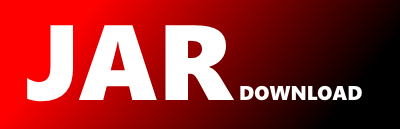
net.sourceforge.openutils.mgnlmessages.pages.ExtractMessagesFromDialogsPage Maven / Gradle / Ivy
package net.sourceforge.openutils.mgnlmessages.pages;
import info.magnolia.cms.beans.config.ContentRepository;
import info.magnolia.cms.core.Content;
import info.magnolia.cms.core.HierarchyManager;
import info.magnolia.cms.core.ItemType;
import info.magnolia.cms.core.NodeData;
import info.magnolia.cms.core.search.Query;
import info.magnolia.cms.core.search.QueryManager;
import info.magnolia.cms.core.search.QueryResult;
import info.magnolia.cms.util.ContentUtil;
import info.magnolia.cms.util.NodeDataUtil;
import info.magnolia.context.MgnlContext;
import info.magnolia.module.admininterface.TemplatedMVCHandler;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import javax.jcr.RepositoryException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import net.sourceforge.openutils.mgnlmessages.lifecycle.MessagesModuleLifecycle;
import org.apache.commons.lang.StringUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* @author molaschi
* @version $Id: $
*/
public class ExtractMessagesFromDialogsPage extends TemplatedMVCHandler
{
private List dialogsRoot;
/**
* Logger.
*/
private Logger log = LoggerFactory.getLogger(ExtractMessagesFromDialogsPage.class);
/**
* @param name
* @param request
* @param response
*/
public ExtractMessagesFromDialogsPage(String name, HttpServletRequest request, HttpServletResponse response)
{
super(name, request, response);
}
/**
* {@inheritDoc}
*/
@SuppressWarnings("unchecked")
@Override
public String show()
{
dialogsRoot = new ArrayList();
QueryManager qm = MgnlContext.getQueryManager(ContentRepository.CONFIG);
Query q;
try
{
q = qm.createQuery("//dialogs", Query.XPATH);
QueryResult qr = q.execute();
Collection dialogs = qr.getContent();
for (Content dialog : dialogs)
{
dialogsRoot.add(dialog.getHandle());
}
q = qm.createQuery("//paragraphs", Query.XPATH);
qr = q.execute();
dialogs = qr.getContent();
for (Content dialog : dialogs)
{
dialogsRoot.add(dialog.getHandle());
}
}
catch (RepositoryException e)
{
// ignore
}
return super.show();
}
/**
* Extract messages keys
* @return view
*/
public String extract()
{
HierarchyManager hmConfig = MgnlContext.getHierarchyManager(ContentRepository.CONFIG);
QueryManager qm = hmConfig.getQueryManager();
HierarchyManager hm = MgnlContext.getHierarchyManager(MessagesModuleLifecycle.REPO);
for (String dialogRoot : this.request.getParameterValues("dialogsRoots"))
{
try
{
doExtraction(dialogRoot, "label", qm, hm, hmConfig);
}
catch (RepositoryException e)
{
log.error("Error extracting labels from dialogs and paragraphs", e);
}
try
{
doExtraction(dialogRoot, "description", qm, hm, hmConfig);
}
catch (RepositoryException e)
{
log.error("Error extracting description from dialogs and paragraphs", e);
}
try
{
doExtraction(dialogRoot, "title", qm, hm, hmConfig);
}
catch (RepositoryException e)
{
log.error("Error extracting description from dialogs and paragraphs", e);
}
}
return this.show();
}
@SuppressWarnings("unchecked")
private void doExtraction(String root, String property, QueryManager qm, HierarchyManager hmMessages,
HierarchyManager hmConfig) throws RepositoryException
{
Query q = qm.createQuery(root.substring(1) + "//*[@" + property + "]", Query.XPATH);
QueryResult qr = q.execute();
Collection labelParents = new ArrayList();
labelParents.addAll(qr.getContent(ItemType.CONTENTNODE.getSystemName()));
labelParents.addAll(qr.getContent(ItemType.CONTENT.getSystemName()));
for (Content labelParent : labelParents)
{
NodeData labelNd = labelParent.getNodeData(property);
String label = labelNd.getString();
if (!StringUtils.isEmpty(label) && MgnlContext.getMessages().get(label).startsWith("???"))
{
String parentPath = labelParent.getHandle();
parentPath = StringUtils.replace(parentPath, "/modules", "");
Content parent = getOrCreateFullPath(hmMessages, parentPath);
Content message = ContentUtil.getOrCreateContent(parent, property, ItemType.CONTENTNODE);
NodeData nd = NodeDataUtil.getOrCreate(message, "en");
nd.setValue(label);
nd = NodeDataUtil.getOrCreate(message, "it");
nd.setValue(label);
String messageKey = message.getHandle().substring(1);
messageKey = StringUtils.replace(messageKey, "/", ".");
labelNd.setValue(messageKey);
}
}
hmMessages.save();
hmConfig.save();
}
private Content getOrCreateFullPath(HierarchyManager mgr, String path) throws RepositoryException
{
try
{
return mgr.getContent(path);
}
catch (RepositoryException ex)
{
String parent = StringUtils.substringBeforeLast(path, "/");
String label = StringUtils.substringAfterLast(path, "/");
if (!StringUtils.isEmpty(parent))
{
getOrCreateFullPath(mgr, parent);
}
else
{
parent = "/";
}
Content c = mgr.createContent(parent, label, ItemType.CONTENTNODE.getSystemName());
mgr.save();
return c;
}
}
/**
* Returns the dialogsRoot.
* @return the dialogsRoot
*/
public List getDialogsRoot()
{
return dialogsRoot;
}
/**
* Sets the dialogsRoot.
* @param dialogsRoot the dialogsRoot to set
*/
public void setDialogsRoot(List dialogsRoot)
{
this.dialogsRoot = dialogsRoot;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy