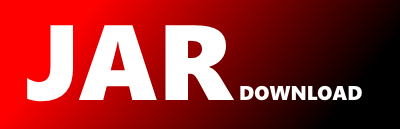
hep.aida.tfloat.ref.FloatVariableAxis Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of parallelcolt Show documentation
Show all versions of parallelcolt Show documentation
Parallel Colt is a multithreaded version of Colt - a library for high performance scientific computing in Java. It contains efficient algorithms for data analysis, linear algebra, multi-dimensional arrays, Fourier transforms, statistics and histogramming.
The newest version!
package hep.aida.tfloat.ref;
import hep.aida.tfloat.FloatIAxis;
import hep.aida.tfloat.FloatIHistogram;
/**
* Variable-width axis; A reference implementation of hep.aida.IAxis.
*
* @author Wolfgang Hoschek, Tony Johnson, and others.
* @version 1.0, 23/03/2000
*/
public class FloatVariableAxis implements FloatIAxis {
/**
*
*/
private static final long serialVersionUID = 1L;
protected float min;
protected int bins;
protected float[] edges;
/**
* Constructs and returns an axis with the given bin edges. Example:
* edges = (0.2, 1.0, 5.0) yields an axis with 2 in-range bins
* [0.2,1.0), [1.0,5.0) and 2 extra bins
* [-inf,0.2), [5.0,inf].
*
* @param edges
* the bin boundaries the partition shall have; must be sorted
* ascending and must not contain multiple identical elements.
* @throws IllegalArgumentException
* if edges.length < 1.
*/
public FloatVariableAxis(float[] edges) {
if (edges.length < 1)
throw new IllegalArgumentException();
// check if really sorted and has no multiple identical elements
for (int i = 0; i < edges.length - 1; i++) {
if (edges[i + 1] <= edges[i]) {
throw new IllegalArgumentException(
"edges must be sorted ascending and must not contain multiple identical values");
}
}
this.min = edges[0];
this.bins = edges.length - 1;
this.edges = edges.clone();
}
public float binCentre(int index) {
return (binLowerEdge(index) + binUpperEdge(index)) / 2;
}
public float binLowerEdge(int index) {
if (index == FloatIHistogram.UNDERFLOW)
return Float.NEGATIVE_INFINITY;
if (index == FloatIHistogram.OVERFLOW)
return upperEdge();
return edges[index];
}
public int bins() {
return bins;
}
public float binUpperEdge(int index) {
if (index == FloatIHistogram.UNDERFLOW)
return lowerEdge();
if (index == FloatIHistogram.OVERFLOW)
return Float.POSITIVE_INFINITY;
return edges[index + 1];
}
public float binWidth(int index) {
return binUpperEdge(index) - binLowerEdge(index);
}
public int coordToIndex(float coord) {
if (coord < min)
return FloatIHistogram.UNDERFLOW;
int index = java.util.Arrays.binarySearch(this.edges, coord);
// int index = new FloatArrayList(this.edges).binarySearch(coord); //
// just for debugging
if (index < 0)
index = -index - 1 - 1; // not found
// else index++; // found
if (index >= bins)
return FloatIHistogram.OVERFLOW;
return index;
}
public float lowerEdge() {
return min;
}
/**
* Returns a string representation of the specified array. The string
* representation consists of a list of the arrays's elements, enclosed in
* square brackets ("[]"). Adjacent elements are separated by the
* characters ", " (comma and space).
*
* @return a string representation of the specified array.
*/
protected static String toString(float[] array) {
StringBuffer buf = new StringBuffer();
buf.append("[");
int maxIndex = array.length - 1;
for (int i = 0; i <= maxIndex; i++) {
buf.append(array[i]);
if (i < maxIndex)
buf.append(", ");
}
buf.append("]");
return buf.toString();
}
public float upperEdge() {
return edges[edges.length - 1];
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy