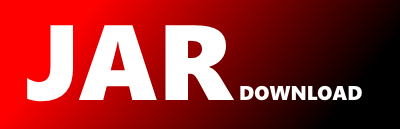
hep.aida.tfloat.ref.FloatConverter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of parallelcolt Show documentation
Show all versions of parallelcolt Show documentation
Parallel Colt is a multithreaded version of Colt - a library for high performance scientific computing in Java. It contains efficient algorithms for data analysis, linear algebra, multi-dimensional arrays, Fourier transforms, statistics and histogramming.
The newest version!
package hep.aida.tfloat.ref;
import hep.aida.tfloat.FloatIAxis;
import hep.aida.tfloat.FloatIHistogram1D;
import hep.aida.tfloat.FloatIHistogram2D;
import hep.aida.tfloat.FloatIHistogram3D;
/**
* Histogram conversions, for example to String and XML format; This class
* requires the Colt distribution, whereas the rest of the package is entirelly
* stand-alone.
*/
public class FloatConverter {
/**
* Creates a new histogram converter.
*/
public FloatConverter() {
}
/**
* Returns all edges of the given axis.
*/
public float[] edges(FloatIAxis axis) {
int b = axis.bins();
float[] bounds = new float[b + 1];
for (int i = 0; i < b; i++)
bounds[i] = axis.binLowerEdge(i);
bounds[b] = axis.upperEdge();
return bounds;
}
String form(cern.colt.matrix.Former formatter, float value) {
return formatter.form(value);
}
/**
* Returns an array[h.xAxis().bins()]; ignoring extra bins.
*/
protected float[] toArrayErrors(FloatIHistogram1D h) {
int xBins = h.xAxis().bins();
float[] array = new float[xBins];
for (int j = xBins; --j >= 0;) {
array[j] = h.binError(j);
}
return array;
}
/**
* Returns an array[h.xAxis().bins()][h.yAxis().bins()]; ignoring extra
* bins.
*/
protected float[][] toArrayErrors(FloatIHistogram2D h) {
int xBins = h.xAxis().bins();
int yBins = h.yAxis().bins();
float[][] array = new float[xBins][yBins];
for (int i = yBins; --i >= 0;) {
for (int j = xBins; --j >= 0;) {
array[j][i] = h.binError(j, i);
}
}
return array;
}
/**
* Returns an array[h.xAxis().bins()]; ignoring extra bins.
*/
protected float[] toArrayHeights(FloatIHistogram1D h) {
int xBins = h.xAxis().bins();
float[] array = new float[xBins];
for (int j = xBins; --j >= 0;) {
array[j] = h.binHeight(j);
}
return array;
}
/**
* Returns an array[h.xAxis().bins()][h.yAxis().bins()]; ignoring extra
* bins.
*/
protected float[][] toArrayHeights(FloatIHistogram2D h) {
int xBins = h.xAxis().bins();
int yBins = h.yAxis().bins();
float[][] array = new float[xBins][yBins];
for (int i = yBins; --i >= 0;) {
for (int j = xBins; --j >= 0;) {
array[j][i] = h.binHeight(j, i);
}
}
return array;
}
/**
* Returns an array[h.xAxis().bins()][h.yAxis().bins()][h.zAxis().bins()];
* ignoring extra bins.
*/
protected float[][][] toArrayHeights(FloatIHistogram3D h) {
int xBins = h.xAxis().bins();
int yBins = h.yAxis().bins();
int zBins = h.zAxis().bins();
float[][][] array = new float[xBins][yBins][zBins];
for (int j = xBins; --j >= 0;) {
for (int i = yBins; --i >= 0;) {
for (int k = zBins; --k >= 0;) {
array[j][i][k] = h.binHeight(j, i, k);
}
}
}
return array;
}
/**
* Returns a string representation of the specified array. The string
* representation consists of a list of the arrays's elements, enclosed in
* square brackets ("[]"). Adjacent elements are separated by the
* characters ", " (comma and space).
*
* @return a string representation of the specified array.
*/
protected static String toString(float[] array) {
StringBuffer buf = new StringBuffer();
buf.append("[");
int maxIndex = array.length - 1;
for (int i = 0; i <= maxIndex; i++) {
buf.append(array[i]);
if (i < maxIndex)
buf.append(", ");
}
buf.append("]");
return buf.toString();
}
/**
* Returns a string representation of the given argument.
*/
public String toString(FloatIAxis axis) {
StringBuffer buf = new StringBuffer();
buf.append("Range: [" + axis.lowerEdge() + "," + axis.upperEdge() + ")");
buf.append(", Bins: " + axis.bins());
buf.append(", Bin edges: " + toString(edges(axis)) + "\n");
return buf.toString();
}
/**
* Returns a string representation of the given argument.
*/
public String toString(FloatIHistogram1D h) {
String columnAxisName = null; // "X";
String rowAxisName = null;
hep.aida.tfloat.bin.FloatBinFunction1D[] aggr = null; // {hep.aida.bin.BinFunctions1D.sum};
String format = "%G";
// String format = "%1.2G";
cern.colt.matrix.Former f = new cern.colt.matrix.FormerFactory().create(format);
String sep = System.getProperty("line.separator");
int[] minMaxBins = h.minMaxBins();
String title = h.title() + ":" + sep + " Entries=" + form(f, h.entries()) + ", ExtraEntries="
+ form(f, h.extraEntries()) + sep + " Mean=" + form(f, h.mean()) + ", Rms=" + form(f, h.rms()) + sep
+ " MinBinHeight=" + form(f, h.binHeight(minMaxBins[0])) + ", MaxBinHeight="
+ form(f, h.binHeight(minMaxBins[1])) + sep + " Axis: " + "Bins=" + form(f, h.xAxis().bins())
+ ", Min=" + form(f, h.xAxis().lowerEdge()) + ", Max=" + form(f, h.xAxis().upperEdge());
String[] xEdges = new String[h.xAxis().bins()];
for (int i = 0; i < h.xAxis().bins(); i++)
xEdges[i] = form(f, h.xAxis().binLowerEdge(i));
String[] yEdges = null;
cern.colt.matrix.tfloat.FloatMatrix2D heights = new cern.colt.matrix.tfloat.impl.DenseFloatMatrix2D(1, h
.xAxis().bins());
heights.viewRow(0).assign(toArrayHeights(h));
// cern.colt.matrix.FloatMatrix2D errors = new
// cern.colt.matrix.impl.DenseFloatMatrix2D(1,h.xAxis().bins());
// errors.viewRow(0).assign(toArrayErrors(h));
return title
+ sep
+ "Heights:"
+ sep
+ new cern.colt.matrix.tfloat.algo.FloatFormatter().toTitleString(heights, yEdges, xEdges, rowAxisName,
columnAxisName, null, aggr);
/*
* + sep + "Errors:" + sep + new
* cern.colt.matrix.floatalgo.Formatter().toTitleString(
* errors,yEdges,xEdges,rowAxisName,columnAxisName,null,aggr);
*/
}
/**
* Returns a string representation of the given argument.
*/
public String toString(FloatIHistogram2D h) {
String columnAxisName = "X";
String rowAxisName = "Y";
hep.aida.tfloat.bin.FloatBinFunction1D[] aggr = { hep.aida.tfloat.bin.FloatBinFunctions1D.sum };
String format = "%G";
// String format = "%1.2G";
cern.colt.matrix.Former f = new cern.colt.matrix.FormerFactory().create(format);
String sep = System.getProperty("line.separator");
int[] minMaxBins = h.minMaxBins();
String title = h.title() + ":" + sep + " Entries=" + form(f, h.entries()) + ", ExtraEntries="
+ form(f, h.extraEntries()) + sep + " MeanX=" + form(f, h.meanX()) + ", RmsX=" + form(f, h.rmsX())
+ sep + " MeanY=" + form(f, h.meanY()) + ", RmsY=" + form(f, h.rmsX()) + sep + " MinBinHeight="
+ form(f, h.binHeight(minMaxBins[0], minMaxBins[1])) + ", MaxBinHeight="
+ form(f, h.binHeight(minMaxBins[2], minMaxBins[3])) + sep +
" xAxis: " + "Bins=" + form(f, h.xAxis().bins()) + ", Min=" + form(f, h.xAxis().lowerEdge())
+ ", Max=" + form(f, h.xAxis().upperEdge()) + sep +
" yAxis: " + "Bins=" + form(f, h.yAxis().bins()) + ", Min=" + form(f, h.yAxis().lowerEdge())
+ ", Max=" + form(f, h.yAxis().upperEdge());
String[] xEdges = new String[h.xAxis().bins()];
for (int i = 0; i < h.xAxis().bins(); i++)
xEdges[i] = form(f, h.xAxis().binLowerEdge(i));
String[] yEdges = new String[h.yAxis().bins()];
for (int i = 0; i < h.yAxis().bins(); i++)
yEdges[i] = form(f, h.yAxis().binLowerEdge(i));
new cern.colt.list.tobject.ObjectArrayList(yEdges).reverse(); // keep coord.
// system
cern.colt.matrix.tfloat.FloatMatrix2D heights = new cern.colt.matrix.tfloat.impl.DenseFloatMatrix2D(
toArrayHeights(h));
heights = heights.viewDice().viewRowFlip(); // keep the histo coord.
// system
// heights = heights.viewPart(1,1,heights.rows()-2,heights.columns()-2);
// // ignore under&overflows
// cern.colt.matrix.FloatMatrix2D errors = new
// cern.colt.matrix.impl.DenseFloatMatrix2D(toArrayErrors(h));
// errors = errors.viewDice().viewRowFlip(); // keep the histo coord
// system
// //errors = errors.viewPart(1,1,errors.rows()-2,errors.columns()-2);
// // ignore under&overflows
return title
+ sep
+ "Heights:"
+ sep
+ new cern.colt.matrix.tfloat.algo.FloatFormatter().toTitleString(heights, yEdges, xEdges, rowAxisName,
columnAxisName, null, aggr);
/*
* + sep + "Errors:" + sep + new
* cern.colt.matrix.floatalgo.Formatter().toTitleString(
* errors,yEdges,xEdges,rowAxisName,columnAxisName,null,aggr);
*/
}
/**
* Returns a string representation of the given argument.
*/
public String toString(FloatIHistogram3D h) {
String columnAxisName = "X";
String rowAxisName = "Y";
String sliceAxisName = "Z";
hep.aida.tfloat.bin.FloatBinFunction1D[] aggr = { hep.aida.tfloat.bin.FloatBinFunctions1D.sum };
String format = "%G";
// String format = "%1.2G";
cern.colt.matrix.Former f = new cern.colt.matrix.FormerFactory().create(format);
String sep = System.getProperty("line.separator");
int[] minMaxBins = h.minMaxBins();
String title = h.title() + ":" + sep + " Entries=" + form(f, h.entries()) + ", ExtraEntries="
+ form(f, h.extraEntries()) + sep + " MeanX=" + form(f, h.meanX()) + ", RmsX=" + form(f, h.rmsX())
+ sep + " MeanY=" + form(f, h.meanY()) + ", RmsY=" + form(f, h.rmsX()) + sep + " MeanZ="
+ form(f, h.meanZ()) + ", RmsZ=" + form(f, h.rmsZ()) + sep + " MinBinHeight="
+ form(f, h.binHeight(minMaxBins[0], minMaxBins[1], minMaxBins[2])) + ", MaxBinHeight="
+ form(f, h.binHeight(minMaxBins[3], minMaxBins[4], minMaxBins[5])) + sep +
" xAxis: " + "Bins=" + form(f, h.xAxis().bins()) + ", Min=" + form(f, h.xAxis().lowerEdge())
+ ", Max=" + form(f, h.xAxis().upperEdge()) + sep +
" yAxis: " + "Bins=" + form(f, h.yAxis().bins()) + ", Min=" + form(f, h.yAxis().lowerEdge())
+ ", Max=" + form(f, h.yAxis().upperEdge()) + sep +
" zAxis: " + "Bins=" + form(f, h.zAxis().bins()) + ", Min=" + form(f, h.zAxis().lowerEdge())
+ ", Max=" + form(f, h.zAxis().upperEdge());
String[] xEdges = new String[h.xAxis().bins()];
for (int i = 0; i < h.xAxis().bins(); i++)
xEdges[i] = form(f, h.xAxis().binLowerEdge(i));
String[] yEdges = new String[h.yAxis().bins()];
for (int i = 0; i < h.yAxis().bins(); i++)
yEdges[i] = form(f, h.yAxis().binLowerEdge(i));
new cern.colt.list.tobject.ObjectArrayList(yEdges).reverse(); // keep coord.
// system
String[] zEdges = new String[h.zAxis().bins()];
for (int i = 0; i < h.zAxis().bins(); i++)
zEdges[i] = form(f, h.zAxis().binLowerEdge(i));
new cern.colt.list.tobject.ObjectArrayList(zEdges).reverse(); // keep coord.
// system
cern.colt.matrix.tfloat.FloatMatrix3D heights = new cern.colt.matrix.tfloat.impl.DenseFloatMatrix3D(
toArrayHeights(h));
heights = heights.viewDice(2, 1, 0).viewSliceFlip().viewRowFlip(); // keep
// the
// histo
// coord.
// system
// heights = heights.viewPart(1,1,heights.rows()-2,heights.columns()-2);
// // ignore under&overflows
// cern.colt.matrix.FloatMatrix2D errors = new
// cern.colt.matrix.impl.DenseFloatMatrix2D(toArrayErrors(h));
// errors = errors.viewDice().viewRowFlip(); // keep the histo coord
// system
// //errors = errors.viewPart(1,1,errors.rows()-2,errors.columns()-2);
// // ignore under&overflows
return title
+ sep
+ "Heights:"
+ sep
+ new cern.colt.matrix.tfloat.algo.FloatFormatter().toTitleString(heights, zEdges, yEdges, xEdges,
sliceAxisName, rowAxisName, columnAxisName, "", aggr);
/*
* + sep + "Errors:" + sep + new
* cern.colt.matrix.floatalgo.Formatter().toTitleString(
* errors,yEdges,xEdges,rowAxisName,columnAxisName,null,aggr);
*/
}
/**
* Returns a XML representation of the given argument.
*/
public String toXML(FloatIHistogram1D h) {
StringBuffer buf = new StringBuffer();
String sep = System.getProperty("line.separator");
buf.append("");
buf.append(sep);
buf.append("");
buf.append(sep);
buf.append("");
buf.append(sep);
buf.append("");
buf.append(sep);
buf.append("");
buf.append(sep);
buf.append("");
buf.append(sep);
buf.append("");
buf.append(sep);
for (int i = 0; i < h.xAxis().bins(); i++) {
buf.append(h.binEntries(i) + "," + h.binError(i));
buf.append(sep);
}
buf.append(" ");
buf.append(sep);
buf.append(" ");
buf.append(sep);
buf.append("");
buf.append(sep);
buf.append(" ");
buf.append(sep);
buf.append(" ");
buf.append(sep);
buf.append(" ");
buf.append(sep);
if (!Float.isNaN(h.mean())) {
buf.append(" ");
buf.append(sep);
}
if (!Float.isNaN(h.rms())) {
buf.append(" ");
buf.append(sep);
}
buf.append(" ");
buf.append(sep);
buf.append(" ");
buf.append(sep);
buf.append(" ");
buf.append(sep);
buf.append(" ");
buf.append(sep);
buf.append(" ");
buf.append(sep);
return buf.toString();
}
/**
* Returns a XML representation of the given argument.
*/
public String toXML(FloatIHistogram2D h) {
StringBuffer out = new StringBuffer();
String sep = System.getProperty("line.separator");
out.append("");
out.append(sep);
out.append("");
out.append(sep);
out.append("");
out.append(sep);
out.append("");
out.append(sep);
out.append("");
out.append(sep);
out.append("");
out.append(sep);
out.append("");
out.append(sep);
for (int i = 0; i < h.xAxis().bins(); i++)
for (int j = 0; j < h.yAxis().bins(); j++) {
out.append(h.binEntries(i, j) + "," + h.binError(i, j));
out.append(sep);
}
out.append(" ");
out.append(sep);
out.append(" ");
out.append(sep);
out.append(" ");
out.append(sep);
// out.append(""); out.append(sep);
// out.append(""); out.append(sep);
// out.append("");
// out.append(sep);
// out.append("");
// out.append(sep);
// out.append("");
// out.append(sep);
// out.append("");
// out.append(sep);
// out.append(" "); out.append(sep);
out.append(" ");
out.append(sep);
out.append(" ");
out.append(sep);
out.append(" ");
out.append(sep);
out.append(" ");
out.append(sep);
return out.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy