0].addActionListener(new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
loadModel(model, factory, config)
.addResultListener(new SwingDefaultResultListener(PlatformConfigPanel.this)
{
public void customResultAvailable(Void result)
{
}
});
}
});
buts[1].addActionListener(new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
Class>[] facts = classpath.scanForFactories();
factories.clear();
factory.removeAllItems();
for(int i=0; i(PlatformConfigPanel.this)
{
public void customResultAvailable(Void result)
{
if(props.getProperty(Starter.CONFIGURATION_NAME)!=null)
{
config.setSelectedItem(props.getStringProperty(Starter.CONFIGURATION_NAME));
}
Property[] argprops = props.getProperties("argument");
for(int i=0; i margs = argmodel.getArguments();
for(String arg: margs.keySet())
{
props.addProperty(new Property(arg, "argument", margs.get(arg)));
}
}
props.addSubproperties("Classpath", classpath.getProperties());
int ok = fc.showOpenDialog(PlatformConfigPanel.this);
if(ok==JFileChooser.APPROVE_OPTION)
{
try
{
String filename = fc.getSelectedFile().getPath();
if(!filename.endsWith(".launch.xml"))
filename += ".launch.xml";
FileOutputStream os = new FileOutputStream(new File(filename));
PropertiesXMLHelper.write(props, os, getClass().getClassLoader());
os.close();
}
catch(Exception ex)
{
SGUI.showError(PlatformConfigPanel.this, "Save Problem", "Warning: Could not save settings: "+ex, ex);
}
}
}
});
buts1[2].addActionListener(new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
List args = new ArrayList();
args.add("-"+Starter.COMPONENT_FACTORY);
args.add((String)factory.getSelectedItem());
args.add("-"+Starter.CONFIGURATION_FILE);
args.add((String)model.getSelectedItem());
if(config.isEnabled())
{
args.add("-"+Starter.CONFIGURATION_NAME);
args.add((String)config.getSelectedItem());
}
if(argmodel!=null)
{
Map margs = argmodel.getArguments();
for(String arg: margs.keySet())
{
args.add("-"+arg);
args.add(margs.get(arg));
}
}
try
{
Class> starterclass = SReflect.classForName(Starter.class.getName(), classpath.getClassLoader());
Object fut = starterclass.getMethod("createPlatform", new Class>[]{String[].class}).
invoke(null, new Object[]{args.toArray(new String[args.size()])});
}
catch(Exception ex)
{
// TODO: handle exception
ex.printStackTrace();
}
// .addResultListener(new SwingDefaultResultListener(PlatformConfigPanel.this)
// {
// public void customResultAvailable(IExternalAccess result)
// {
// }
// });
}
});
this.add(modelpanel, BorderLayout.NORTH);
}
/**
* Load a model.
*/
public IFuture loadModel(JComboBox model, JComboBox factory, final JComboBox config)
{
final Future ret = new Future();
SBootstrapLoader.loadModel(classpath.getClassLoader(), (String)model.getSelectedItem(), (String)factory.getSelectedItem())
.addResultListener(new SwingExceptionDelegationResultListener(ret)
{
public void customResultAvailable(final IModelInfo mi)
{
if(argpanel!=null)
{
PlatformConfigPanel.this.remove(argpanel);
}
config.removeAllItems();
if(mi.getConfigurationNames().length>0)
{
for(int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy