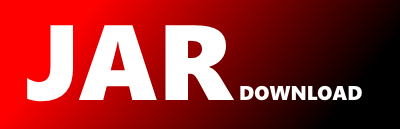
it.openutils.web.spring.ParamNameUrlHandlerMapping Maven / Gradle / Ivy
/*
* Copyright Openmind http://www.openmindonline.it
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package it.openutils.web.spring;
import java.util.HashMap;
import java.util.Map;
import java.util.Properties;
import javax.servlet.http.HttpServletRequest;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.BeansException;
import org.springframework.web.servlet.handler.AbstractUrlHandlerMapping;
/**
* Implementation of the HandlerMapping interface to map from URLs to request handler beans.
* @author Fabrizio Giustina
*/
public class ParamNameUrlHandlerMapping extends AbstractUrlHandlerMapping
{
/**
* Logger.
*/
private static Logger log = LoggerFactory.getLogger(ParamNameUrlHandlerMapping.class);
/**
* Map
*/
private final Map urlMap = new HashMap();
/**
* Name of the param mapped to the controller.
*/
private String paramName;
/**
* Set a Map with URL paths as keys and handler beans as values. Convenient for population with bean references.
*
* Supports direct URL matches and Ant-style pattern matches. For syntax details, see the PathMatcher class.
* @param urlMap map with URLs as keys and beans as values
* @see org.springframework.util.PathMatcher
*/
public void setUrlMap(Map urlMap)
{
this.urlMap.putAll(urlMap);
}
/**
* set the parameter name
* @param paramName
*/
public void setParamName(String paramName)
{
this.paramName = paramName;
}
/**
* Map URL paths to handler bean names. This the typical way of configuring this HandlerMapping.
*
* Supports direct URL matches and Ant-style pattern matches. For syntax details, see the PathMatcher class.
* @param mappings properties with URLs as keys and bean names as values
* @see org.springframework.util.PathMatcher
*/
@SuppressWarnings("unchecked")
public void setMappings(Properties mappings)
{
((Map) urlMap).putAll(mappings);
}
/**
* init the application context
* @see org.springframework.context.support.ApplicationObjectSupport#initApplicationContext()
*/
@Override
public void initApplicationContext() throws BeansException
{
if (this.paramName == null)
{
logger.error("'paramName' not specified for ParamNameUrlHandlerMapping");
}
if (this.urlMap.isEmpty())
{
logger.info("Neither 'urlMap' nor 'mappings' set on ParamNameUrlHandlerMapping");
}
else
{
for (String url : this.urlMap.keySet())
{
Object handler = this.urlMap.get(url);
registerHandler(url, handler);
}
}
}
/**
* {@inheritDoc}
*/
@Override
protected Object getHandlerInternal(HttpServletRequest request) throws Exception
{
String lookupPath = request.getParameter(paramName);
if (lookupPath == null)
{
lookupPath = (String) request.getAttribute(paramName);
}
if (lookupPath == null)
{
log.debug("No parameter found in request for [{}]", paramName);
return null;
}
Object handler = lookupHandler(lookupPath, request);
log.debug("Returning handler [{}] for action [{}]", handler, lookupPath);
return handler;
}
}