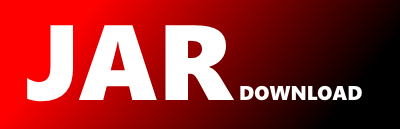
net.sourceforge.javadpkg.control.impl.BinaryControlImpl Maven / Gradle / Ivy
/*
* dpkg - Debian Package library and the Debian Package Maven plugin
* (c) Copyright 2015 Gerrit Hohl
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU General Public License
* as published by the Free Software Foundation; either version 2
* of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.
*/
package net.sourceforge.javadpkg.control.impl;
import java.util.ArrayList;
import java.util.LinkedList;
import java.util.List;
import net.sourceforge.javadpkg.control.Architecture;
import net.sourceforge.javadpkg.control.BinaryControl;
import net.sourceforge.javadpkg.control.Description;
import net.sourceforge.javadpkg.control.Homepage;
import net.sourceforge.javadpkg.control.PackageDependency;
import net.sourceforge.javadpkg.control.PackageMaintainer;
import net.sourceforge.javadpkg.control.PackageMultiArchitecture;
import net.sourceforge.javadpkg.control.PackageName;
import net.sourceforge.javadpkg.control.PackagePriority;
import net.sourceforge.javadpkg.control.PackageVersion;
import net.sourceforge.javadpkg.control.Section;
import net.sourceforge.javadpkg.control.Size;
/**
*
* A {@link BinaryControl} implementation.
*
*
* @author Gerrit Hohl ([email protected])
* @version 1.0, 31.12.2015 by Gerrit Hohl
*/
public class BinaryControlImpl extends AbstractControl implements BinaryControl {
/** The name of the binary package. */
private PackageName packageName;
/** The section. */
private Section section;
/** The priority. */
private PackagePriority priority;
/**
*
* The flag if the package is essential for the system and can't be removed.
*
*/
private Boolean essential;
/** The packages on which this package depends on. */
private List depends;
/**
*
* The packages which this package has a strong, but not absolute,
* dependency.
*
*/
private List recommends;
/**
*
* The packages which this package suggests to install for enhancing its
* functionality.
*
*/
private List suggest;
/**
*
* The packages which are enhanced in their functionality by this package.
*
*/
private List enhances;
/**
*
* The packages which must be installed before this package is installed.
*
*/
private List preDepends;
/** The packages which will "broken" by this package. */
private List breaks;
/** The packages which will be in conflict with this package. */
private List conflicts;
/** The virtual packages which are provided by this package. */
private List provides;
/** The packages which are replaced by this package. */
private List replaces;
/** The packages which were used to built this package. */
private List builtUsing;
/**
*
* The estimate of the total amount of disk space required to install this
* package.
*
*/
private Size installedSize;
/** The module aliases. */
private String moduleAliases;
/** The description. */
private Description description;
/**
*
* Creates a binary control.
*
*/
public BinaryControlImpl() {
super();
this.packageName = null;
this.section = null;
this.priority = null;
this.essential = null;
this.depends = new LinkedList<>();
this.recommends = new LinkedList<>();
this.suggest = new LinkedList<>();
this.enhances = new LinkedList<>();
this.preDepends = new LinkedList<>();
this.breaks = new LinkedList<>();
this.conflicts = new LinkedList<>();
this.provides = new LinkedList<>();
this.replaces = new LinkedList<>();
this.builtUsing = new LinkedList<>();
this.installedSize = null;
this.moduleAliases = null;
this.description = null;
}
/**
*
* Creates a binary control.
*
*
* @param source
* The source package dependency.
* @param version
* The version of the package.
* @param architecture
* The architecture.
* @param multiArchitecture
* The multiple architecture property.
* @param maintainer
* The maintainer.
* @param homepage
* The home-page.
* @param packageName
* The name of the binary package.
* @param section
* The section.
* @param priority
* The priority.
* @param essential
* The flag if the package is essential for the system and can't
* be removed.
* @param depends
* The packages on which this package depends on.
* @param recommends
* The packages which this package has a strong, but not
* absolute, dependency.
* @param suggest
* The packages which this package suggests to install for
* enhancing its functionality.
* @param enhances
* The packages which are enhanced in their functionality by this
* package.
* @param preDepends
* The packages which must be installed before this package is
* installed.
* @param breaks
* The packages which will "broken" by this package.
* @param conflicts
* The packages which will be in conflict with this package.
* @param provides
* The virtual packages which are provided by this package.
* @param replaces
* The packages which are replaced by this package.
* @param builtUsing
* The packages which were used to built this package.
* @param installedSize
* The estimate of the total amount of disk space required to
* install this package.
* @param description
* The description.
*/
public BinaryControlImpl(PackageDependency source, PackageVersion version, Architecture architecture,
PackageMultiArchitecture multiArchitecture, PackageMaintainer maintainer, Homepage homepage,
PackageName packageName, Section section, PackagePriority priority, Boolean essential,
List depends, List recommends, List suggest,
List enhances, List preDepends, List breaks,
List conflicts, List provides, List replaces,
List builtUsing, Size installedSize, Description description) {
super(source, version, architecture, multiArchitecture, maintainer, homepage);
this.packageName = packageName;
this.section = section;
this.priority = priority;
this.essential = essential;
this.depends = getList(depends);
this.recommends = getList(recommends);
this.suggest = getList(suggest);
this.enhances = getList(enhances);
this.preDepends = getList(preDepends);
this.breaks = getList(breaks);
this.conflicts = getList(conflicts);
this.provides = getList(provides);
this.replaces = getList(replaces);
this.builtUsing = getList(builtUsing);
this.installedSize = null;
this.description = null;
}
/**
*
* Returns a list.
*
*
* If a list is passed a copy of that list will be returned. Otherwise an
* empty list will be returned.
*
*
* @param list
* The list (optional).
* @return The list.
*/
private static List getList(List list) {
if (list == null)
return new LinkedList<>();
return (new LinkedList<>(list));
}
@Override
public PackageName getPackage() {
return this.packageName;
}
/**
*
* Sets the name of the binary package.
*
*
* @param packageName
* The name.
*/
public void setPackage(PackageName packageName) {
this.packageName = packageName;
}
@Override
public Section getSection() {
return this.section;
}
/**
*
* Sets the section.
*
*
* @param section
* The section.
*/
public void setSection(Section section) {
this.section = section;
}
@Override
public PackagePriority getPriority() {
return this.priority;
}
/**
*
* Sets the priority.
*
*
* @param priority
* The priority.
*/
public void setPriority(PackagePriority priority) {
this.priority = priority;
}
@Override
public Boolean getEssential() {
return this.essential;
}
/**
*
* Sets the flag if the package is essential for the system and can't be
* removed.
*
*
* @param essential
* The flag.
*/
public void setEssential(Boolean essential) {
this.essential = essential;
}
@Override
public List getDepends() {
return (new ArrayList<>(this.depends));
}
/**
*
* Sets the the packages on which this package depends on.
*
*
* @param depends
* The dependencies.
*/
public void setDepends(List depends) {
this.depends = getList(depends);
}
@Override
public List getRecommends() {
return (new ArrayList<>(this.recommends));
}
/**
*
* Sets the packages which this package has a strong, but not absolute,
* dependency.
*
*
* @param recommends
* The dependencies.
*/
public void setRecommends(List recommends) {
this.recommends = getList(recommends);
}
@Override
public List getSuggests() {
return (new ArrayList<>(this.suggest));
}
/**
*
* Sets the packages which this package suggests to install for enhancing
* its functionality.
*
*
* @param suggest
* The dependencies.
*/
public void setSuggests(List suggest) {
this.suggest = getList(suggest);
}
@Override
public List getEnhances() {
return (new ArrayList<>(this.enhances));
}
/**
*
* Sets the packages which are enhanced in their functionality by this
* package.
*
*
* @param enhances
* The dependencies.
*/
public void setEnhances(List enhances) {
this.enhances = getList(enhances);
}
@Override
public List getPreDepends() {
return (new ArrayList<>(this.preDepends));
}
/**
*
* Sets the packages which must be installed before this package is
* installed.
*
*
* @param preDepends
* The dependencies.
*/
public void setPreDepends(List preDepends) {
this.preDepends = getList(preDepends);
}
@Override
public List getBreaks() {
return (new ArrayList<>(this.breaks));
}
/**
*
* Sets the packages which will "broken" by this package.
*
*
* @param breaks
* The dependencies.
*/
public void setBreaks(List breaks) {
this.breaks = getList(breaks);
}
@Override
public List getConflicts() {
return (new ArrayList<>(this.conflicts));
}
/**
*
* Sets the packages which will be in conflict with this package.
*
*
* @param conflicts
* The dependencies.
*/
public void setConflicts(List conflicts) {
this.conflicts = getList(conflicts);
}
@Override
public List getProvides() {
return (new ArrayList<>(this.provides));
}
/**
*
* Sets the virtual packages which are provided by this package.
*
*
* @param provides
* The dependencies.
*/
public void setProvides(List provides) {
this.provides = getList(provides);
}
@Override
public List getReplaces() {
return (new ArrayList<>(this.replaces));
}
/**
*
* Sets the packages which are replaced by this package.
*
*
* @param replaces
* The dependencies.
*/
public void setReplaces(List replaces) {
this.replaces = getList(replaces);
}
@Override
public List getBuiltUsing() {
return (new ArrayList<>(this.builtUsing));
}
/**
*
* Sets the packages which were used to built this package.
*
*
* @param builtUsing
* The dependencies.
*/
public void setBuiltUsing(List builtUsing) {
this.builtUsing = getList(builtUsing);
}
@Override
public Size getInstalledSize() {
return this.installedSize;
}
/**
*
* Sets an estimate of the total amount of disk space required to install
* this package.
*
*
* @param installedSize
* The installed size.
*/
public void setInstalledSize(Size installedSize) {
this.installedSize = installedSize;
}
@Override
public String getModuleAliases() {
return this.moduleAliases;
}
/**
*
* Sets the module aliases.
*
*
* @param moduleAliases
* The module aliases.
*/
public void setModuleAliases(String moduleAliases) {
this.moduleAliases = moduleAliases;
}
@Override
public Description getDescription() {
return this.description;
}
/**
*
* Sets the description.
*
*
* @param description
* The description.
*/
public void setDescription(Description description) {
this.description = description;
}
}