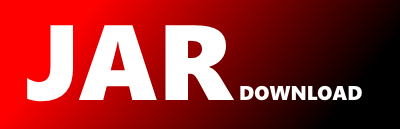
clime.messadmin.utils.StringUtils Maven / Gradle / Ivy
The newest version!
/**
*
*/
package clime.messadmin.utils;
/**
* Copy of org.apache.commons.lang.StringUtils 2.1
* @author Cédrik LIME
*/
public class StringUtils {
private StringUtils() {
}
/**
* Checks if a String is not empty ("") and not null.
*
* @param str the String to check, may be null
* @return true
if the String is not empty or null
*/
public static boolean isEmpty(String str) {
return str == null || str.length() == 0;
}
/**
* Checks if a String is not empty ("") and not null.
*
* @param str the String to check, may be null
* @return true
if the String is not empty and not null
*/
public static boolean isNotEmpty(String str) {
return str != null && str.length() > 0;
}
/**
* Checks if a String is whitespace, empty ("") or null.
*
* @param str the String to check, may be null
* @return true
if the String is null, empty or whitespace
*/
public static boolean isBlank(String str) {
int strLen;
if (str == null || (strLen = str.length()) == 0) {
return true;
}
for (int i = 0; i < strLen; ++i) {
if ( ! Character.isWhitespace(str.charAt(i)) ) {
return false;
}
}
return true;
}
/**
* Checks if a String is not empty (""), not null and not whitespace only.
*
* @param str the String to check, may be null
* @return true
if the String is not empty and not null and not whitespace
*/
public static boolean isNotBlank(String str) {
int strLen;
if (str == null || (strLen = str.length()) == 0) {
return false;
}
for (int i = 0; i < strLen; ++i) {
if ( ! Character.isWhitespace(str.charAt(i)) ) {
return true;
}
}
return false;
}
private static final int HIGHEST_SPECIAL = '>';
private static char[][] specialCharactersRepresentation = new char[HIGHEST_SPECIAL + 1][];
static {
specialCharactersRepresentation['&'] = "&".toCharArray();//$NON-NLS-1$
specialCharactersRepresentation['<'] = "<".toCharArray();//$NON-NLS-1$
specialCharactersRepresentation['>'] = ">".toCharArray();//$NON-NLS-1$
specialCharactersRepresentation['"'] = """.toCharArray();//$NON-NLS-1$
specialCharactersRepresentation['\''] = "'".toCharArray();//$NON-NLS-1$
}
/**
* Copied and adapted from org.apache.taglibs.standard.tag.common.core.Util v1.1.2
*
* Performs the following substring replacements
* (to facilitate output to XML/HTML pages):
*
* & -> &
* < -> <
* > -> >
* " -> "
* ' -> '
*
* See also OutSupport.writeEscapedXml().
*/
public static String escapeXml(String buffer) {
if (null == buffer) {
return null;
}
int start = 0;
int length = buffer.length();
char[] arrayBuffer = buffer.toCharArray();
StringBuffer escapedBuffer = null;
for (int i = 0; i < length; ++i) {
char c = arrayBuffer[i];
if (c <= HIGHEST_SPECIAL) {
char[] escaped = specialCharactersRepresentation[c];
if (escaped != null) {
// create StringBuffer to hold escaped xml string
if (start == 0) {
escapedBuffer = new StringBuffer(length + 5);
}
// add unescaped portion
if (start < i) {
escapedBuffer.append(arrayBuffer,start,i-start);
}
start = i + 1;
// add escaped xml
escapedBuffer.append(escaped);
}
}
}
// no xml escaping was necessary
if (start == 0) {
return buffer;
}
// add rest of unescaped portion
if (start < length) {
escapedBuffer.append(arrayBuffer,start,length-start);
}
return escapedBuffer.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy