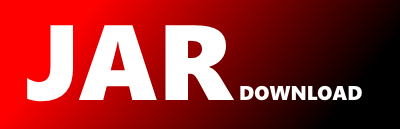
clime.messadmin.utils.SimpleImmutableEntry Maven / Gradle / Ivy
The newest version!
/**
*
*/
package clime.messadmin.utils;
import java.io.Serializable;
import java.util.Map;
/**
* An Entry maintaining an immutable key and value. This class
* does not support method setValue. This class may be
* convenient in methods that return thread-safe snapshots of
* key-value mappings.
*
* @since 1.6
* @author Cédrik LIME
*/
public class SimpleImmutableEntry extends SimpleEntry implements Map.Entry, Serializable {
/**
* Creates an entry representing a mapping from the specified
* key to the specified value.
*
* @param key the key represented by this entry
* @param value the value represented by this entry
*/
public SimpleImmutableEntry(Object key, Object value) {
super(key, value);
}
/**
* Creates an entry representing the same mapping as the
* specified entry.
*
* @param entry the entry to copy
*/
public SimpleImmutableEntry(Map.Entry entry) {
super(entry);
}
/**
* Replaces the value corresponding to this entry with the specified
* value (optional operation). This implementation simply throws
* UnsupportedOperationException, as this class implements
* an immutable map entry.
*
* @param value new value to be stored in this entry
* @return (Does not return)
* @throws UnsupportedOperationException always
*/
public Object setValue(Object value) {
throw new UnsupportedOperationException();
}
}