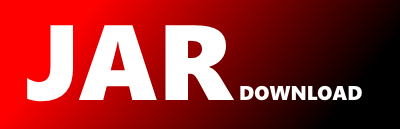
net.sourceforge.javadpkg.control.PackageDependency Maven / Gradle / Ivy
/*
* dpkg - Debian Package library and the Debian Package Maven plugin
* (c) Copyright 2015 Gerrit Hohl
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU General Public License
* as published by the Free Software Foundation; either version 2
* of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.
*/
package net.sourceforge.javadpkg.control;
import java.util.List;
/**
*
* The dependency of a package to other packages.
*
*
* See
* Chapter 7 - Declaring relationships between packages for further
* information.
*
*
* @author Gerrit Hohl ([email protected])
* @version 1.0, 31.12.2015 by Gerrit Hohl
*/
public interface PackageDependency {
/*
* TODO Split that interface later in a super interface for package dependencies and a sub interface for normal
* package dependencies (package, relation operator, version) and conditional package dependencies (condition and list
* of normal package dependencies).
*/
/**
*
* A condition for a package dependency.
*
*
* @author Gerrit Hohl ([email protected])
* @version 1.0, 03.01.2016 by Gerrit Hohl
*/
public enum Condition {
/** An or condition. */
OR
}
/**
*
* Returns the flag if this package dependency is a condition.
*
*
* If the package dependency is a condition the {@link #getCondition()} and
* {@link #getConditionPackageDependencies()} methods must be used.
* Otherwise the {@link #getPackage()}, {@link #getRelationOperator()} and
* {@link #getVersion()} methods must be used.
*
*
* @return The flag: true
, if this package dependency is a
* condition, false
otherwise.
*/
boolean isCondition();
/**
*
* Returns the condition.
*
*
* @return The condition or null
, if this package dependency is
* a not a condition.
* @see #isCondition()
*/
Condition getCondition();
/**
*
* Returns the package dependencies for the condition.
*
*
* @return The package dependencies or an empty list, if this package
* dependency is a not a condition.
* @see #isCondition()
*/
List getConditionPackageDependencies();
/**
*
* Returns the name of the package.
*
*
* @return The name or null
, if this package dependency is a
* condition.
* @see #isCondition()
*/
PackageName getPackage();
/**
*
* Returns the relational operator.
*
*
* @return The relational operator or null
, if no version is
* specified or this package dependency is a condition.
* @see #isCondition()
*/
PackageVersionRelationOperator getRelationOperator();
/**
*
* Returns the version.
*
*
* @return The version or null
, if no version is specified or
* this package dependency is a condition.
* @see #isCondition()
*/
PackageVersion getVersion();
}