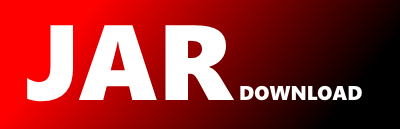
net.sourceforge.javadpkg.impl.CopyrightBuilderImpl Maven / Gradle / Ivy
/*
* dpkg - Debian Package library and the Debian Package Maven plugin
* (c) Copyright 2016 Gerrit Hohl
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU General Public License
* as published by the Free Software Foundation; either version 2
* of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.
*/
package net.sourceforge.javadpkg.impl;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import java.util.Map.Entry;
import net.sourceforge.javadpkg.BuildException;
import net.sourceforge.javadpkg.Context;
import net.sourceforge.javadpkg.Copyright;
import net.sourceforge.javadpkg.CopyrightBuilder;
import net.sourceforge.javadpkg.CopyrightConstants;
import net.sourceforge.javadpkg.CopyrightLicense;
import net.sourceforge.javadpkg.FilesCopyright;
import net.sourceforge.javadpkg.field.Field;
import net.sourceforge.javadpkg.field.FieldBuilder;
import net.sourceforge.javadpkg.field.impl.EmptyField;
import net.sourceforge.javadpkg.field.impl.FieldBuilderImpl;
import net.sourceforge.javadpkg.field.impl.FieldImpl;
import net.sourceforge.javadpkg.io.DataTarget;
/**
*
* A {@link CopyrightBuilder} implementation.
*
*
* @author Gerrit Hohl ([email protected])
* @version 1.0, 04.05.2016 by Gerrit Hohl
*/
public class CopyrightBuilderImpl implements CopyrightBuilder, CopyrightConstants {
/** The builder for the fields. */
private FieldBuilder fieldBuilder;
/**
*
* Creates a builder.
*
*/
public CopyrightBuilderImpl() {
super();
this.fieldBuilder = new FieldBuilderImpl();
}
@Override
public void buildCopyright(Copyright copyright, DataTarget target, Context context) throws IOException, BuildException {
List fields;
if (copyright == null)
throw new IllegalArgumentException("Argument copyright is null.");
if (target == null)
throw new IllegalArgumentException("Argument target is null.");
if (context == null)
throw new IllegalArgumentException("Argument context is null.");
// --- Build the fields ---
fields = this.buildFields(copyright);
// --- Build the copyright ---
this.buildCopyright(fields, target, context);
}
/**
*
* Builds the copyright into the fields.
*
*
* @param copyright
* The copyright.
* @return The fields.
* @throws BuildException
* If an error occurs during the building.
*/
private List buildFields(Copyright copyright) throws BuildException {
List fields;
String format;
format = copyright.getFormat();
if (format == null) {
format = FORMAT_V1_0;
} else if (!FORMAT_V1_0.equals(format))
throw new BuildException("The format of the copyright is |" + format + "|, but only the format |" + FORMAT_V1_0
+ "| is supported by the builder.");
fields = new ArrayList<>();
this.addField(fields, FIELD_FORMAT, format, false);
this.addField(fields, FIELD_UPSTREAM_NAME, copyright.getUpstreamName(), false);
this.addField(fields, FIELD_UPSTREAM_CONTACT, copyright.getUpstreamContact(), false);
this.addField(fields, FIELD_SOURCE, copyright.getSource(), false);
this.addField(fields, FIELD_DISCLAIMER, copyright.getDisclaimer(), false);
this.addField(fields, FIELD_COMMENT, copyright.getComment(), false);
this.addField(fields, FIELD_LICENSE, copyright.getLicense());
this.addField(fields, FIELD_COPYRIGHT, copyright.getCopyright(), false);
for (FilesCopyright files : copyright.getFilesCopyrights()) {
fields.add(EmptyField.EMPTY_FIELD);
this.addField(fields, FIELD_FILES, files.getFiles());
this.addField(fields, FIELD_COPYRIGHT, files.getCopyright(), false);
this.addField(fields, FIELD_LICENSE, files.getLicense());
this.addField(fields, FIELD_COMMENT, files.getComment(), false);
}
for (Entry entry : copyright.getLicenses().entrySet()) {
fields.add(EmptyField.EMPTY_FIELD);
this.addField(fields, FIELD_LICENSE, entry.getValue());
if (entry.getValue().getComment() != null) {
// TODO Add warning as comments are not part of the specification.
this.addField(fields, FIELD_COMMENT, entry.getValue().getComment(), false);
}
}
return fields;
}
/**
*
* Adds a field to the list of fields if a value is available.
*
*
* @param fields
* The fields.
* @param name
* The name.
* @param value
* The value (optional).
* @param formatValue
* The flag if the value should be formatted.
*/
private void addField(List fields, String name, String value, boolean formatValue) {
Field field;
if (value == null)
return;
field = new FieldImpl(name, value, formatValue);
fields.add(field);
}
/**
*
* Adds a field to the list of fields if a value is available.
*
*
* @param fields
* The fields.
* @param name
* The name.
* @param values
* The values (optional).
*/
private void addField(List fields, String name, List values) {
StringBuilder sb;
if ((values == null) || values.isEmpty())
return;
sb = new StringBuilder();
for (String value : values) {
if (sb.length() > 0) {
sb.append('\n');
}
sb.append(value);
}
this.addField(fields, name, sb.toString(), false);
}
/**
*
* Adds a field to the list of fields if a license is available.
*
*
* @param fields
* The fields.
* @param name
* The name.
* @param license
* The license (optional).
*/
private void addField(List fields, String name, CopyrightLicense license) {
StringBuilder sb;
if (license == null)
return;
sb = new StringBuilder();
sb.append(license.getName());
if (license.getText() != null) {
sb.append('\n');
sb.append(license.getText());
}
this.addField(fields, name, sb.toString(), true);
}
/**
*
* Builds the copyright from the fields into the target.
*
*
* @param fields
* The fields.
* @param target
* The target.
* @param context
* The context.
* @throws IOException
* If an I/O error occurs.
* @throws BuildException
* If an error occurs during the building.
*/
private void buildCopyright(List fields, DataTarget target, Context context) throws IOException, BuildException {
try {
this.fieldBuilder.buildFields(fields, target, context);
} catch (IOException e) {
throw new IOException("Couldn't build copyright |" + target.getName() + "|: " + e.getMessage());
} catch (BuildException e) {
throw new BuildException("Couldn't build copyright |" + target.getName() + "|: " + e.getMessage());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy