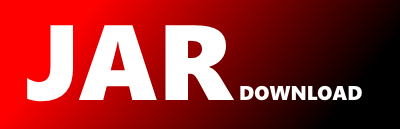
it.openutils.migration.generic.JdbcColumnBasedConditionalTask Maven / Gradle / Ivy
/*
* Copyright Openmind http://www.openmindonline.it
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package it.openutils.migration.generic;
import it.openutils.migration.task.setup.BaseConditionalTask;
import java.sql.Connection;
import java.sql.DatabaseMetaData;
import java.sql.ResultSet;
import java.sql.ResultSetMetaData;
import java.sql.SQLException;
import java.util.HashMap;
import java.util.Map;
import org.apache.commons.lang.StringUtils;
import org.springframework.dao.DataAccessException;
import org.springframework.jdbc.core.ConnectionCallback;
import org.springframework.jdbc.core.simple.SimpleJdbcTemplate;
/**
* Base conditional task that operates on conditions related to a specific column. This base task takes care of
* retrieving the column metadata, so that subclasses only need to override checkColumnMetadata()
.
* @author fgiust
* @version $Id: JdbcColumnBasedConditionalTask.java 837 2008-06-11 21:25:54Z fgiust $
*/
public abstract class JdbcColumnBasedConditionalTask extends BaseConditionalTask
{
/**
* Column name
*/
protected String column;
/**
* Catalog name
*/
protected String catalog;
/**
* Schema name
*/
protected String schema;
/**
* Sets the catalog.
* @param catalog the catalog to set
*/
public void setCatalog(String catalog)
{
this.catalog = catalog;
}
/**
* Sets the schema.
* @param schema the schema to set
*/
public void setSchema(String schema)
{
this.schema = schema;
}
/**
* Sets the column.
* @param column the column to set
*/
public void setColumn(String column)
{
this.column = column;
}
/**
* {@inheritDoc}
*/
@Override
public boolean check(SimpleJdbcTemplate jdbcTemplate)
{
String columnTrim = StringUtils.trim(column);
final String tableName = StringUtils.substringBefore(columnTrim, ".");
final String columnName = StringUtils.substringAfter(columnTrim, ".");
return (Boolean) jdbcTemplate.getJdbcOperations().execute(new ConnectionCallback()
{
public Object doInConnection(Connection con) throws SQLException, DataAccessException
{
DatabaseMetaData dbMetadata = con.getMetaData();
ResultSet rs = dbMetadata.getColumns(catalog, schema, tableName, columnName);
boolean conditionMet = rs.next();
if (conditionMet)
{
ResultSetMetaData rsmeta = rs.getMetaData();
int colcount = rsmeta.getColumnCount();
Map params = new HashMap();
for (int j = 1; j <= colcount; j++)
{
params.put(rsmeta.getColumnName(j), rs.getObject(j));
}
conditionMet = checkColumnMetadata(params);
}
rs.close();
return conditionMet;
}
});
}
/**
*
* Check if a specific condition is met depending on column metadata.
*
* Column attributes included in the Map are:
*
* - TABLE_CAT String => table catalog (may be
null
)
* - TABLE_SCHEM String => table schema (may be
null
)
* - TABLE_NAME String => table name
* - COLUMN_NAME String => column name
* - DATA_TYPE int => SQL type from java.sql.Types
* - TYPE_NAME String => Data source dependent type name, for a UDT the type name is fully
* qualified
* - COLUMN_SIZE int => column size.
* - BUFFER_LENGTH is not used.
* - DECIMAL_DIGITS int => the number of fractional digits. Null is returned for data types
* where DECIMAL_DIGITS is not applicable.
* - NUM_PREC_RADIX int => Radix (typically either 10 or 2)
* - NULLABLE int => is NULL allowed.
*
* - columnNoNulls - might not allow
NULL
values
* - columnNullable - definitely allows
NULL
values
* - columnNullableUnknown - nullability unknown
*
* - REMARKS String => comment describing column (may be
null
)
* - COLUMN_DEF String => default value for the column, which should be interpreted as a string
* when the value is enclosed in single quotes (may be
null
)
* - SQL_DATA_TYPE int => unused
* - SQL_DATETIME_SUB int => unused
* - CHAR_OCTET_LENGTH int => for char types the maximum number of bytes in the column
* - ORDINAL_POSITION int => index of column in table (starting at 1)
* - IS_NULLABLE String => ISO rules are used to determine the nullability for a column.
*
* - YES --- if the parameter can include NULLs
* - NO --- if the parameter cannot include NULLs
* - empty string --- if the nullability for the parameter is unknown
*
* - SCOPE_CATLOG String => catalog of table that is the scope of a reference attribute (
null
* if DATA_TYPE isn't REF)
* - SCOPE_SCHEMA String => schema of table that is the scope of a reference attribute (
null
* if the DATA_TYPE isn't REF)
* - SCOPE_TABLE String => table name that this the scope of a reference attribure (
null
* if the DATA_TYPE isn't REF)
* - SOURCE_DATA_TYPE short => source type of a distinct type or user-generated Ref type, SQL
* type from java.sql.Types (
null
if DATA_TYPE isn't DISTINCT or user-generated REF)
* - IS_AUTOINCREMENT String => Indicates whether this column is auto incremented
*
* - YES --- if the column is auto incremented
* - NO --- if the column is not auto incremented
* - empty string --- if it cannot be determined whether the column is auto incremented parameter is unknown
*
*
* @param metadata column metadata
* @return true
if the condition is met
*/
protected abstract boolean checkColumnMetadata(Map metadata);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy