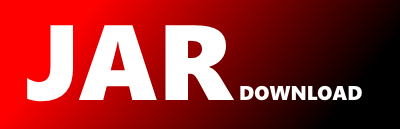
de.mcs.jmeasurement.example.UserDataStore Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of JMeasurement Show documentation
Show all versions of JMeasurement Show documentation
JMeasurement profiling programs in production enviroment
/*
* MCS Media Computer Software
* Copyright (c) 2005 by MCS
* --------------------------------------
* Created on 23.04.2005 by w.klaas
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package de.mcs.jmeasurement.example;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.OutputStream;
import java.io.PrintWriter;
import java.io.Serializable;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Date;
import java.util.Iterator;
import de.mcs.jmeasurement.IUserData;
/**
* This is a Object to store all measured data into an ArrayList. The data will
* be stored with the date, currentMilliSecond and the accrued fields. Then, if
* you want, you can store the data automatically every x data into a file. The
* file will be opend first time when writing data and can be closed any time
* you want. After measuring the file must be closed (with
* UserDataStore.close()). You can also read the measuring with the
* UserDataStore.getData()
*
* @author w.klaas
*/
public class UserDataStore implements IUserData, Serializable {
/**
*
*/
private static final long serialVersionUID = 7121946243997081081L;
/** default buffer size. */
private static final int BUFFER_SIZE = 256;
/**
* Class for holding one measuredata.
*
* @author w.klaas
*/
static class Item implements Serializable {
/**
*
*/
private static final long serialVersionUID = 1L;
/** position to store. */
private long pos;
/** date to store. */
private Date date;
/** msec to store. */
private long msec;
/** accrued to store. */
private long accrued;
/**
* user data store item.
*
* @param position
* position
* @param aAccrued
* accrued
* @param milliseconds
* milliseconds
* @param aDate
* date
*/
public Item(final long position, final long aAccrued, final long milliseconds, final Date aDate) {
this.pos = position;
this.date = aDate;
this.msec = milliseconds;
this.accrued = aAccrued;
}
}
/** data array for the data. */
private ArrayList- store;
/** separator for the dataentries. */
private char sepChar;
/** how many datas must be stored before they will be writing to the file. */
private int writingCount;
/** output stream for writing. */
private transient FileOutputStream fileOut;
/** filename to write to. */
private String filename;
/** position of this data. */
private long actualPosition;
/**
* Constructor for this object.
*
* @param aWritingCount
* if the are writingCount data items in the list, the data will
* be stored to the file. Set this to -1 no data will be stored
* automatically. But you can store the data manually with the
* writeToStream methode.
* @param aFileName
* name of the file to write to (incl. path)
*/
public UserDataStore(final int aWritingCount, final String aFileName) {
if (aWritingCount > 0) {
store = new ArrayList
- (aWritingCount);
} else {
store = new ArrayList
- (1);
}
this.writingCount = aWritingCount;
filename = aFileName;
actualPosition = 0;
}
/**
* writeing all data to the stream and resetting the internal array.
*
* @param stream
* Stream to write
*/
public final void writeToStream(final OutputStream stream) {
PrintWriter printWriter = new PrintWriter(stream);
synchronized (store) {
String[] datas = getData();
for (int i = 0; i < datas.length; i++) {
String string = datas[i];
printWriter.println(string);
}
store.clear();
}
printWriter.flush();
}
/**
* @return Returns the sepChar.
*/
public final char getSepChar() {
return sepChar;
}
/**
* @param aSepChar
* The sepChar to set.
*/
public final void setSepChar(final char aSepChar) {
this.sepChar = aSepChar;
}
/**
* Adding data with the actual datetime.
*
* @param accrued
* the data to add
*/
public final void addData(final long accrued) {
addData(accrued, new Date());
}
/**
* Adding data with defined date.
*
* @param accrued
* data to add
* @param date
* date when to add
*/
public final void addData(final long accrued, final Date date) {
synchronized (store) {
store.add(new Item(actualPosition, accrued, System.currentTimeMillis(), date));
if ((writingCount > 0) && (store.size() >= writingCount)) {
writeToFile();
}
actualPosition++;
}
}
/**
* This methode will write all measure data to the file defined.
*/
private void writeToFile() {
if (null == fileOut) {
try {
if (null != filename) {
fileOut = new FileOutputStream(filename, true);
PrintWriter printWriter = new PrintWriter(fileOut);
printWriter.print("Number");
printWriter.print(sepChar);
printWriter.print("Date and Time");
printWriter.print(sepChar);
printWriter.print("CurrentTimeMillis");
printWriter.print(sepChar);
printWriter.println("Accrued");
printWriter.flush();
}
} catch (FileNotFoundException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
if (null != fileOut) {
writeToStream(fileOut);
}
}
/**
* Writing the last datas and close the file.
*/
public final void closeFile() {
writeToFile();
if (null != fileOut) {
try {
fileOut.flush();
fileOut.close();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
fileOut = null;
}
}
/**
* Getting all data as String array (like the file, but without the header).
*
* @return String[]
*/
public final String[] getData() {
SimpleDateFormat dateFormat = new SimpleDateFormat();
String[] data = null;
synchronized (store) {
data = new String[store.size()];
int count = 0;
for (Iterator
- iter = store.iterator(); iter.hasNext();) {
Item element = iter.next();
StringBuffer dataLine = new StringBuffer(BUFFER_SIZE);
dataLine.append(Long.toString(element.pos));
dataLine.append(sepChar);
if (null != element.date) {
dataLine.append(dateFormat.format(element.date));
} else {
dataLine.append("no date set");
}
dataLine.append(sepChar);
dataLine.append(Long.toString(element.msec));
dataLine.append(sepChar);
dataLine.append(Long.toString(element.accrued));
data[count] = dataLine.toString();
count++;
}
}
return data;
}
/**
* cloning this userdata object.
*
* @return Object
*/
@SuppressWarnings("unchecked")
public final Object clone() {
try {
super.clone();
} catch (CloneNotSupportedException e) {
// e.printStackTrace();
}
UserDataStore udat = new UserDataStore(this.writingCount, this.filename);
udat.actualPosition = this.actualPosition;
udat.sepChar = this.sepChar;
udat.store = (ArrayList
- ) this.store.clone();
return udat;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy