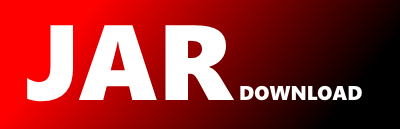
net.sourceforge.javadpkg.store.DataStore Maven / Gradle / Ivy
/*
* dpkg - Debian Package library and the Debian Package Maven plugin
* (c) Copyright 2016 Gerrit Hohl
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU General Public License
* as published by the Free Software Foundation; either version 2
* of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.
*/
package net.sourceforge.javadpkg.store;
import java.io.IOException;
import java.security.MessageDigest;
import java.util.List;
import org.apache.commons.compress.archivers.tar.TarArchiveOutputStream;
import net.sourceforge.javadpkg.control.Size;
import net.sourceforge.javadpkg.io.DataSource;
import net.sourceforge.javadpkg.io.FileMode;
import net.sourceforge.javadpkg.io.FileOwner;
/**
*
* A store for managing the files in the data archive of a Debian package.
*
*
* @author Gerrit Hohl ([email protected])
* @version 1.0, 26.04.2016 by Gerrit Hohl
*/
public interface DataStore {
/**
*
* Adds a directory to the store.
*
*
* This method is equal to the method
* {@link #addDirectory(String, FileOwner, FileMode)} using the following
* values:
*
*
* group ID
* group name
* user ID
* user name
* mode (octal)
*
*
* 0
* root
* 0
* root
* 0755
*
*
*
*
* @param path
* The installation path.
* @throws IllegalArgumentException
* If the installation path is null
, the parent
* directory wasn't added before or already contains an entry
* with the same name. Only exception is the root "/"
* directory which always exists.
*/
void addDirectory(String path);
/**
*
* Adds a directory to the store.
*
*
* @param path
* The installation path.
* @param owner
* The owner.
* @param mode
* The mode.
* @throws IllegalArgumentException
* If any of the parameters are null
, the path
* doesn't contain an valid path, the parent directory wasn't
* added before or already contains an entry with the same name.
* Only exception is the root "/" directory which
* always exists.
*/
void addDirectory(String path, FileOwner owner, FileMode mode);
/**
*
* Adds a file to the store.
*
*
* This method is equal to the method
* {@link #addFile(DataSource, String, FileOwner, FileMode)} using the
* following values:
*
*
* group ID
* group name
* user ID
* user name
* mode (octal)
*
*
* 0
* root
* 0
* root
* 0644
*
*
*
*
* @param source
* The source for the context of the file.
* @param path
* The installation path.
* @throws IllegalArgumentException
* If any of the parameters are null
, the parent
* directory wasn't added before or already contains an entry
* with the same name. Only exception is the root "/"
* directory which always exists.
*/
void addFile(DataSource source, String path);
/**
*
* Adds a file to the store.
*
*
* @param source
* The source for the content of the file.
* @param path
* The installation path.
* @param owner
* The owner.
* @param mode
* The mode.
* @throws IllegalArgumentException
* If any of the parameters are null
, the parent
* directory wasn't added before or already contains an entry
* with the same name. Only exception is the root "/"
* directory which always exists.
*/
void addFile(DataSource source, String path, FileOwner owner, FileMode mode);
/**
*
* Adds a symbolic link to the store.
*
*
* @param path
* The installation path.
* @param target
* The target of the symbolic link.
* @param owner
* The owner.
* @param mode
* The mode.
* @throws IllegalArgumentException
* If any of the parameters are null
, the parent
* directory wasn't added before or already contains an entry
* with the same name. Only exception is the root "/"
* directory which always exists.
*/
void addSymLink(String path, String target, FileOwner owner, FileMode mode);
/**
*
* Returns the flag if a directory or file with the specified path exists.
*
*
* @param path
* The path.
* @return The flag: true
, if the path exists,
* false
otherwise.
* @throws IllegalArgumentException
* If the path is null
.
*/
boolean exists(String path);
/**
*
* Returns the size of all files added to the store.
*
*
* @return The size.
* @throws IOException
* If an I/O error occurs.
*/
Size getSize() throws IOException;
/**
*
* Writes the directories and files added to the store into a TAR archive
* using the specified stream.
*
*
* @param out
* The stream on the TAR archive.
* @throws IllegalArgumentException
* If the stream is null
.
* @throws IOException
* If an I/O error occurs.
*/
void write(TarArchiveOutputStream out) throws IOException;
/**
*
* Creates the hashes for all files in the store using the specified digest.
*
*
* @param digest
* The digest.
* @return The hashes.
* @throws IllegalArgumentException
* If the digest is null
.
* @throws IOException
* If an I/O error occurs.
*/
List createFileHashes(MessageDigest digest) throws IOException;
}