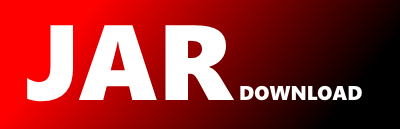
org.coode.suggestor.impl.AbstractMatcher Maven / Gradle / Ivy
package org.coode.suggestor.impl;
import java.util.HashSet;
import java.util.Set;
import javax.annotation.Nonnull;
import org.semanticweb.owlapi.model.OWLClassExpression;
import org.semanticweb.owlapi.model.OWLPropertyExpression;
import org.semanticweb.owlapi.model.OWLPropertyRange;
import org.semanticweb.owlapi.reasoner.Node;
import org.semanticweb.owlapi.reasoner.NodeSet;
abstract class AbstractMatcher
implements Matcher {
public AbstractMatcher() {}
@Override
public final boolean
isMatch(OWLClassExpression c, P p, R f, boolean direct) {
if (!direct) {
return isMatch(c, p, f);
}
if (!isMatch(c, p, f)) {
return false;
}
NodeSet directSubs = getDirectSubs(f);
for (Node node : directSubs) {
F representativeElement = node.getRepresentativeElement();
if (isMatch(c, p, representativeElement)) {
return false;
}
}
return true;
}
@Override
public final NodeSet getLeaves(OWLClassExpression c, P p, R start,
boolean direct) {
Set> nodes = new HashSet<>();
if (isMatch(c, p, start)) {
for (Node sub : getDirectSubs(start)) {
nodes.addAll(getLeaves(c, p, sub.getRepresentativeElement(),
direct).getNodes());
}
if (!direct || nodes.isEmpty() && !start.isTopEntity()) {
nodes.add(getEquivalents(start));
// non-optimal as we already had the node before recursing
}
}
return createNodeSet(nodes);
}
@Override
public final NodeSet getRoots(OWLClassExpression c, P p, R start,
boolean direct) {
Set> nodes = new HashSet<>();
for (Node sub : getDirectSubs(start)) {
if (isMatch(c, p, sub.getRepresentativeElement())) {
nodes.add(sub);
if (!direct) {
nodes.addAll(getRoots(c, p, sub.getRepresentativeElement(),
direct).getNodes());
}
}
}
return createNodeSet(nodes);
}
@Nonnull
protected abstract NodeSet getDirectSubs(@Nonnull R f);
@Nonnull
protected abstract Node getEquivalents(@Nonnull R f);
@Nonnull
protected abstract NodeSet createNodeSet(@Nonnull Set> nodes);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy