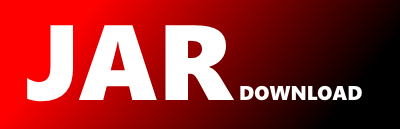
java-fsm.code.net.openai.util.fsm.ComparableCondition Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of openaifsm Show documentation
Show all versions of openaifsm Show documentation
OpenAI FSM is used by Apache cTAKES.
It was originally developed out of sourceforge openAI group
The newest version!
/*****************************************************************************
* net.openai.fsm.ComparableCondition
*****************************************************************************
* @author JC on E
* @date 9/18/2000
* 2001 OpenAI Labs
*****************************************************************************/
package net.openai.util.fsm;
/**
* ComparableCondition class
*/
public final class ComparableCondition extends AbstractCondition {
/** Indicates that this is an "equal to" operation. This is the
default type of ComparableCondition. */
public static final int EQUAL_TO = 0;
/** Indicates that this is a "not equal to" operation. */
public static final int NOT_EQUAL_TO = 1;
/** Indicates that this is a "greater than" operation. */
public static final int GREATER_THAN = 2;
/** Indicates that this is a "less than" operation. */
public static final int LESS_THAN = 3;
/** Indicates that this is a "greater than or equal to" operation. */
public static final int GREATER_THAN_OR_EQUAL_TO = 4;
/** Indicates that this is a "less than or equal to" operation. */
public static final int LESS_THAN_OR_EQUAL_TO = 5;
/** A shorthand for the "equal to" operation. */
public static final int EQ = EQUAL_TO;
/** A shorthand for the "not equal to" operation. */
public static final int NE = NOT_EQUAL_TO;
/** A shorthand for the "greater than" operation. */
public static final int GT = GREATER_THAN;
/** A shorthand for the "less than" operation. */
public static final int LT = LESS_THAN;
/** A shorthand for the "greater than or equal to" operation. */
public static final int GTE = GREATER_THAN_OR_EQUAL_TO;
/** A shorthand for the "less than or equal to" operation. */
public static final int LTE = LESS_THAN_OR_EQUAL_TO;
/** The type of ComparableCondition this instance is. */
private int type = EQUAL_TO;
/** The Comparable object that this instance will be comparing to. */
private Comparable comparable = null;
/**
* Constructs a new ComparableCondition with the default type of
* EQUALS_TO.
*
* @param comparable The Comparable object to compare against.
*/
public ComparableCondition(Comparable comparable) {
createSelf(comparable, type);
}
/**
* Constructs a new ComparableCondition with the default type of
* EQUALS_TO.
*
* @param comparable The Comparable object to compare against.
* @param type The type of comparison to be done.
*/
public ComparableCondition(Comparable comparable, int type) {
createSelf(comparable, type);
}
/**
* Constructs a new ComparableCondition with the default type of
* EQUALS_TO.
*
* @param comparable The Comparable object to compare against.
*/
public ComparableCondition(byte comparable) {
createSelf(new Byte(comparable), type);
}
/**
* Constructs a new ComparableCondition with the default type of
* EQUALS_TO.
*
* @param comparable The Comparable object to compare against.
* @param type The type of comparison to be done.
*/
public ComparableCondition(byte comparable, int type) {
createSelf(new Byte(comparable), type);
}
/**
* Constructs a new ComparableCondition with the default type of
* EQUALS_TO.
*
* @param comparable The Comparable object to compare against.
*/
public ComparableCondition(char comparable) {
createSelf(new Character(comparable), type);
}
/**
* Constructs a new ComparableCondition with the default type of
* EQUALS_TO.
*
* @param comparable The Comparable object to compare against.
* @param type The type of comparison to be done.
*/
public ComparableCondition(char comparable, int type) {
createSelf(new Character(comparable), type);
}
/**
* Constructs a new ComparableCondition with the default type of
* EQUALS_TO.
*
* @param comparable The Comparable object to compare against.
*/
public ComparableCondition(double comparable) {
createSelf(new Double(comparable), type);
}
/**
* Constructs a new ComparableCondition with the default type of
* EQUALS_TO.
*
* @param comparable The Comparable object to compare against.
* @param type The type of comparison to be done.
*/
public ComparableCondition(double comparable, int type) {
createSelf(new Double(comparable), type);
}
/**
* Constructs a new ComparableCondition with the default type of
* EQUALS_TO.
*
* @param comparable The Comparable object to compare against.
*/
public ComparableCondition(float comparable) {
createSelf(new Float(comparable), type);
}
/**
* Constructs a new ComparableCondition with the default type of
* EQUALS_TO.
*
* @param comparable The Comparable object to compare against.
* @param type The type of comparison to be done.
*/
public ComparableCondition(float comparable, int type) {
createSelf(new Float(comparable), type);
}
/**
* Constructs a new ComparableCondition with the default type of
* EQUALS_TO.
*
* @param comparable The Comparable object to compare against.
*/
public ComparableCondition(int comparable) {
createSelf(new Integer(comparable), type);
}
/**
* Constructs a new ComparableCondition with the default type of
* EQUALS_TO.
*
* @param comparable The Comparable object to compare against.
* @param type The type of comparison to be done.
*/
public ComparableCondition(int comparable, int type) {
createSelf(new Integer(comparable), type);
}
/**
* Constructs a new ComparableCondition with the default type of
* EQUALS_TO.
*
* @param comparable The Comparable object to compare against.
*/
public ComparableCondition(long comparable) {
createSelf(new Long(comparable), type);
}
/**
* Constructs a new ComparableCondition with the default type of
* EQUALS_TO.
*
* @param comparable The Comparable object to compare against.
* @param type The type of comparison to be done.
*/
public ComparableCondition(long comparable, int type) {
createSelf(new Long(comparable), type);
}
/**
* Constructs a new ComparableCondition with the default type of
* EQUALS_TO.
*
* @param comparable The Comparable object to compare against.
*/
public ComparableCondition(short comparable) {
createSelf(new Short(comparable), type);
}
/**
* Constructs a new ComparableCondition with the default type of
* EQUALS_TO.
*
* @param comparable The Comparable object to compare against.
* @param type The type of comparison to be done.
*/
public ComparableCondition(short comparable, int type) {
createSelf(new Short(comparable), type);
}
/**
* Constructs a new ComparableCondition with the default type of
* EQUALS_TO.
*
* @param state The target state for this condition.
* @param comparable The Comparable object to compare against.
*/
public ComparableCondition(State state, Comparable comparable) {
super(state);
createSelf(comparable, type);
}
/**
* Constructs a new ComparableCondition with the default type of
* EQUALS_TO.
*
* @param state The target state for this condition.
* @param comparable The Comparable object to compare against.
* @param type The type of comparison to be done.
*/
public ComparableCondition(State state, Comparable comparable, int type) {
super(state);
createSelf(comparable, type);
}
/**
* Constructs a new ComparableCondition with the default type of
* EQUALS_TO.
*
* @param state The target state for this condition.
* @param comparable The Comparable object to compare against.
*/
public ComparableCondition(State state, byte comparable) {
super(state);
createSelf(new Byte(comparable), type);
}
/**
* Constructs a new ComparableCondition with the default type of
* EQUALS_TO.
*
* @param state The target state for this condition.
* @param comparable The Comparable object to compare against.
* @param type The type of comparison to be done.
*/
public ComparableCondition(State state, byte comparable, int type) {
super(state);
createSelf(new Byte(comparable), type);
}
/**
* Constructs a new ComparableCondition with the default type of
* EQUALS_TO.
*
* @param state The target state for this condition.
* @param comparable The Comparable object to compare against.
*/
public ComparableCondition(State state, char comparable) {
super(state);
createSelf(new Character(comparable), type);
}
/**
* Constructs a new ComparableCondition with the default type of
* EQUALS_TO.
*
* @param state The target state for this condition.
* @param comparable The Comparable object to compare against.
* @param type The type of comparison to be done.
*/
public ComparableCondition(State state, char comparable, int type) {
super(state);
createSelf(new Character(comparable), type);
}
/**
* Constructs a new ComparableCondition with the default type of
* EQUALS_TO.
*
* @param state The target state for this condition.
* @param comparable The Comparable object to compare against.
*/
public ComparableCondition(State state, double comparable) {
super(state);
createSelf(new Double(comparable), type);
}
/**
* Constructs a new ComparableCondition with the default type of
* EQUALS_TO.
*
* @param state The target state for this condition.
* @param comparable The Comparable object to compare against.
* @param type The type of comparison to be done.
*/
public ComparableCondition(State state, double comparable, int type) {
super(state);
createSelf(new Double(comparable), type);
}
/**
* Constructs a new ComparableCondition with the default type of
* EQUALS_TO.
*
* @param state The target state for this condition.
* @param comparable The Comparable object to compare against.
*/
public ComparableCondition(State state, float comparable) {
super(state);
createSelf(new Float(comparable), type);
}
/**
* Constructs a new ComparableCondition with the default type of
* EQUALS_TO.
*
* @param state The target state for this condition.
* @param comparable The Comparable object to compare against.
* @param type The type of comparison to be done.
*/
public ComparableCondition(State state, float comparable, int type) {
super(state);
createSelf(new Float(comparable), type);
}
/**
* Constructs a new ComparableCondition with the default type of
* EQUALS_TO.
*
* @param state The target state for this condition.
* @param comparable The Comparable object to compare against.
*/
public ComparableCondition(State state, int comparable) {
super(state);
createSelf(new Integer(comparable), type);
}
/**
* Constructs a new ComparableCondition with the default type of
* EQUALS_TO.
*
* @param comparable The Comparable object to compare against.
* @param type The type of comparison to be done.
*/
public ComparableCondition(State state, int comparable, int type) {
super(state);
createSelf(new Integer(comparable), type);
}
/**
* Constructs a new ComparableCondition with the default type of
* EQUALS_TO.
*
* @param state The target state for this condition.
* @param comparable The Comparable object to compare against.
*/
public ComparableCondition(State state, long comparable) {
super(state);
createSelf(new Long(comparable), type);
}
/**
* Constructs a new ComparableCondition with the default type of
* EQUALS_TO.
*
* @param state The target state for this condition.
* @param comparable The Comparable object to compare against.
* @param type The type of comparison to be done.
*/
public ComparableCondition(State state, long comparable, int type) {
super(state);
createSelf(new Long(comparable), type);
}
/**
* Constructs a new ComparableCondition with the default type of
* EQUALS_TO.
*
* @param state The target state for this condition.
* @param comparable The Comparable object to compare against.
*/
public ComparableCondition(State state, short comparable) {
super(state);
createSelf(new Short(comparable), type);
}
/**
* Constructs a new ComparableCondition with the default type of
* EQUALS_TO.
*
* @param state The target state for this condition.
* @param comparable The Comparable object to compare against.
* @param type The type of comparison to be done.
*/
public ComparableCondition(State state, short comparable, int type) {
super(state);
createSelf(new Short(comparable), type);
}
/**
* Does the actual creation of a ComparableCondition.
*
* @param comparable The Comparable object to compare against.
* @param type The type of comparison to be done.
*/
private final void createSelf(Comparable comparable, int type) {
if(comparable == null)
throw new NullPointerException("null Comparable");
this.comparable = comparable;
this.type = type;
}
/**
* Sets the type of ComparableCondition this is.
*
* @param type The new type of this condition.
*/
public final void setType(int type) {
if((type < EQUAL_TO) ||
(type > LESS_THAN_OR_EQUAL_TO))
throw new IndexOutOfBoundsException("Type is invalid: " + type);
this.type = type;
}
/**
* Returns the type of ComparableCondition this is.
*
* @return An int representation of the type of ComparableCondition this
* is.
*/
public final int getType() {
return type;
}
/**
* Implemented method for the Condition interface.
*
* Called to check if the conditional meets the criteria defined by this
* state. This is the only method to implement in order to used this
* interface.
*
* @param conditional The object to check.
* @return Implementors should return true
if this condition
* is met by the Object conditional
and
* false
otherwise.
*/
public final boolean satisfiedBy(Object conditional) {
if(conditional == null)
return false;
if(conditional instanceof Comparable) {
Comparable cond = (Comparable)conditional;
int checkType = type;
switch(checkType) {
case EQ:
return (cond.compareTo(comparable) == 0);
case NE:
return (cond.compareTo(comparable) != 0);
case GT:
return (cond.compareTo(comparable) > 0);
case LT:
return (cond.compareTo(comparable) < 0);
case GTE:
return (cond.compareTo(comparable) >= 0);
case LTE:
return (cond.compareTo(comparable) <= 0);
default:
System.err.println("ComparableCondition.satisfiedBy: WARNING" +
": invalid type: " + checkType);
}
}
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy