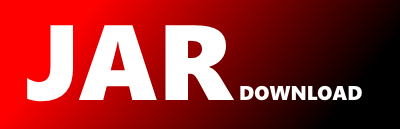
net.sourceforge.lept4j.L_Sudoku Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lept4j Show documentation
Show all versions of lept4j Show documentation
# Lept4J
## Description:
A Java JNA wrapper for Leptonica Image Processing library.
Lept4J is released and distributed under the Apache License, v2.0.
package net.sourceforge.lept4j;
import com.sun.jna.Pointer;
import com.sun.jna.Structure;
import com.sun.jna.ptr.IntByReference;
import java.util.Arrays;
import java.util.List;
/**
* sudoku.h
* The L_Sudoku holds all the information of the current state.
* The input to sudokuCreate() is a file with any number of lines
* starting with '#', followed by 9 lines consisting of 9 numbers
* in each line. These have the known values and use 0 for the unknowns.
* Blank lines are ignored.
* The @locs array holds the indices of the unknowns, numbered
* left-to-right and top-to-bottom from 0 to 80. The array size
* is initialized to @num. @current is the index into the @locs
* array of the current guess: locs[current].
* The @state array is used to determine the validity of each guess.
* It is of size 81, and is initialized by setting the unknowns to 0
* and the knowns to their input values.
* native declaration : sudoku.h:24
* This file was autogenerated by JNAerator,
* a tool written by Olivier Chafik that uses a few opensource projects..
* For help, please visit NativeLibs4Java or JNA.
*/
public class L_Sudoku extends Structure {
/**
* number of unknowns
* C type : l_int32
*/
public int num;
/**
* location of unknowns
* C type : l_int32*
*/
public IntByReference locs;
/**
* index into @locs of current location
* C type : l_int32
*/
public int current;
/**
* initial state, with 0 representing
* C type : l_int32*
*/
public IntByReference init;
/**
* present state, including inits and
* C type : l_int32*
*/
public IntByReference state;
/**
* shows current number of guesses
* C type : l_int32
*/
public int nguess;
/**
* set to 1 when solved
* C type : l_int32
*/
public int finished;
/**
* set to 1 if no solution is possible
* C type : l_int32
*/
public int failure;
public L_Sudoku() {
super();
}
/**
* Gets this Structure's field names in their proper order.
* @return list of ordered field names
*/
@Override
protected List getFieldOrder() {
return Arrays.asList("num", "locs", "current", "init", "state", "nguess", "finished", "failure");
}
/**
* @param num number of unknowns
* C type : l_int32
* @param locs location of unknowns
* C type : l_int32*
* @param current index into @locs of current location
* C type : l_int32
* @param init initial state, with 0 representing
* C type : l_int32*
* @param state present state, including inits and
* C type : l_int32*
* @param nguess shows current number of guesses
* C type : l_int32
* @param finished set to 1 when solved
* C type : l_int32
* @param failure set to 1 if no solution is possible
* C type : l_int32
*/
public L_Sudoku(int num, IntByReference locs, int current, IntByReference init, IntByReference state, int nguess, int finished, int failure) {
super();
this.num = num;
this.locs = locs;
this.current = current;
this.init = init;
this.state = state;
this.nguess = nguess;
this.finished = finished;
this.failure = failure;
}
public L_Sudoku(Pointer peer) {
super(peer);
read();
}
public static class ByReference extends L_Sudoku implements Structure.ByReference {
};
public static class ByValue extends L_Sudoku implements Structure.ByValue {
};
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy