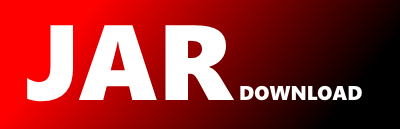
it.openutils.spring.email.TemplatedMailSenderImpl Maven / Gradle / Ivy
The newest version!
/*
* Copyright Openmind http://www.openmindonline.it
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package it.openutils.spring.email;
import freemarker.template.Configuration;
import freemarker.template.Template;
import freemarker.template.TemplateException;
import java.io.File;
import java.io.IOException;
import java.util.List;
import java.util.Map;
import javax.mail.internet.MimeMessage;
import org.apache.commons.lang.ArrayUtils;
import org.apache.commons.lang.StringUtils;
import org.apache.commons.lang.exception.NestableRuntimeException;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.core.io.FileSystemResource;
import org.springframework.core.io.InputStreamSource;
import org.springframework.mail.MailException;
import org.springframework.mail.javamail.JavaMailSender;
import org.springframework.mail.javamail.MimeMessageHelper;
import org.springframework.mail.javamail.MimeMessagePreparator;
import org.springframework.ui.freemarker.FreeMarkerTemplateUtils;
/**
* @author fgiust
* @version $Id: TemplatedMailSenderImpl.java 527 2008-01-14 12:01:09Z fgiust $
*/
public class TemplatedMailSenderImpl implements TemplatedMailSender
{
/**
* Logger.
*/
private Logger log = LoggerFactory.getLogger(TemplatedMailSenderImpl.class);
/**
* Spring mail sender.
*/
private JavaMailSender mailSender;
private Configuration freemarkerConfiguration;
private MessageStore store;
/**
* Allow async delivery.
*/
private boolean allowAsync;
/**
* Sets the mailSender.
* @param mailSender the mailSender to set
*/
public void setMailSender(JavaMailSender mailSender)
{
this.mailSender = mailSender;
}
/**
* Sets the freemarkerConfiguration.
* @param freemarkerConfiguration the freemarkerConfiguration to set
*/
public void setFreemarkerConfiguration(Configuration freemarkerConfiguration)
{
this.freemarkerConfiguration = freemarkerConfiguration;
}
/**
* Sets the allowAsync.
* @param allowAsync the allowAsync to set
*/
public void setAllowAsync(boolean allowAsync)
{
this.allowAsync = allowAsync;
}
/**
* Sets the store.
* @param store the store to set
*/
public void setStore(MessageStore store)
{
this.store = store;
}
/**
* {@inheritDoc}
*/
public void prepareAndSendMail(final String template, final Map model, final String from,
final String[] to, final String[] cc, final String[] bcc, final String replyto, boolean async,
final List attachments)
{
String errorMessageTmp = "Error sending mail to " + ArrayUtils.toString(to);
String infoMsg = "Mail is sent to " + ArrayUtils.toString(to);
String infoPrepareMsg = "Preparing to send mail to " + ArrayUtils.toString(to);
StringBuffer infoBuf = new StringBuffer();
StringBuffer prepareBuf = new StringBuffer();
final String infoMessage = infoMsg + infoBuf.toString();
final String infoPrepareMessage = infoPrepareMsg + prepareBuf.toString();
final String errorMessage = errorMessageTmp;
if (StringUtils.isEmpty(from))
{
log.error(errorMessage + "sender not set", new Exception("Stacktrace added for debug only"));
return;
}
log.info(infoPrepareMessage);
Thread sendmailThread = new Thread()
{
@Override
public void run()
{
MimeMessagePreparator preparator = new MimeMessagePreparator()
{
public void prepare(MimeMessage mimeMessage) throws Exception
{
MimeMessageHelper message = new MimeMessageHelper(mimeMessage, attachments != null
&& !attachments.isEmpty());
message.setFrom(from);
message.setTo(to);
if (StringUtils.isNotEmpty(replyto))
{
message.setReplyTo(replyto);
}
if (cc != null && cc.length > 0)
{
message.setCc(cc);
}
if (bcc != null && bcc.length > 0)
{
message.setBcc(bcc);
}
String text;
try
{
Template freemarkerTemplate = freemarkerConfiguration.getTemplate(template, "UTF-8");
text = FreeMarkerTemplateUtils.processTemplateIntoString(freemarkerTemplate, model);
}
catch (IOException e)
{
throw new NestableRuntimeException(e);
}
catch (TemplateException e)
{
throw new NestableRuntimeException(e);
}
String subject = StringUtils.trim(StringUtils.substringBefore(text, "\n"));
message.setSubject(subject);
message.setText(StringUtils.substringAfter(text, "\n"), true);
if (attachments != null && !attachments.isEmpty())
{
for (File file : attachments)
{
InputStreamSource attachment = new FileSystemResource(file);
message.addAttachment(file.getName(), attachment);
}
}
if (store != null)
{
store.saveMessage(mimeMessage, template);
}
}
};
try
{
mailSender.send(preparator);
log.info(infoMessage);
}
catch (MailException me)
{
log.error(errorMessage, me);
throw me;
}
}
};
if (async && allowAsync)
{
sendmailThread.start();
}
else
{
sendmailThread.run();
}
}
}