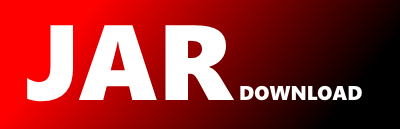
cern.colt.matrix.doc-files.function3.html Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of parallelcolt Show documentation
Show all versions of parallelcolt Show documentation
Parallel Colt is a multithreaded version of Colt - a library for high performance scientific computing in Java. It contains efficient algorithms for data analysis, linear algebra, multi-dimensional arrays, Fourier transforms, statistics and histogramming.
The newest version!
Function Objects
Example 3: Selection views based on conditions
Using condition functions (predicates), we can filter away uninteresting data
and keep only interesting data. In physics codes, this process is often called
cutting on a predicate.
Conditions on 1-d matrices (vectors)
// the naming shortcut (alias) saves some keystrokes:
cern.jet.math.Functions F = cern.jet.math.Functions.functions;
double[] v1 = {0, 1, 2, 3};
DoubleMatrix1D matrix = new DenseDoubleMatrix1D(v1);
// 0 1 2 3
// view all cells for which holds: lower <= value <= upper
final double lower = 0.2;
final double upper = 2.5
matrix.viewSelection(F.isBetween(lower, upper));
// --> 1 2
// equivalent, but less concise:
matrix.viewSelection(
new DoubleProcedure() {
public final boolean apply(double a) { return lower <= a && a <= upper; }
}
);
// --> 1 2
// view all cells with even value
matrix.viewSelection(
new DoubleProcedure() {
public final boolean apply(double a) { return a % 2 == 0; }
}
);
// --> 0 2
// sum of all cells for which holds: lower <= value <= upper
double sum = matrix.viewSelection(F.isBetween(lower, upper)).zSum();
// --> 3
// equivalent:
double sum = matrix.viewSelection(F.isBetween(lower, upper)).aggregate(F.plus,F.identity);
Conditions on 2-d matrices
// view all rows which have a value < threshold in the first column (representing "age")
final double threshold = 16;
matrix.viewSelection(
new DoubleMatrix1DProcedure() {
public final boolean apply(DoubleMatrix1D m) { return m.get(0) < threshold; }
}
);
// view all rows with RMS < threshold.
// the RMS (Root-Mean-Square) is a measure of the average "size" of the elements of a data sequence.
final double threshold = 0.5;
matrix.viewSelection(
new DoubleMatrix1DProcedure() {
public final boolean apply(DoubleMatrix1D m) { return Math.sqrt(m.aggregate(F.plus,F.square) / m.size()) < threshold; }
}
);
Conditions on 3-d matrices
// view all slices which have an aggregate sum > 1000
matrix.viewSelection(
new DoubleMatrix2DProcedure() {
public final boolean apply(DoubleMatrix2D m) { return m.zSum() > 1000; }
}
);
© 2015 - 2025 Weber Informatics LLC | Privacy Policy