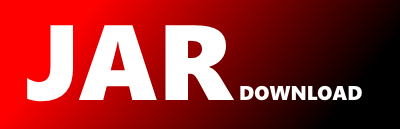
cern.jet.math.tlong.LongFunctions Maven / Gradle / Ivy
Show all versions of parallelcolt Show documentation
/*
Copyright (C) 1999 CERN - European Organization for Nuclear Research.
Permission to use, copy, modify, distribute and sell this software and its documentation for any purpose
is hereby granted without fee, provided that the above copyright notice appear in all copies and
that both that copyright notice and this permission notice appear in supporting documentation.
CERN makes no representations about the suitability of this software for any purpose.
It is provided "as is" without expressed or implied warranty.
*/
package cern.jet.math.tlong;
import cern.colt.function.tlong.LongFunction;
import cern.colt.function.tlong.LongLongFunction;
import cern.colt.function.tlong.LongLongProcedure;
import cern.colt.function.tlong.LongProcedure;
import cern.jet.math.tdouble.DoubleArithmetic;
import cern.jet.math.tdouble.DoubleFunctions;
/**
* Long Function objects to be passed to generic methods. Same as
* {@link DoubleFunctions} except operating on longs.
*
* For aliasing see {@link #longFunctions}.
*
* @author [email protected]
* @version 1.0, 09/24/99
*/
public class LongFunctions extends Object {
/**
* Little trick to allow for "aliasing", that is, renaming this class.
* Writing code like
*
* LongFunctions.chain(LongFunctions.plus,LongFunctions.mult(3),LongFunctions.chain(LongFunctions.square,LongFunctions.div(2)));
*
* is a bit awkward, to say the least. Using the aliasing you can instead
* write
*
* LongFunctions F = LongFunctions.longFunctions;
F.chain(F.plus,F.mult(3),F.chain(F.square,F.div(2)));
*
*/
public static final LongFunctions longFunctions = new LongFunctions();
/***************************************************************************
*
Unary functions
**************************************************************************/
/**
* Function that returns Math.abs(a) == (a < 0) ? -a : a.
*/
public static final LongFunction abs = new LongFunction() {
public final long apply(long a) {
return (a < 0) ? -a : a;
}
};
/**
* Function that returns a--.
*/
public static final LongFunction dec = new LongFunction() {
public final long apply(long a) {
return a--;
}
};
/**
* Function that returns (long) Arithmetic.factorial(a).
*/
public static final LongFunction factorial = new LongFunction() {
public final long apply(long a) {
return (long) DoubleArithmetic.factorial(a);
}
};
/**
* Function that returns its argument.
*/
public static final LongFunction identity = new LongFunction() {
public final long apply(long a) {
return a;
}
};
/**
* Function that returns a++.
*/
public static final LongFunction inc = new LongFunction() {
public final long apply(long a) {
return a++;
}
};
/**
* Function that returns -a.
*/
public static final LongFunction neg = new LongFunction() {
public final long apply(long a) {
return -a;
}
};
/**
* Function that returns ~a.
*/
public static final LongFunction not = new LongFunction() {
public final long apply(long a) {
return ~a;
}
};
/**
* Function that returns a < 0 ? -1 : a > 0 ? 1 : 0.
*/
public static final LongFunction sign = new LongFunction() {
public final long apply(long a) {
return a < 0 ? -1 : a > 0 ? 1 : 0;
}
};
/**
* Function that returns a * a.
*/
public static final LongFunction square = new LongFunction() {
public final long apply(long a) {
return a * a;
}
};
/***************************************************************************
* Binary functions
**************************************************************************/
/**
* Function that returns a & b.
*/
public static final LongLongFunction and = new LongLongFunction() {
public final long apply(long a, long b) {
return a & b;
}
};
/**
* Function that returns a < b ? -1 : a > b ? 1 : 0.
*/
public static final LongLongFunction compare = new LongLongFunction() {
public final long apply(long a, long b) {
return a < b ? -1 : a > b ? 1 : 0;
}
};
/**
* Function that returns a / b.
*/
public static final LongLongFunction div = new LongLongFunction() {
public final long apply(long a, long b) {
return a / b;
}
};
/**
* Function that returns -(a / b).
*/
public static final LongLongFunction divNeg = new LongLongFunction() {
public final long apply(long a, long b) {
return -(a / b);
}
};
/**
* Function that returns a == b ? 1 : 0.
*/
public static final LongLongFunction equals = new LongLongFunction() {
public final long apply(long a, long b) {
return a == b ? 1 : 0;
}
};
/**
* Function that returns a == b.
*/
public static final LongLongProcedure isEqual = new LongLongProcedure() {
public final boolean apply(long a, long b) {
return a == b;
}
};
/**
* Function that returns a < b.
*/
public static final LongLongProcedure isLess = new LongLongProcedure() {
public final boolean apply(long a, long b) {
return a < b;
}
};
/**
* Function that returns a > b.
*/
public static final LongLongProcedure isGreater = new LongLongProcedure() {
public final boolean apply(long a, long b) {
return a > b;
}
};
/**
* Function that returns Math.max(a,b).
*/
public static final LongLongFunction max = new LongLongFunction() {
public final long apply(long a, long b) {
return (a >= b) ? a : b;
}
};
/**
* Function that returns Math.min(a,b).
*/
public static final LongLongFunction min = new LongLongFunction() {
public final long apply(long a, long b) {
return (a <= b) ? a : b;
}
};
/**
* Function that returns a - b.
*/
public static final LongLongFunction minus = new LongLongFunction() {
public final long apply(long a, long b) {
return a - b;
}
};
/**
* Function that returns a % b.
*/
public static final LongLongFunction mod = new LongLongFunction() {
public final long apply(long a, long b) {
return a % b;
}
};
/**
* Function that returns a * b.
*/
public static final LongLongFunction mult = new LongLongFunction() {
public final long apply(long a, long b) {
return a * b;
}
};
/**
* Function that returns -(a * b).
*/
public static final LongLongFunction multNeg = new LongLongFunction() {
public final long apply(long a, long b) {
return -(a * b);
}
};
/**
* Function that returns a * b^2.
*/
public static final LongLongFunction multSquare = new LongLongFunction() {
public final long apply(long a, long b) {
return a * b * b;
}
};
/**
* Function that returns a | b.
*/
public static final LongLongFunction or = new LongLongFunction() {
public final long apply(long a, long b) {
return a | b;
}
};
/**
* Function that returns a + b.
*/
public static final LongLongFunction plus = new LongLongFunction() {
public final long apply(long a, long b) {
return a + b;
}
};
/**
* Function that returns Math.abs(a) + Math.abs(b).
*/
public static final LongLongFunction plusAbs = new LongLongFunction() {
public final long apply(long a, long b) {
return Math.abs(a) + Math.abs(b);
}
};
/**
* Function that returns (long) Math.pow(a,b).
*/
public static final LongLongFunction pow = new LongLongFunction() {
public final long apply(long a, long b) {
return (long) Math.pow(a, b);
}
};
/**
* Function that returns a << b.
*/
public static final LongLongFunction shiftLeft = new LongLongFunction() {
public final long apply(long a, long b) {
return a << b;
}
};
/**
* Function that returns a >> b.
*/
public static final LongLongFunction shiftRightSigned = new LongLongFunction() {
public final long apply(long a, long b) {
return a >> b;
}
};
/**
* Function that returns a >>> b.
*/
public static final LongLongFunction shiftRightUnsigned = new LongLongFunction() {
public final long apply(long a, long b) {
return a >>> b;
}
};
/**
* Function that returns a ^ b.
*/
public static final LongLongFunction xor = new LongLongFunction() {
public final long apply(long a, long b) {
return a ^ b;
}
};
/**
* Makes this class non instantiable, but still let's others inherit from
* it.
*/
protected LongFunctions() {
}
/**
* Constructs a function that returns a & b. a is a
* variable, b is fixed.
*/
public static LongFunction and(final long b) {
return new LongFunction() {
public final long apply(long a) {
return a & b;
}
};
}
/**
* Constructs a function that returns (from<=a && a<=to) ? 1 : 0.
* a is a variable, from and to are fixed.
*/
public static LongFunction between(final long from, final long to) {
return new LongFunction() {
public final long apply(long a) {
return (from <= a && a <= to) ? 1 : 0;
}
};
}
/**
* Constructs a unary function from a binary function with the first operand
* (argument) fixed to the given constant c. The second operand is
* variable (free).
*
* @param function
* a binary function taking operands in the form
* function.apply(c,var).
* @return the unary function function(c,var).
*/
public static LongFunction bindArg1(final LongLongFunction function, final long c) {
return new LongFunction() {
public final long apply(long var) {
return function.apply(c, var);
}
};
}
/**
* Constructs a unary function from a binary function with the second
* operand (argument) fixed to the given constant c. The first
* operand is variable (free).
*
* @param function
* a binary function taking operands in the form
* function.apply(var,c).
* @return the unary function function(var,c).
*/
public static LongFunction bindArg2(final LongLongFunction function, final long c) {
return new LongFunction() {
public final long apply(long var) {
return function.apply(var, c);
}
};
}
/**
* Constructs the function g( h(a) ).
*
* @param g
* a unary function.
* @param h
* a unary function.
* @return the unary function g( h(a) ).
*/
public static LongFunction chain(final LongFunction g, final LongFunction h) {
return new LongFunction() {
public final long apply(long a) {
return g.apply(h.apply(a));
}
};
}
/**
* Constructs the function g( h(a,b) ).
*
* @param g
* a unary function.
* @param h
* a binary function.
* @return the unary function g( h(a,b) ).
*/
public static LongLongFunction chain(final LongFunction g, final LongLongFunction h) {
return new LongLongFunction() {
public final long apply(long a, long b) {
return g.apply(h.apply(a, b));
}
};
}
/**
* Constructs the function f( g(a), h(b) ).
*
* @param f
* a binary function.
* @param g
* a unary function.
* @param h
* a unary function.
* @return the binary function f( g(a), h(b) ).
*/
public static LongLongFunction chain(final LongLongFunction f, final LongFunction g, final LongFunction h) {
return new LongLongFunction() {
public final long apply(long a, long b) {
return f.apply(g.apply(a), h.apply(b));
}
};
}
/**
* Constructs a function that returns a < b ? -1 : a > b ? 1 : 0.
* a is a variable, b is fixed.
*/
public static LongFunction compare(final long b) {
return new LongFunction() {
public final long apply(long a) {
return a < b ? -1 : a > b ? 1 : 0;
}
};
}
/**
* Constructs a function that returns the constant c.
*/
public static LongFunction constant(final long c) {
return new LongFunction() {
public final long apply(long a) {
return c;
}
};
}
/**
* Constructs a function that returns a / b. a is a
* variable, b is fixed.
*/
public static LongFunction div(final long b) {
return new LongFunction() {
public final long apply(long a) {
return a / b;
}
};
}
/**
* Constructs a function that returns a == b ? 1 : 0. a is
* a variable, b is fixed.
*/
public static LongFunction equals(final long b) {
return new LongFunction() {
public final long apply(long a) {
return a == b ? 1 : 0;
}
};
}
/**
* Constructs a function that returns from<=a && a<=to. a
* is a variable, from and to are fixed.
*/
public static LongProcedure isBetween(final long from, final long to) {
return new LongProcedure() {
public final boolean apply(long a) {
return from <= a && a <= to;
}
};
}
/**
* Constructs a function that returns a == b. a is a
* variable, b is fixed.
*/
public static LongProcedure isEqual(final long b) {
return new LongProcedure() {
public final boolean apply(long a) {
return a == b;
}
};
}
/**
* Constructs a function that returns a > b. a is a
* variable, b is fixed.
*/
public static LongProcedure isGreater(final long b) {
return new LongProcedure() {
public final boolean apply(long a) {
return a > b;
}
};
}
/**
* Constructs a function that returns a < b. a is a
* variable, b is fixed.
*/
public static LongProcedure isLess(final long b) {
return new LongProcedure() {
public final boolean apply(long a) {
return a < b;
}
};
}
/**
* Constructs a function that returns Math.max(a,b). a is
* a variable, b is fixed.
*/
public static LongFunction max(final long b) {
return new LongFunction() {
public final long apply(long a) {
return (a >= b) ? a : b;
}
};
}
/**
* Constructs a function that returns Math.min(a,b). a is
* a variable, b is fixed.
*/
public static LongFunction min(final long b) {
return new LongFunction() {
public final long apply(long a) {
return (a <= b) ? a : b;
}
};
}
/**
* Constructs a function that returns a - b. a is a
* variable, b is fixed.
*/
public static LongFunction minus(final long b) {
return new LongFunction() {
public final long apply(long a) {
return a - b;
}
};
}
/**
* Constructs a function that returns a - b*constant. a
* and b are variables, constant is fixed.
*/
public static LongLongFunction minusMult(final long constant) {
return plusMultSecond(-constant);
}
/**
* Constructs a function that returns a % b. a is a
* variable, b is fixed.
*/
public static LongFunction mod(final long b) {
return new LongFunction() {
public final long apply(long a) {
return a % b;
}
};
}
/**
* Constructs a function that returns a * b. a is a
* variable, b is fixed.
*/
public static LongFunction mult(final long b) {
return new LongFunction() {
public final long apply(long a) {
return a * b;
}
};
}
/**
* Constructs a function that returns a | b. a is a
* variable, b is fixed.
*/
public static LongFunction or(final long b) {
return new LongFunction() {
public final long apply(long a) {
return a | b;
}
};
}
/**
* Constructs a function that returns a + b. a is a
* variable, b is fixed.
*/
public static LongFunction plus(final long b) {
return new LongFunction() {
public final long apply(long a) {
return a + b;
}
};
}
/**
* Constructs a function that returns b*constant.
*/
public static LongLongFunction multSecond(final long constant) {
return new LongLongFunction() {
public final long apply(long a, long b) {
return b * constant;
}
};
}
/**
* Constructs a function that returns (long) Math.pow(a,b).
* a is a variable, b is fixed.
*/
public static LongFunction pow(final long b) {
return new LongFunction() {
public final long apply(long a) {
return (long) Math.pow(a, b);
}
};
}
/**
* Constructs a function that returns a + b*constant. a
* and b are variables, constant is fixed.
*/
public static LongLongFunction plusMultSecond(final long constant) {
return new LongPlusMultSecond(constant);
}
/**
* Constructs a function that returns a * constant + b. a
* and b are variables, constant is fixed.
*/
public static LongLongFunction plusMultFirst(final long constant) {
return new LongPlusMultFirst(constant);
}
/**
* Constructs a function that returns a 32 bit uniformly distributed random
* number in the closed longerval [Long.MIN_VALUE,Long.MAX_VALUE]
* (including Long.MIN_VALUE and Long.MAX_VALUE).
* Currently the engine is
* {@link cern.jet.random.tdouble.engine.DoubleMersenneTwister} and is
* seeded with the current time.
*
* Note that any random engine derived from
* {@link cern.jet.random.tdouble.engine.DoubleRandomEngine} and any random
* distribution derived from
* {@link cern.jet.random.tdouble.AbstractDoubleDistribution} are function
* objects, because they implement the proper longerfaces. Thus, if you are
* not happy with the default, just pass your favourite random generator to
* function evaluating methods.
*/
public static LongFunction random() {
return new cern.jet.random.tdouble.engine.DoubleMersenneTwister(new java.util.Date());
}
/**
* Constructs a function that returns a << b. a is a
* variable, b is fixed.
*/
public static LongFunction shiftLeft(final long b) {
return new LongFunction() {
public final long apply(long a) {
return a << b;
}
};
}
/**
* Constructs a function that returns a >> b. a is a
* variable, b is fixed.
*/
public static LongFunction shiftRightSigned(final long b) {
return new LongFunction() {
public final long apply(long a) {
return a >> b;
}
};
}
/**
* Constructs a function that returns a >>> b. a is a
* variable, b is fixed.
*/
public static LongFunction shiftRightUnsigned(final long b) {
return new LongFunction() {
public final long apply(long a) {
return a >>> b;
}
};
}
/**
* Constructs a function that returns function.apply(b,a), i.e.
* applies the function with the first operand as second operand and the
* second operand as first operand.
*
* @param function
* a function taking operands in the form
* function.apply(a,b).
* @return the binary function function(b,a).
*/
public static LongLongFunction swapArgs(final LongLongFunction function) {
return new LongLongFunction() {
public final long apply(long a, long b) {
return function.apply(b, a);
}
};
}
/**
* Constructs a function that returns a | b. a is a
* variable, b is fixed.
*/
public static LongFunction xor(final long b) {
return new LongFunction() {
public final long apply(long a) {
return a ^ b;
}
};
}
}