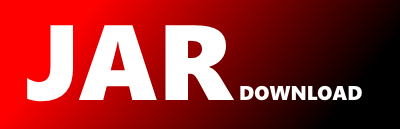
net.sourceforge.javadpkg.io.impl.FileModeImpl Maven / Gradle / Ivy
/*
* dpkg - Debian Package library and the Debian Package Maven plugin
* (c) Copyright 2016 Gerrit Hohl
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU General Public License
* as published by the Free Software Foundation; either version 2
* of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.
*/
package net.sourceforge.javadpkg.io.impl;
import net.sourceforge.javadpkg.io.FileMode;
/**
*
* A {@link FileMode} implementation.
*
*
* @author Gerrit Hohl ([email protected])
* @version 1.0, 12.05.2016 by Gerrit Hohl
*/
public class FileModeImpl implements FileMode {
/** The mode. */
private int mode;
/**
*
* Creates the mode of a file.
*
*
* @param mode
* The mode: A number from octal 0000 (decimal: 0) to octal 7777
* (decimal: 4095).
*/
public FileModeImpl(int mode) {
super();
if ((mode < 00000) || (mode > 07777))
throw new IllegalArgumentException(
"Argument mode is less than octal 000 (decimal: 0) or greater than octal 7777 (decimal: 4095): octal "
+ Integer.toOctalString(mode) + " (decimal: " + mode + ")");
this.mode = mode;
}
@Override
public int getMode() {
return this.mode;
}
@Override
public String getOctal() {
StringBuilder sb;
sb = new StringBuilder();
// --- Ignore sticky bit ---
sb.append(Integer.toOctalString(this.mode & 0777));
while (sb.length() < 3) {
sb.insert(0, '0');
}
return sb.toString();
}
@Override
public String getText() {
StringBuilder sb;
sb = new StringBuilder();
// --- Ignore sticky bit ---
this.addFlag(sb, 'r', this.isOwnerReadable());
this.addFlag(sb, 'w', this.isOwnerWriteable());
this.addFlag(sb, 'x', this.isOwnerExecutable());
this.addFlag(sb, 'r', this.isGroupReadable());
this.addFlag(sb, 'w', this.isGroupWriteable());
this.addFlag(sb, 'x', this.isGroupExecutable());
this.addFlag(sb, 'r', this.isOtherReadable());
this.addFlag(sb, 'w', this.isOtherWriteable());
this.addFlag(sb, 'x', this.isOtherExecutable());
return sb.toString();
}
/**
*
* Adds the flag.
*
*
* @param sb
* The {@link StringBuilder}. The flag will be added to it.
* @param flag
* The flag: "r", "w" or "x".
* @param value
* The value.
*/
private void addFlag(StringBuilder sb, char flag, boolean value) {
if (value) {
sb.append(flag);
} else {
sb.append('-');
}
}
@Override
public int getStickyBit() {
return ((this.mode >> 9) & 00007);
}
@Override
public boolean isOwnerReadable() {
return ((this.mode & 00400) != 0);
}
@Override
public boolean isOwnerWriteable() {
return ((this.mode & 00200) != 0);
}
@Override
public boolean isOwnerExecutable() {
return ((this.mode & 00100) != 0);
}
@Override
public boolean isGroupReadable() {
return ((this.mode & 00040) != 0);
}
@Override
public boolean isGroupWriteable() {
return ((this.mode & 00020) != 0);
}
@Override
public boolean isGroupExecutable() {
return ((this.mode & 00010) != 0);
}
@Override
public boolean isOtherReadable() {
return ((this.mode & 00004) != 0);
}
@Override
public boolean isOtherWriteable() {
return ((this.mode & 00002) != 0);
}
@Override
public boolean isOtherExecutable() {
return ((this.mode & 00001) != 0);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy