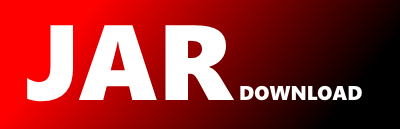
org.jmol.adapter.readers.spartan.SpartanArchive Maven / Gradle / Ivy
/* $RCSfile$
* $Author: hansonr $
* $Date: 2006-07-05 00:45:22 -0500 (Wed, 05 Jul 2006) $
* $Revision: 5271 $
*
* Copyright (C) 2003-2005 Miguel, Jmol Development, www.jmol.org
*
* Contact: [email protected]
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA.
*/
package org.jmol.adapter.readers.spartan;
import org.jmol.adapter.readers.quantum.BasisFunctionReader;
import org.jmol.adapter.smarter.AtomSetCollectionReader;
import org.jmol.adapter.smarter.Bond;
import org.jmol.adapter.smarter.Atom;
import org.jmol.adapter.smarter.SmarterJmolAdapter;
import org.jmol.api.JmolAdapter;
import javajs.util.AU;
import javajs.util.Lst;
import javajs.util.PT;
import java.util.Hashtable;
import java.util.Map;
import org.jmol.quantum.QS;
import org.jmol.util.Logger;
import javajs.util.V3;
class SpartanArchive {
// OK, a bit of a hack.
// It's not a reader, but it needs the capabilities of BasisFunctionReader
private int modelCount = 0;
private int ac = 0;
private String bondData; // not in archive; may or may not have
private int moCount = 0;
private int coefCount = 0;
private int shellCount = 0;
private int gaussianCount = 0;
private String endCheck; // for SMOL or SPARTAN files: "END Directory Entry "
private boolean isSMOL;
private BasisFunctionReader r;
SpartanArchive(BasisFunctionReader r, String bondData, String endCheck) {
initialize(r, bondData);
this.endCheck = endCheck;
isSMOL = (endCheck != null);
}
private void initialize(BasisFunctionReader r, String bondData) {
this.r = r;
r.moData.put("isNormalized", Boolean.TRUE);
r.moData.put("energyUnits","");
this.bondData = bondData;
}
int readArchive(String infoLine, boolean haveGeometryLine,
int ac0, boolean doAddAtoms) throws Exception {
modelAtomCount = setInfo(infoLine);
line = (haveGeometryLine ? "GEOMETRY" : "");
boolean haveMOData = false;
boolean skipping = false;
while (line != null) {
if (line.equals("GEOMETRY")) {
if (!isSMOL && !r.doGetModel(++modelCount, null)) {
readLine();
skipping = true;
continue;
}
skipping = false;
readAtoms(ac0, doAddAtoms);
if (doAddAtoms && bondData.length() > 0)
addBonds(bondData, ac0);
} else if (line.indexOf("BASIS") == 0) {
if (r.doReadMolecularOrbitals) {
readBasis();
} else {
r.discardLinesUntilContains("ENERGY");
line = r.line;
continue;
}
} else if (line.indexOf("WAVEFUNC") == 0 || line.indexOf("BETA") == 0) {
if (r.doReadMolecularOrbitals && !skipping) {
readMolecularOrbital();
haveMOData = true;
} else {
r.discardLinesUntilContains("GEOM");
line = r.line;
}
} else if (line.indexOf("ENERGY") == 0 && !skipping) {
readEnergy();
} else if (line.equals("ENDARCHIVE")
|| isSMOL && line.indexOf(endCheck) == 0) {
break;
}
readLine();
}
if (haveMOData)
r.finalizeMOData(r.moData);
return ac;
}
private void readEnergy() throws Exception {
String[] tokens = PT.getTokens(readLine());
float value = parseFloat(tokens[0]);
r.asc.setCurrentModelInfo("energy", Float.valueOf(value));
if (isSMOL)
((SpartanSmolReader)r).setEnergy(value);
r.asc.setAtomSetEnergy(tokens[0], value);
}
private int modelAtomCount;
private int setInfo(String info) throws Exception {
// 5 17 11 18 0 1 17 0 RHF 3-21G(d) NOOPT FREQ
// 0 1 2 3 4 5 6 7 8 9
String[] tokens = PT.getTokens(info);
if (Logger.debugging) {
Logger.debug("reading Spartan archive info :" + info);
}
modelAtomCount = parseInt(tokens[0]);
coefCount = parseInt(tokens[1]);
shellCount = parseInt(tokens[2]);
gaussianCount = parseInt(tokens[3]);
//overallCharge = parseInt(tokens[4]);
moCount = parseInt(tokens[6]);
r.calculationType = tokens[9];
String s = (String) r.moData.get("calculationType");
if (s == null)
s = r.calculationType;
else if (s.indexOf(r.calculationType) < 0)
s = r.calculationType + s;
r.moData.put("calculationType", r.calculationType = s);
return modelAtomCount;
}
private void readAtoms(int ac0, boolean doAddAtoms) throws Exception {
for (int i = 0; i < modelAtomCount; i++) {
String tokens[] = PT.getTokens(readLine());
Atom atom = (doAddAtoms ? r.asc.addNewAtom()
: r.asc.atoms[ac0 - modelAtomCount + i]);
atom.elementSymbol = AtomSetCollectionReader
.getElementSymbol(parseInt(tokens[0]));
r.setAtomCoordScaled(atom, tokens, 1, AtomSetCollectionReader.ANGSTROMS_PER_BOHR);
}
if (doAddAtoms && Logger.debugging) {
Logger.debug(r.asc.ac + " atoms read");
}
}
void addBonds(String data, int ac0) {
/* from cached data:
1 2 1
1 3 1
1 4 1
1 5 1
1 6 1
1 7 1
*/
String tokens[] = PT.getTokens(data);
for (int i = modelAtomCount; i < tokens.length;) {
int sourceIndex = parseInt(tokens[i++]) - 1 + ac0;
int targetIndex = parseInt(tokens[i++]) - 1 + ac0;
int bondOrder = parseInt(tokens[i++]);
if (bondOrder > 0) {
r.asc.addBond(new Bond(sourceIndex, targetIndex,
bondOrder < 4 ? bondOrder : bondOrder == 5 ? JmolAdapter.ORDER_AROMATIC : 1));
}
}
int bondCount = r.asc.bondCount;
if (Logger.debugging) {
Logger.debug(bondCount + " bonds read");
}
}
/*
* Jmol: XX, YY, ZZ, XY, XZ, YZ
* qchem: dxx, dxy, dyy, dxz, dyz, dzz : VERIFIED
* Jmol: d0, d1+, d1-, d2+, d2-
* qchem: d 1=d2-, d 2=d1-, d 3=d0, d 4=d1+, d 5=d2+
* Jmol: XXX, YYY, ZZZ, XYY, XXY, XXZ, XZZ, YZZ, YYZ, XYZ
* qchem: fxxx, fxxy, fxyy, fyyy, fxxz, fxyz, fyyz, fxzz, fyzz, fzzz
* Jmol: f0, f1+, f1-, f2+, f2-, f3+, f3-
* qchem: f 1=f3-, f 2=f2-, f 3=f1-, f 4=f0, f 5=f1+, f 6=f2+, f 7=f3+
*
*/
//private static String DS_LIST = "d2- d1- d0 d1+ d2+";
//private static String FS_LIST = "f3- f2- f1- f0 f1+ f2+ f3+";
//private static String DC_LIST = "DXX DXY DYY DXZ DYZ DZZ";
//private static String FC_LIST = "XXX XXY XYY YYY XXZ XYZ YYZ XZZ YZZ ZZZ";
void readBasis() throws Exception {
/*
* standard Gaussian format:
BASIS
0 2 1 1 0
0 1 3 1 0
0 3 4 2 0
1 2 7 2 0
1 1 9 2 0
0 3 10 3 0
...
5.4471780000D+00
3.9715132057D-01 0.0000000000D+00 0.0000000000D+00 0.0000000000D+00
8.2454700000D-01
5.5791992333D-01 0.0000000000D+00 0.0000000000D+00 0.0000000000D+00
*/
Lst shells = new Lst();
float[][] gaussians = AU.newFloat2(gaussianCount);
int[] typeArray = new int[gaussianCount];
//if (false) { // checking these still
// r.getDFMap(DC_LIST, JmolAdapter.SHELL_D_CARTESIAN, BasisFunctionReader.CANONICAL_DC_LIST, 3);
// r.getDFMap(FC_LIST, JmolAdapter.SHELL_F_CARTESIAN, BasisFunctionReader.CANONICAL_FC_LIST, 3);
// r.getDFMap(DS_LIST, JmolAdapter.SHELL_D_SPHERICAL, BasisFunctionReader.CANONICAL_DS_LIST, 3);
// r.getDFMap(FS_LIST, JmolAdapter.SHELL_F_SPHERICAL, BasisFunctionReader.CANONICAL_FS_LIST, 3);
// }
for (int i = 0; i < shellCount; i++) {
String[] tokens = PT.getTokens(readLine());
boolean isSpherical = (tokens[4].charAt(0) == '1');
int[] slater = new int[4];
slater[0] = parseInt(tokens[3]) - 1; //atom pointer; 1-based
int iBasis = parseInt(tokens[0]); //0 = S, 1 = SP, 2 = D, 3 = F
switch (iBasis) {
case 0:
iBasis = QS.S;
break;
case 1:
iBasis = (isSpherical ? QS.P : QS.SP);
break;
case 2:
iBasis = (isSpherical ? QS.DS : QS.DC);
break;
case 3:
iBasis = (isSpherical ? QS.FS : QS.FC);
break;
}
slater[1] = iBasis;
int gaussianPtr = slater[2] = parseInt(tokens[2]) - 1;
int nGaussians = slater[3] = parseInt(tokens[1]);
for (int j = 0; j < nGaussians; j++)
typeArray[gaussianPtr + j] = iBasis;
shells.addLast(slater);
}
for (int i = 0; i < gaussianCount; i++) {
float alpha = parseFloat(readLine());
String[] tokens = PT.getTokens(readLine());
int nData = tokens.length;
float[] data = new float[nData + 1];
data[0] = alpha;
//we put D and F into coef 1. This may change if I find that Gaussian output
//lists D and F in columns 3 and 4 as well.
switch (typeArray[i]) {
case QS.S:
data[1] = parseFloat(tokens[0]);
break;
case QS.P:
data[1] = parseFloat(tokens[1]);
break;
case QS.SP:
data[1] = parseFloat(tokens[0]);
data[2] = parseFloat(tokens[1]);
if (data[1] == 0) {
data[1] = data[2];
typeArray[i] = QS.SP;
}
break;
case QS.DC:
case QS.DS:
data[1] = parseFloat(tokens[2]);
break;
case QS.FC:
case QS.FS:
data[1] = parseFloat(tokens[3]);
break;
}
gaussians[i] = data;
}
int nCoeff = 0;
for (int i = 0; i < shellCount; i++) {
int[] slater = shells.get(i);
switch(typeArray[slater[2]]) {
case QS.S:
nCoeff++;
break;
case QS.P:
slater[1] = QS.P;
nCoeff += 3;
break;
case QS.SP:
nCoeff += 4;
break;
case QS.DS:
nCoeff += 5;
break;
case QS.DC:
nCoeff += 6;
break;
case QS.FS:
nCoeff += 7;
break;
case QS.FC:
nCoeff += 10;
break;
}
}
boolean isD5F7 = (nCoeff < coefCount);
if (isD5F7)
for (int i = 0; i < shellCount; i++) {
int[] slater = shells.get(i);
switch (typeArray[i]) {
case QS.DC:
slater[1] = QS.DS;
break;
case QS.FC:
slater[1] = QS.FS;
break;
}
}
r.moData.put("shells", shells);
r.moData.put("gaussians", gaussians);
if (Logger.debugging) {
Logger.debug(shells.size() + " slater shells read");
Logger.debug(gaussians.length + " gaussian primitives read");
}
}
void readMolecularOrbital() throws Exception {
int tokenPt = 0;
r.orbitals = new Lst
© 2015 - 2025 Weber Informatics LLC | Privacy Policy