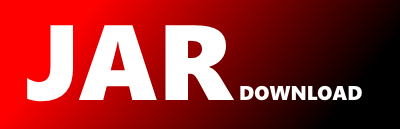
net.sourceforge.openutils.mgnlmessages.i18n.RepositoryMessagesImpl Maven / Gradle / Ivy
package net.sourceforge.openutils.mgnlmessages.i18n;
import info.magnolia.cms.core.Content;
import info.magnolia.cms.core.HierarchyManager;
import info.magnolia.cms.core.ItemType;
import info.magnolia.cms.core.search.Query;
import info.magnolia.cms.core.search.QueryManager;
import info.magnolia.cms.core.search.QueryResult;
import info.magnolia.cms.i18n.AbstractMessagesImpl;
import info.magnolia.cms.util.NodeDataUtil;
import info.magnolia.context.MgnlContext;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Iterator;
import java.util.List;
import java.util.Locale;
import java.util.MissingResourceException;
import javax.jcr.RepositoryException;
import org.apache.commons.lang.StringUtils;
/**
* @author molaschi
* @version $Id: RepositoryMessagesImpl.java 4465 2008-09-28 10:59:58Z fgiust $
*/
public class RepositoryMessagesImpl extends AbstractMessagesImpl
{
private static final String MESS_REPO = "messages";
private List keys;
/**
* @param basename
* @param locale
*/
public RepositoryMessagesImpl(String basename, Locale locale)
{
super(basename, locale);
}
/**
* Get the message from the bundle
* @param key the key
* @return message
*/
public String get(String key)
{
if (key == null)
{
return "??????";
}
try
{
String handle = StringUtils.replace(key, ".", "/");
HierarchyManager hm = MgnlContext.getSystemContext().getHierarchyManager(MESS_REPO);
Content c = hm.getContent(handle);
String locale1 = this.locale.getLanguage() + "_" + this.locale.getCountry();
String locale2 = this.locale.getLanguage();
if (c == null || (!c.hasNodeData(locale1) && !c.hasNodeData(locale2)))
{
return "???" + key + "???";
}
if (c.hasNodeData(locale1))
{
return NodeDataUtil.getString(c, locale1);
}
else
{
return NodeDataUtil.getString(c, locale2);
}
}
catch (MissingResourceException e)
{
return "???" + key + "???";
}
catch (RepositoryException e)
{
return "???" + key + "???";
}
}
/**
* {@inheritDoc}
*/
public void reload() throws Exception
{
keys = null;
}
/**
* {@inheritDoc}
*/
@SuppressWarnings("unchecked")
public Iterator keys()
{
long ms = System.currentTimeMillis();
if (keys == null)
{
keys = new ArrayList();
try
{
QueryManager qm = MgnlContext.getSystemContext().getQueryManager(MESS_REPO);
Query q = qm.createQuery("//*", Query.XPATH);
QueryResult qr = q.execute();
for (Content c : (Collection) qr.getContent(ItemType.CONTENTNODE.getSystemName()))
{
if (c.getNodeDataCollection().size() > 0)
{
keys.add(StringUtils.replace(c.getHandle(), "/", ".").substring(1));
}
}
}
catch (RepositoryException e)
{
}
}
log.debug("Messages loaded in {} ms", System.currentTimeMillis() - ms);
return keys.iterator();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy