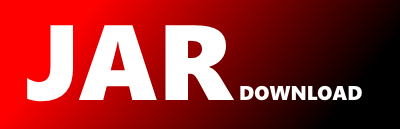
net.sourceforge.jbizmo.commons.selenium.junit.AbstractSeleniumTestCase Maven / Gradle / Ivy
/*
* This file is part of JBizMo, a set of tools, libraries and plug-ins
* for modeling and creating Java-based enterprise applications.
* For more information visit:
*
* http://sourceforge.net/projects/jbizmo/
*
* This software is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 2 of the License, or
* (at your option) any later version.
*
* This software is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this software; if not, write to the Free Software
* Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA
*/
package net.sourceforge.jbizmo.commons.selenium.junit;
import java.util.logging.Level;
import java.util.logging.Logger;
import org.junit.After;
import org.junit.Before;
import org.junit.Test;
/**
*
* Base class for all generated JUnit Selenium tests. This class is responsible for providing a Selenium WebDriver and initializing the test data provider.
*
*
* Copyright 2017 (C) by Martin Ganserer
*
* @author Martin Ganserer
* @version 1.0.0
*/
public abstract class AbstractSeleniumTestCase
{
private static SeleniumTestContext context;
protected Logger logger;
/**
* Create a Selenium WebDriver and initialize the test data provider
*/
@Before
public void setUp()
{
logger = Logger.getLogger(getClass().getName());
// Create the Selenium test context if it hasn't been initialized yet (e.g. by a test suite)
if(context == null)
{
context = SeleniumTestContextFactory.initContext();
// Close the driver after finishing the test!
context.setCloseDriver(true);
}
context.getDataProvider().init(this.getClass());
}
/**
* If applicable, close the driver
*/
@After
public void tearDown()
{
if(context.isCloseDriver())
{
if(logger.isLoggable(Level.FINE))
logger.fine("Close driver");
context.getDriver().quit();
// Clear the context as subsequent tests should use their own!
context = null;
}
}
/**
* Run one test in order to transfer the test context to the implementation method!
*/
@Test
public void test()
{
if(logger.isLoggable(Level.FINE))
logger.fine("Start test");
test(context);
if(logger.isLoggable(Level.FINE))
logger.fine("Finish test");
}
/**
* Every implementation must override this method in order to be able to consume the test context!
* @param context
*/
public abstract void test(SeleniumTestContext context);
/**
* Method to set the Selenium test context if it has been initialized externally (e.g. in a test suite)
* @param context
*/
public static void setContext(SeleniumTestContext context)
{
AbstractSeleniumTestCase.context = context;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy