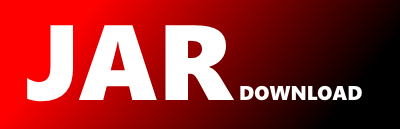
org.qcschema.QCSchemaUnits Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jmol Show documentation
Show all versions of jmol Show documentation
Jmol: an open-source Java viewer for chemical structures in 3D
package org.qcschema;
import java.util.Hashtable;
import java.util.Map;
import java.util.ArrayList;
import org.jmol.viewer.Viewer;
/**
* A general Java class for working with QCShema units and array types.
*
* j2sNative blocks can be ignored -- they just increase efficiency in the JavaScript rendition of Jmol.
*
* for examples of use, see
*
* org.jmol.adapters.readers.quantum.QCJSONReader
*
* org.jmol.adapters.writers.QCJSONWriter extends org.jmol.util.JSONWriter
*
* @author Bob Hanson
*/
public class QCSchemaUnits {
public final static String version = "QCJSON 0-0-0.Jmol_"
+ Viewer.getJmolVersion().replace(' ', '_');
//
// source: http://cccbdb.nist.gov/hartree.asp
// A hartree is equal to 2625.5 kJ/mol, 627.5 kcal/mol, 27.211 eV, and 219474.6 cm-1.
// One bohr = 0.529 177 210 67 x 10-10 m
public final static String UNITS_FRACTIONAL = "fractional";
public final static String UNITS_AU = "au";
public final static double TOAU_AU = 1;
// distance
public final static String UNITS_CM = "cm";
public final static double TOAU_CM = 1/0.52917721067e-8;
public final static String UNITS_M = "m";
public final static double TOAU_M = 1/0.52917721067e-10;
public final static String UNITS_ANGSTROMS = "angstroms";
public final static double TOAU_ANGSTROMS = 1/0.52917721067; // 1.88972613;
public final static String UNITS_BOHR = "bohr";
public final static double TOAU_BOHR = 1;
// energy
public final static String UNITS_HARTREE = "hartree";
public final static double TOAU_HARTREE = 1;
public final static String UNITS_EV = "ev";
public final static double TOAU_EV = 1/27.211; //0.03688675765;
public final static String UNITS_CM_1 = "cm-1";
public final static double TOAU_CM_1 = 1/219474.6; //4.5563359e-6;
public final static String UNITS_KJ_MOL = "kj/mol";
public final static double TOAU_KJ_MOL = 1/2635.5; //0.00038087983;
public final static String UNITS_KCAL_MOL = "kcal/mol";
public final static double TOAU_KCAL_MOL = 1/627.5; //0.00159362549;
/**
* A very simple and efficient way to catalog string matches. Far faster than ENUM.
* Note that singular or plural on anstroms, bohrs, or hartrees both work.
*/
private final static String knownUnits =
/////0 1 2 3 4 5 6 7 8
/////012345678901234567890123456789012345678901234567890123456789012345678901234567890123
"cm cm^-1 cm-1 angstroms au atomic units fractional bohrs hartrees ev kj_mol kcal_mol";
private static Hashtable htConvert = new Hashtable();
/**
* Get the standard conversion factor to atomic units for this unit.
*
* @param units
* @return the nominal conversion factor or 0 ("fractional") or Double.NaN (unknown)
*/
public static double getFactorToAU(String units) {
switch (knownUnits.indexOf(units.toLowerCase())) {
case 0:
// units = UNITS_CM
return TOAU_CM;
case 1:
// units = UNITS_M
return TOAU_M;
case 3:
case 9:
//units = UNITS_CM_1;
return TOAU_CM_1;
case 14:
//units = UNITS_ANGSTROMS;
return TOAU_ANGSTROMS;
case 24:
case 27:
//units = UNITS_AU;
return 1;
case 40:
//units = "UNITS_FRACTIONAL";
return 0;
case 51:
//units = UNITS_BOHR;
return TOAU_BOHR;
case 57:
//units = UNITS_HARTREE;
return TOAU_HARTREE;
case 66:
//units = UNITS_EV;
return TOAU_EV;
case 69:
//units = UNITS_KCAL_MOL;
return TOAU_KCAL_MOL;
case 76:
//units = UNITS_KJ_MOL;
return TOAU_KJ_MOL;
default:
return Double.NaN;
}
}
/**
* Calculate the unit conversion between two units, using a static
* unit-to-unit cache for efficiency.
*
* Not used in Jmol.
*
* @param fromUnits
* @param toUnits
* @return conversion factor or Double.NaN if anything goes wrong.
*/
public static double getUnitConversion(String fromUnits, String toUnits) {
if (fromUnits.equalsIgnoreCase(toUnits))
return 1;
String key = "" + fromUnits + toUnits;
Double d = htConvert.get(key);
if (d != null)
return d.doubleValue();
double val = Double.NaN;
try {
double toAUDesired = getFactorToAU(toUnits);
double toAUActual = getFactorToAU(fromUnits);
val = toAUActual / toAUDesired;
} catch (Exception e) {
// just leave it as 1
}
htConvert.put(key, Double.valueOf(val));
return val;
}
/**
* For a reader, use the JSON [units, factor] along with a desired unit to get the conversion
* factor from file values to desired units.
*
* Currently, this method only looks at the factor in the JSON if we do not already know the conversion factor.
*
* @param unitsFactor [units, factor] list or null if to AU is desired.
* @param unitsDesired
* @return the conversion factor or Double.NaN if not uncodable
*/
public static double getConversionFactorTo(ArrayList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy