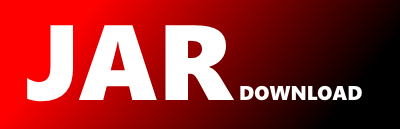
net.sourceforge.javadpkg.DocumentPaths Maven / Gradle / Ivy
/*
* dpkg - Debian Package library and the Debian Package Maven plugin
* (c) Copyright 2016 Gerrit Hohl
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU General Public License
* as published by the Free Software Foundation; either version 2
* of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.
*/
package net.sourceforge.javadpkg;
/**
*
* The paths of the files in the document folder of the Debian package.
*
*
* @author Gerrit Hohl ([email protected])
* @version 1.0, 06.05.2016 by Gerrit Hohl
*/
public interface DocumentPaths {
/**
*
* Returns the base path of the document folder.
*
*
* Normally this should be:
* /usr/share/doc/
*
*
* @return The base path.
*/
String getDocumentBasePath();
/**
*
* Returns the path of the document folder of the Debian package.
*
*
* Normally this should be:
* /usr/share/doc/[package-name]/
*
*
* @return The path.
*/
String getDocumentPath();
/**
*
* Returns the flag if the specified path is the path of the copyright file.
*
*
* @param path
* The path.
* @return The flag: true
, if the path is the path of the
* copyright file, false
otherwise.
* @throws IllegalArgumentException
* If the path is null
.
*/
boolean isCopyrightPath(String path);
/**
*
* Returns the path of the copyright file.
*
*
* Normally this should be:
* /usr/share/doc/[package-name]/copyright
*
*
* @return The path.
*/
String getCopyrightPath();
/**
*
* Returns the flag if the specified path is the path of the change log
* file.
*
*
* The method doesn't determine the format and/or compression of the change
* log file.
*
*
* @param path
* The path.
* @return The flag: true
, if the path is the path of the
* change log file, false
otherwise.
* @throws IllegalArgumentException
* If the path is null
.
* @see #isChangeLogGzipPath(String)
* @see #isChangeLogHtmlPath(String)
*/
boolean isChangeLogPath(String path);
/**
*
* Returns the flag if the specified path is the path of the Debian change
* log file.
*
*
*
* The filename of the Debian change log starts with
* "changelog.Debian.*". It often exists parallel to the
* "changelog.*" file which in this case has its own, non-standard
* format. If both files exists the Debian change log should always be used.
*
*
* @param path
* The path.
* @return The flag: true
, if the path is the path of the
* Debian change log file, false
otherwise.
* @throws IllegalArgumentException
* If the path is null
.
*/
boolean isChangeLogDebianPath(String path);
/**
*
* Returns the flag if the specified path is the path of a GZIP compressed
* change log file.
*
*
* The method doesn't determine the format of the change log file.
*
*
* @param path
* The path.
* @return The flag: true
, if the path is the path of a GZIP
* compressed change log file, false
otherwise.
* @see #isChangeLogHtmlPath(String)
*/
boolean isChangeLogGzipPath(String path);
/**
*
* Returns the flag if the specified path is the path of a HTML formatted
* change log file.
*
*
* The method doesn't determine the compression of the change log file.
*
*
* @param path
* The path.
* @return The flag: true
, if the path is the path of a HTML
* formatted change log file, false
otherwise.
* @see #isChangeLogGzipPath(String)
*/
boolean isChangeLogHtmlPath(String path);
/**
*
* Returns the path of the change log file.
*
*
* Normally this should be:
* /usr/share/doc/[package-name]/changelog
*
*
* @return The path.
*/
String getChangeLogPath();
/**
*
* Returns the path of the GZIP compressed change log file.
*
*
* Normally this should be:
* /usr/share/doc/[package-name]/changelog.gz
*
*
* @return The path.
*/
String getChangeLogGzipPath();
/**
*
* Returns the path of the HTML formatted change log file.
*
*
* Normally this should be:
* /usr/share/doc/[package-name]/changelog.html
*
*
* @return The path.
*/
String getChangeLogHtmlPath();
/**
*
* Returns the path of the GZIP compressed HTML formatted change log file.
*
*
* Normally this should be:
* /usr/share/doc/[package-name]/changelog.html.gz
*
*
* @return The path.
*/
String getChangeLogHtmlGzipPath();
}