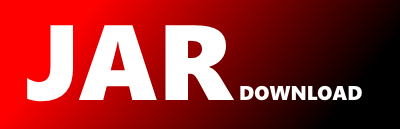
it.openutils.mgnlspring.WrappedResponse Maven / Gradle / Ivy
/*
* Copyright 2007 Fabrizio Giustina.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package it.openutils.mgnlspring;
import java.io.ByteArrayOutputStream;
import java.io.CharArrayWriter;
import java.io.IOException;
import java.io.PrintWriter;
import javax.servlet.ServletOutputStream;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpServletResponseWrapper;
/**
* @author fgiust
* @version $Id: WrappedResponse.java 344 2007-06-30 15:31:28Z fgiust $
*/
public class WrappedResponse extends HttpServletResponseWrapper
{
/**
* The buffered response.
*/
private CharArrayWriter outputWriter;
/**
* The outputWriter stream.
*/
private SimpleServletOutputStream servletOutputStream;
/**
* @param httpServletResponse the response to wrap
*/
public WrappedResponse(HttpServletResponse httpServletResponse)
{
super(httpServletResponse);
this.outputWriter = new CharArrayWriter();
this.servletOutputStream = new SimpleServletOutputStream();
}
/**
* {@inheritDoc}
*/
@Override
public PrintWriter getWriter() throws IOException
{
return new PrintWriter(this.outputWriter);
}
/**
* Flush the buffer, not the response.
* @throws IOException if encountered when flushing
*/
@Override
public void flushBuffer() throws IOException
{
if (outputWriter != null)
{
this.outputWriter.flush();
this.servletOutputStream.outputStream.reset();
}
}
/**
* {@inheritDoc}
*/
@Override
public ServletOutputStream getOutputStream() throws IOException
{
return this.servletOutputStream;
}
/**
* @return buffered response
*/
public char[] getContent()
{
return this.outputWriter.toCharArray();
}
class SimpleServletOutputStream extends ServletOutputStream
{
/**
* My outputWriter stream, a buffer.
*/
ByteArrayOutputStream outputStream = new ByteArrayOutputStream();
/**
* {@inheritDoc}
*/
@Override
public void write(int b)
{
this.outputStream.write(b);
}
/**
* {@inheritDoc} Get the contents of the outputStream.
* @return contents of the outputStream
*/
@Override
public String toString()
{
return this.outputStream.toString();
}
/**
* Reset the wrapped ByteArrayOutputStream.
*/
public void reset()
{
outputStream.reset();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy