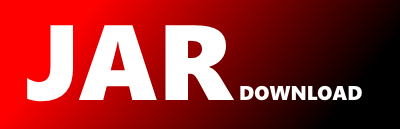
net.sourceforge.javadpkg.DebianPackageBuilder Maven / Gradle / Ivy
/*
* dpkg - Debian Package library and the Debian Package Maven plugin
* (c) Copyright 2015 Gerrit Hohl
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU General Public License
* as published by the Free Software Foundation; either version 2
* of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.
*/
package net.sourceforge.javadpkg;
import java.io.IOException;
import net.sourceforge.javadpkg.control.Control;
import net.sourceforge.javadpkg.control.Size;
import net.sourceforge.javadpkg.io.DataSource;
import net.sourceforge.javadpkg.io.DataTarget;
import net.sourceforge.javadpkg.io.FileMode;
import net.sourceforge.javadpkg.io.FileOwner;
/**
*
* A builder for Debian packages.
*
*
* @author Gerrit Hohl ([email protected])
* @version 1.0, 27.12.2015 by Gerrit Hohl
*/
public interface DebianPackageBuilder {
/**
*
* Sets the control information.
*
*
* If no installed size is specified in the control information the builder
* will sum up the sizes of the specified files and use that value as
* installed size.
*
*
* @param control
* The control information.
*/
void setControl(Control control);
/**
*
* Sets the overhead which will be added to the specified installed size.
*
*
* If a installed size is specified in the control information passed to the
* {@link #setControl(Control)} method this size will be added. If no
* installed size is specified the builder will sum up the sizes of the
* specified files and add the overhead.
*
*
* The overhead can be used for files which will be created after the
* installation. This way space can be "reserved".
*
*
* @param installedSizeOverhead
* The overhead.
*/
void setInstalledSizeOverhead(Size installedSizeOverhead);
/**
*
* Sets the script which will be executed before the package is installed.
*
*
* @param preInstall
* The script or null
, if the standard script should
* be used.
*/
void setPreInstall(Script preInstall);
/**
*
* Sets the script which will be executed after the package is installed.
*
*
* @param postInstall
* The script or null
, if the standard script should
* be used.
*/
void setPostInstall(Script postInstall);
/**
*
* Sets the script which will be executed before the package is removed.
*
*
* @param preRemove
* The script or null
, if the standard script should
* be used.
*/
void setPreRemove(Script preRemove);
/**
*
* Sets the script which will be executed after the package is removed.
*
*
* @param postRemove
* The script or null
, if the standard script should
* be used.
*/
void setPostRemove(Script postRemove);
/**
*
* Adds a directory to the data of the package.
*
*
* This method is equal to the method
* {@link #addDataDirectory(String, FileOwner, FileMode)} using the
* following values:
*
*
* group ID
* group name
* user ID
* user name
* mode (octal)
*
*
* 0
* root
* 0
* root
* 0755
*
*
*
*
* @param path
* The installation path.
* @throws IllegalArgumentException
* If the installation path is null
.
* @throws IllegalStateException
* If the parent directory wasn't added before. Only exception
* is the root "/" directory which always exists.
*/
void addDataDirectory(String path);
/**
*
* Adds a directory to the data of the package.
*
*
* @param path
* The installation path.
* @param owner
* The owner.
* @param mode
* The mode.
* @throws IllegalArgumentException
* If any of the parameters are null
.
* @throws IllegalStateException
* If the parent directory wasn't added before. Only exception
* is the root "/" directory which always exists.
*/
void addDataDirectory(String path, FileOwner owner, FileMode mode);
/**
*
* Adds a file to the data of the package.
*
*
* This method is equal to the method
* {@link #addDataFile(DataSource, String, FileOwner, FileMode)} using the
* following values:
*
*
* group ID
* group name
* user ID
* user name
* mode (octal)
*
*
* 0
* root
* 0
* root
* 0644
*
*
*
*
* @param source
* The source for the context of the file.
* @param path
* The installation path.
* @throws IllegalArgumentException
* If any of the parameters are null
.
* @throws IllegalStateException
* If the parent directory wasn't added before. Only exception
* is the root "/" directory which always exists.
*/
void addDataFile(DataSource source, String path);
/**
*
* Adds a file to the data of the package.
*
*
* @param source
* The source for the context of the file.
* @param path
* The installation path.
* @param owner
* The owner.
* @param mode
* The mode.
* @throws IllegalArgumentException
* If any of the parameters are null
.
* @throws IllegalStateException
* If the parent directory wasn't added before. Only exception
* is the root "/" directory which always exists.
*/
void addDataFile(DataSource source, String path, FileOwner owner, FileMode mode);
/**
*
* Adds a symbolic link to the data of the package.
*
*
* @param path
* The installation path.
* @param target
* The target path.
* @param owner
* The owner.
* @param mode
* The mode.
* @throws IllegalArgumentException
* If any of the parameters are null
.
* @throws IllegalStateException
* If the parent directory wasn't added before. Only exception
* is the root "/" directory which always exists.
*/
void addDataSymLink(String path, String target, FileOwner owner, FileMode mode);
/**
*
* Sets the copyright.
*
*
* @param copyright
* The copyright.
*/
void setCopyright(Copyright copyright);
/**
*
* Sets the change log.
*
*
* @param changeLog
* The change log.
*/
void setChangeLog(ChangeLog changeLog);
/**
*
* Builds a Debian package by writing it to the specified target.
*
*
* @param target
* The target
* @param context
* The context.
* @throws IllegalArgumentException
* If any of the parameters are null
.
* @throws IllegalStateException
* If no control information, no copyright and/or no change log
* is set.
* @throws IOException
* If an I/O error occurs while writing the file.
* @throws BuildException
* If an error occurs during the building.
* @see #setControl(Control)
*/
void buildDebianPackage(DataTarget target, Context context) throws IOException, BuildException;
}