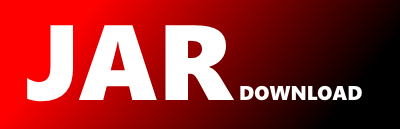
net.sourceforge.kivu4j.utils.json.google.JsonUtilImpl Maven / Gradle / Ivy
/**
* Kivu4j. Copyright (C) 2010 . see
This program is free software: you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation, either version 3 of the License, or
(at your option) any later version.
This program is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with this program. If not, see .
*/
package net.sourceforge.kivu4j.utils.json.google;
import java.io.Reader;
import java.text.DateFormat;
import net.sourceforge.kivu4j.utils.json.JSON;
import net.sourceforge.kivu4j.utils.json.JsonUtil;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
/**
* 使用gson工具实现。
*
*
* 为了方便转换,日期类型转换成为毫秒。
*
*
* @author Lucky Yang
* @see com.google.gson.Gson
*/
public class JsonUtilImpl implements JsonUtil {
private static JsonUtil jsonUtil;
private GsonBuilder gsonBuilder;
private static synchronized void initJsonUtil(){
if (jsonUtil != null) return;
jsonUtil = new JsonUtilImpl();
}
private synchronized void initGsonBuilder(){
if (this.gsonBuilder != null) return;
this.gsonBuilder = new GsonBuilder()
.registerTypeAdapter(java.lang.Object.class, new ObjectSerializer())
.registerTypeAdapter(java.util.Date.class, new UtilDateSerializer())
.registerTypeAdapter(java.util.Date.class, new UtilDateDeserializer())
.setDateFormat(DateFormat.LONG);
}
private Gson createGson(){
if (this.gsonBuilder == null) this.initGsonBuilder();
return this.gsonBuilder.create();
}
/**
*
* @param
* @param gson
* @param s
* @param clazz
* @return
*/
private T fromJson(Gson gson, String s, Class clazz){
if (clazz == null) throw new IllegalArgumentException("clazz");
s = (s == null ?JSON.EMPTY_JSON :s);
return gson.fromJson(s, clazz);
}
/**
*
* @param
* @param gson
* @param reader
* @param clazz
* @return
*/
private T fromJson(Gson gson, Reader reader, Class clazz){
if (clazz == null) throw new IllegalArgumentException("clazz");
if (reader == null){
return this.fromJson(gson, JSON.EMPTY_JSON, clazz);
}
else
return gson.fromJson(reader, clazz);
}
/**
*
* @param gson
* @param o
* @return
*/
private String toJson(Gson gson, Object o){
if (o == null){
return JSON.EMPTY_STRING;
}
else
return gson.toJson(o);
}
@Override
public T fromJson(String s, Class clazz) {
Gson gson = this.createGson();
return this.fromJson(gson, s, clazz);
}
@Override
public String toJson(Object o) {
Gson gson = this.createGson();
return this.toJson(gson, o);
}
@Override
public T fromJson(Reader reader, Class clazz) {
Gson gson = this.createGson();
return this.fromJson(gson, reader, clazz);
}
public static JsonUtil getInstance(){
if (jsonUtil == null) initJsonUtil();
return jsonUtil;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy