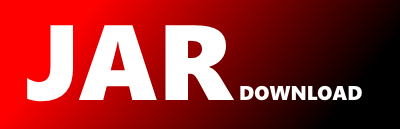
clime.messadmin.utils.ComparableBoolean Maven / Gradle / Ivy
package clime.messadmin.utils;
import java.io.Serializable;
/**
* Comparable Boolean for JDK <= 1.4. Useless for JDK 5.
* Note that some static methods have been removed, as they where already defined in Boolean.
* This class is not intended as a replacement for java.lang.Boolean!
*
* (From the JDK documentation:)
* The Boolean class wraps a value of the primitive type
* boolean
in an object. An object of type
* Boolean
contains a single field whose type is
* boolean
.
*
* In addition, this class provides many methods for
* converting a boolean
to a String
and a
* String
to a boolean
, as well as other
* constants and methods useful when dealing with a
* boolean
.
*
* @author Arthur van Hoff, Cédrik LIME
* @version 1.51, 05/11/04
* @since JDK1.0
*/
public final class ComparableBoolean implements Serializable, Comparable {//extends Boolean
/**
* The ComparableBoolean
object corresponding to the primitive
* value true
.
*/
public static final ComparableBoolean TRUE = new ComparableBoolean(true);
/**
* The ComparableBoolean
object corresponding to the primitive
* value false
.
*/
public static final ComparableBoolean FALSE = new ComparableBoolean(false);
/**
* The Class object representing the primitive type boolean.
*
* @since JDK1.1
*/
public static final Class TYPE = Boolean.TYPE;
/**
* The value of the Boolean.
*
* @serial
*/
private final boolean value;
/** use serialVersionUID from JDK 1.0.2 for interoperability */
//private static final long serialVersionUID = -3665804199014368530L;
/**
* Allocates a ComparableBoolean
object representing the
* value
argument.
*
*
Note: It is rarely appropriate to use this constructor.
* Unless a new instance is required, the static factory
* {@link #valueOf(boolean)} is generally a better choice. It is
* likely to yield significantly better space and time performance.
*
* @param value the value of the Boolean
.
*/
private ComparableBoolean(boolean value) {
this.value = value;
}
/**
* Returns the value of this ComparableBoolean object as a boolean
* primitive.
*
* @return the primitive boolean
value of this object.
*/
public boolean booleanValue() {
return value;
}
/**
* Returns a ComparableBoolean instance representing the specified
* boolean value. If the specified boolean value
* is true, this method returns ComparableBoolean.TRUE;
* if it is false, this method returns ComparableBoolean.FALSE.
* If a new ComparableBoolean instance is not required, this method
* should generally be used in preference to the constructor
* {@link #ComparableBoolean(boolean)}, as this method is likely to yield
* significantly better space and time performance.
*
* @param b a boolean value.
* @return a ComparableBoolean instance representing b.
* @since 1.4
*/
public static ComparableBoolean valueOf(boolean b) {
return (b ? TRUE : FALSE);
}
/**
* Returns a String object representing this ComparableBoolean's
* value. If this object represents the value true
,
* a string equal to "true"
is returned. Otherwise, a
* string equal to "false"
is returned.
*
* @return a string representation of this object.
*/
public String toString() {
return value ? "true" : "false";//Boolean.toString(value);//$NON-NLS-1$//$NON-NLS-2$
}
/**
* Returns a hash code for this ComparableBoolean object.
*
* @return the integer 1231 if this object represents
* true; returns the integer 1237 if this
* object represents false.
*/
public int hashCode() {
return value ? 1231 : 1237;//Boolean.valueOf(value).hashCode();
}
/**
* Returns true
if and only if the argument is not
* null
and is a ComparableBoolean
object that
* represents the same boolean
value as this object.
*
* @param obj the object to compare with.
* @return true
if the Boolean objects represent the
* same value; false
otherwise.
*/
public boolean equals(Object obj) {
if (obj instanceof ComparableBoolean) {
return value == ((ComparableBoolean)obj).booleanValue();
}
if (obj instanceof Boolean) {
return value == ((Boolean)obj).booleanValue();
}
return false;
}
/**
* Compares this ComparableBoolean instance with another.
*
* @param o the ComparableBoolean or Boolean instance to be compared
* @return zero if this object represents the same boolean value as the
* argument; a positive value if this object represents true
* and the argument represents false; and a negative value if
* this object represents false and the argument represents true
* @throws NullPointerException if the argument is null
* @see Comparable
* @since 1.5
*/
public int compareTo(Object o) {
if (o instanceof ComparableBoolean) {
Boolean bool = ((ComparableBoolean)o).value ? Boolean.TRUE : Boolean.FALSE;//Boolean.valueOf(((ComparableBoolean)o).value);
return compareTo(bool);
} else if (o instanceof Boolean) {
return (((Boolean)o).booleanValue() == value ? 0 : (value ? 1 : -1));
}
throw new ClassCastException(o.getClass().getName());
}
}